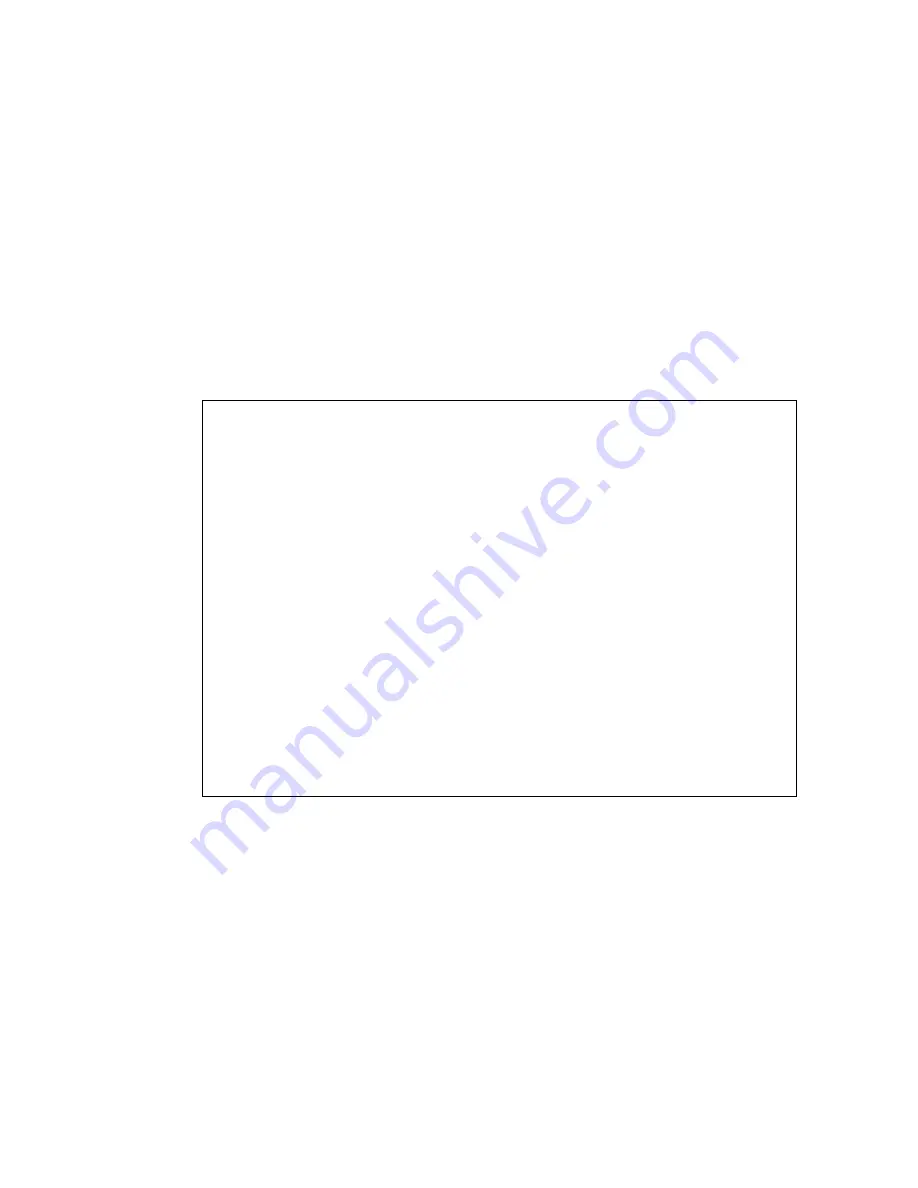
2:528
Volume 2, Part 2: MP Coherence and Synchronization
2.4.2
Simple Barrier Synchronization
A barrier is a common synchronization primitive used to hold a set of processes at a
particular point in the program (the barrier) until all processors reach the location.
Once all processes arrive at the barrier, they may all continue to execute.
shows a sense-reversing barrier synchronization based on the
fetchadd
instruction
from Hennessy and Patterson [HP96].
This type of barrier prevents a process that races ahead to the next instance of the
barrier from trapping other (slow) processors that are in the process of leaving the
barrier.
The barrier code begins by atomically updating the number of processors that are
waiting at the barrier,
count
, using a
fetchadd
instruction. For the last processor that
reaches the barrier, the
fetchadd
instruction returns the same value as the
total
shared variable, which is one less than the number of processors that wait at the
barrier. Other processors each get a unique value on the interval [0,
total
) based on
the order in which they arrive at the barrier.
All processors except the last processor wait in the
wait_on_others
loop for the signal
that all have arrived at the barrier. The last processor to arrive at the barrier provides
this signal.
The signal to leave the barrier is deduced from the value of the
release
shared variable
and the
local_sense
local variable. Upon entering the barrier, each processor
complements the value in its private
local_sense
variable. Once in the barrier, all
processors always have the same value in their
local_sense
variables. This variable
Figure 2-5.
Sense-reversing Barrier Synchronization Code
// The total shared variable is one less than the number of processors
// that wait at the barrier.
// The release shared variable indicates if the processor must wait at
// the barrier (initially, this variable is 0).
// local_sense is a per-processor local variable that indicates the
// "sense" of the barrier (initially, this variable is 0).
sr_barrier:
fetchadd8.acq
r1 = [count], 1
// update counter
ld8
r2 = [total]
// get number of procs - 1
ld8
r3 = [local_sense] ;;
// get local “sense” variable
xor
r3 = 1, r3
// local_sense =! local_sense
cmp.eq
p1, p2 = r1, r2;;
// p1 => last proc to arrive
st8
[local_sense] = r3
// save new value of local_sense
(p1)
st8
[count] = r0
// last resets count to 0
(p1)
st8.rel
[release] = r3 ;;
// last allows other to leave
wait_on_others:
(p2)
ld8
r1 = [release] ;;
// p2 => more procs to come
(p2)
cmp.ne.and
p0, p2 = r1, r3
// have all arrived yet?
(p2)
br.cond.sptk
wait_on_others ;;
// nope, continue waiting
// This mf prevents memory operations that follow the barrier code
// from moving ahead of memory operations that precede the barrier
// code
mf ;;
Summary of Contents for ITANIUM ARCHITECTURE - SOFTWARE DEVELOPERS VOLUME 3 REV 2.3
Page 1: ......
Page 11: ...x Intel Itanium Architecture Software Developer s Manual Rev 2 3 ...
Page 13: ...1 2 Intel Itanium Architecture Software Developer s Manual Rev 2 3 ...
Page 33: ...1 22 Volume 1 Part 1 Introduction to the Intel Itanium Architecture ...
Page 57: ...1 46 Volume 1 Part 1 Execution Environment ...
Page 147: ...1 136 Intel Itanium Architecture Software Developer s Manual Rev 2 3 ...
Page 149: ...1 138 Volume 1 Part 2 About the Optimization Guide ...
Page 191: ...1 180 Volume 1 Part 2 Predication Control Flow and Instruction Stream ...
Page 230: ......
Page 248: ...236 Intel Itanium Architecture Software Developer s Manual Rev 2 3 ...
Page 250: ...2 2 Intel Itanium Architecture Software Developer s Manual Rev 2 3 ...
Page 264: ...2 16 Volume 2 Part 1 Intel Itanium System Environment ...
Page 380: ...2 132 Volume 2 Part 1 Interruptions ...
Page 398: ...2 150 Volume 2 Part 1 Register Stack Engine ...
Page 486: ...2 238 Volume 2 Part 1 IA 32 Interruption Vector Descriptions ...
Page 750: ...2 502 Intel Itanium Architecture Software Developer s Manual Rev 2 3 ...
Page 754: ...2 506 Volume 2 Part 2 About the System Programmer s Guide ...
Page 796: ...2 548 Volume 2 Part 2 Interruptions and Serialization ...
Page 808: ...2 560 Volume 2 Part 2 Context Management ...
Page 842: ...2 594 Volume 2 Part 2 Floating point System Software ...
Page 850: ...2 602 Volume 2 Part 2 IA 32 Application Support ...
Page 862: ...2 614 Volume 2 Part 2 External Interrupt Architecture ...
Page 870: ...2 622 Volume 2 Part 2 Performance Monitoring Support ...
Page 891: ......
Page 1099: ...3 200 Volume 3 Instruction Reference padd Interruptions Illegal Operation fault ...
Page 1295: ...3 396 Volume 3 Resource and Dependency Semantics ...
Page 1296: ......
Page 1302: ...402 Intel Itanium Architecture Software Developer s Manual Rev 2 3 ...
Page 1494: ...4 192 Volume 4 Base IA 32 Instruction Reference FWAIT Wait See entry for WAIT ...
Page 1647: ...Volume 4 Base IA 32 Instruction Reference 4 345 ROL ROR Rotate See entry for RCL RCR ROL ROR ...
Page 1884: ...4 582 Volume 4 IA 32 SSE Instruction Reference ...
Page 1885: ...Index Intel Itanium Architecture Software Developer s Manual Rev 2 3 Index ...
Page 1886: ...Index Intel Itanium Architecture Software Developer s Manual Rev 2 3 ...
Page 1898: ...INDEX Index 12 Index for Volumes 1 2 3 and 4 ...