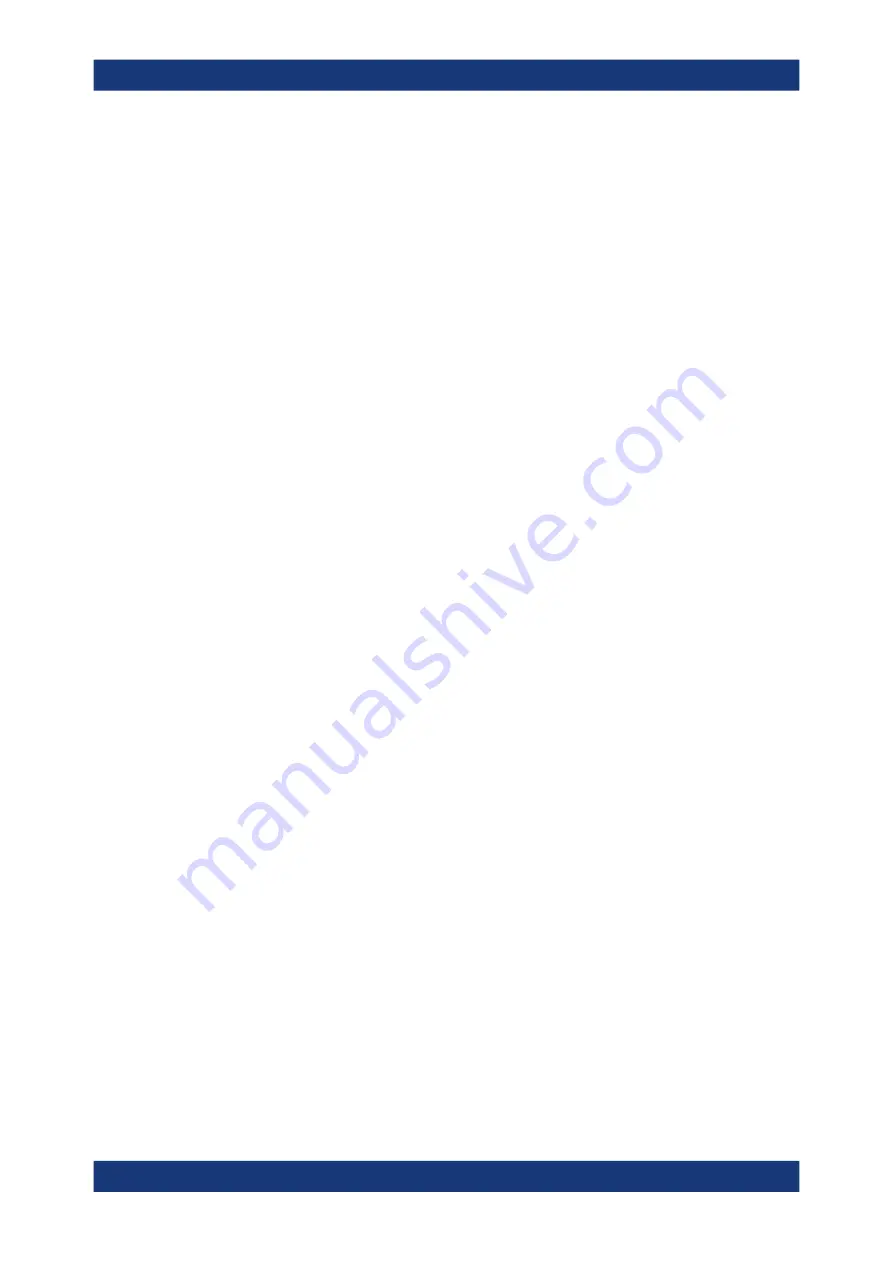
Remote control commands
R&S
®
RTO6
1272
User Manual 1801.6687.02 ─ 05
EXPort:WAVeform:NAME "C:\Users\Public\Documents\Rohde-Schwarz\RTx\Temp\DataExpWfm.bin"
//store data in this path and filename
EXPort:WAVeform:RAW ON
//export as raw ADC integer values (saves memory) --> data needs to be converted later
EXPort:WAVeform:INCXvalues OFF
//disable time values in data file. Time can be constructed from the header file
CHANnel1:WAV1:HISTory:STATe ON //switch to history view
EXPort:WAVeform:DLOGging ON //enable data logging & history
EXPort:WAVeform:TIMestamps ON //enable relative time stamp for each acq.
CHANnel1:WAV1:HISTory:STARt -99 //oldest waveform of n acq. has index =(-1)*(n-1)
CHANnel1:WAV1:HISTory:STOP 0 //newest waveforms has index 0
CHANnel1:WAV1:HISTory:REPLay OFF
CHANnel1:WAV1:HISTory:PLAY; *OPC? //exports waveforms to defined file location.
//2 files are created: header file *.bin and waveform data file *.Wfm.bin
// --- Put files into output buffer of scope and collect ---
// The following code lines are mostly Pseudo Code.
//Sorting out the binary waveform data is more complex and require additional coding
binaryFormat = '1 byte'
//Pseudo Code, tell your language how to interpret the binary data,
//e.g. 'int8' for MATLAB
MMEM:DATA? "C:\Users\Public\Documents\Rohde-Schwarz\RTx\Temp\DataExpWfm.bin"
//Put header file into output buffer
header = readSCPIBinary(visaInstrument, binaryFormat);
//Pseudo Code, use appropriate command from your programming language,
//e.g. binblockread in MATLAB
MMEM:DATA? "C:\Users\Public\Documents\Rohde-Schwarz\RTx\Temp\DataExpWfm.Wfm.bin"
//Put data file into output buffer
//(your input buffer of the VISA resource might need to be increased)
wfmRaw = readSCPIBinary(visaInstrument, binaryFormat)
//Pseudo Code, use appropriate command from your programming language,
//e.g. binblockread in MATLAB.
//Note: Sort different acquisitions into an array separately after file transfer.
// --- Convert raw ADC values into voltage floating point values ---
// header is assumed to be a struct and the members are accessed via "." syntax.
vertOffsetByPosition = header.VerticalScale * header.VerticalPosition
conversionFactor = (1/header.NofQuantisationLevels) *
header.VerticalScale * header.VerticalDivisionCount
for(i = 0; i<100; i++){
wfmVolt(i) = wfmRaw(i) * conversion
header.VerticalOffset - vertOffsetByPosition
}
// Note: Depending on settings, the waveform can contain more samples than the
//record length. Remove leading and trailing samples from the waveform.
Programming examples