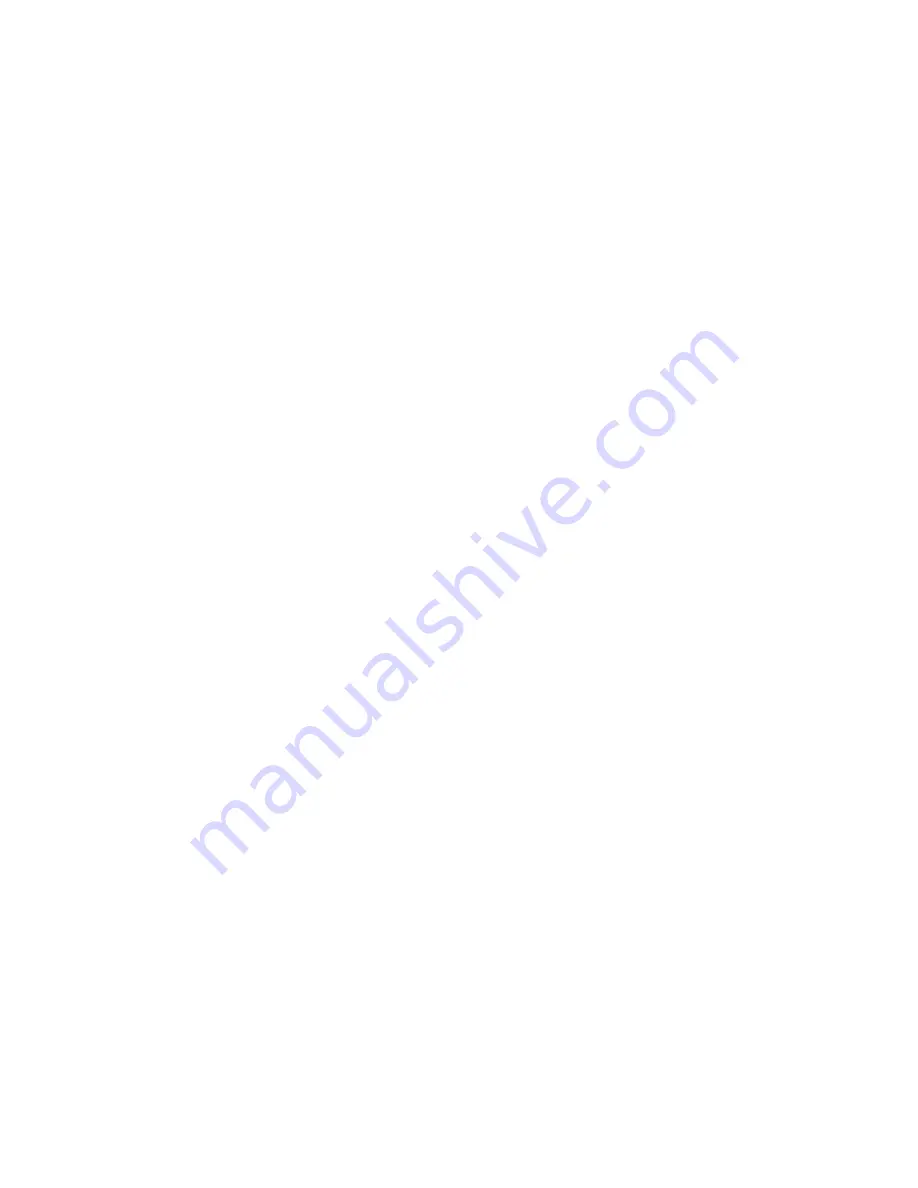
Programming Examples
40
Keysight InfiniiVision M9241/42/43A PXIe Oscilloscopes SCPI Programmer's Guide
1461
# Get the waveform data.
sData = do_query_ieee_block(":WAVeform:DATA?")
# Unpack unsigned byte data.
values = struct.unpack("%dB" % len(sData), sData)
print "Number of data values: %d" % len(values)
# Save waveform data values to CSV file.
f = open("waveform_data.csv", "w")
for i in xrange(0, len(values) - 1):
time_val = x_ (i * x_increment)
voltage = ((values[i] - y_reference) * y_increment) + y_origin
f.write("%E, %f\n" % (time_val, voltage))
f.close()
print "Waveform format BYTE data written to waveform_data.csv."
# =========================================================
# Send a command and check for errors:
# =========================================================
def do_command(command, hide_params=False):
if hide_params:
(header, data) = string.split(command, " ", 1)
if debug:
print "\nCmd = '%s'" % header
else:
if debug:
print "\nCmd = '%s'" % command
InfiniiVision.write("%s" % command)
if hide_params:
check_instrument_errors(header)
else:
check_instrument_errors(command)
# =========================================================
# Send a command and binary values and check for errors:
# =========================================================
def do_command_ieee_block(command, values):
if debug:
print "Cmb = '%s'" % command
InfiniiVision.write_binary_values("%s " % command, values, datatype='c'
)
check_instrument_errors(command)
# =========================================================
# Send a query, check for errors, return string:
# =========================================================
def do_query_string(query):
if debug:
print "Qys = '%s'" % query