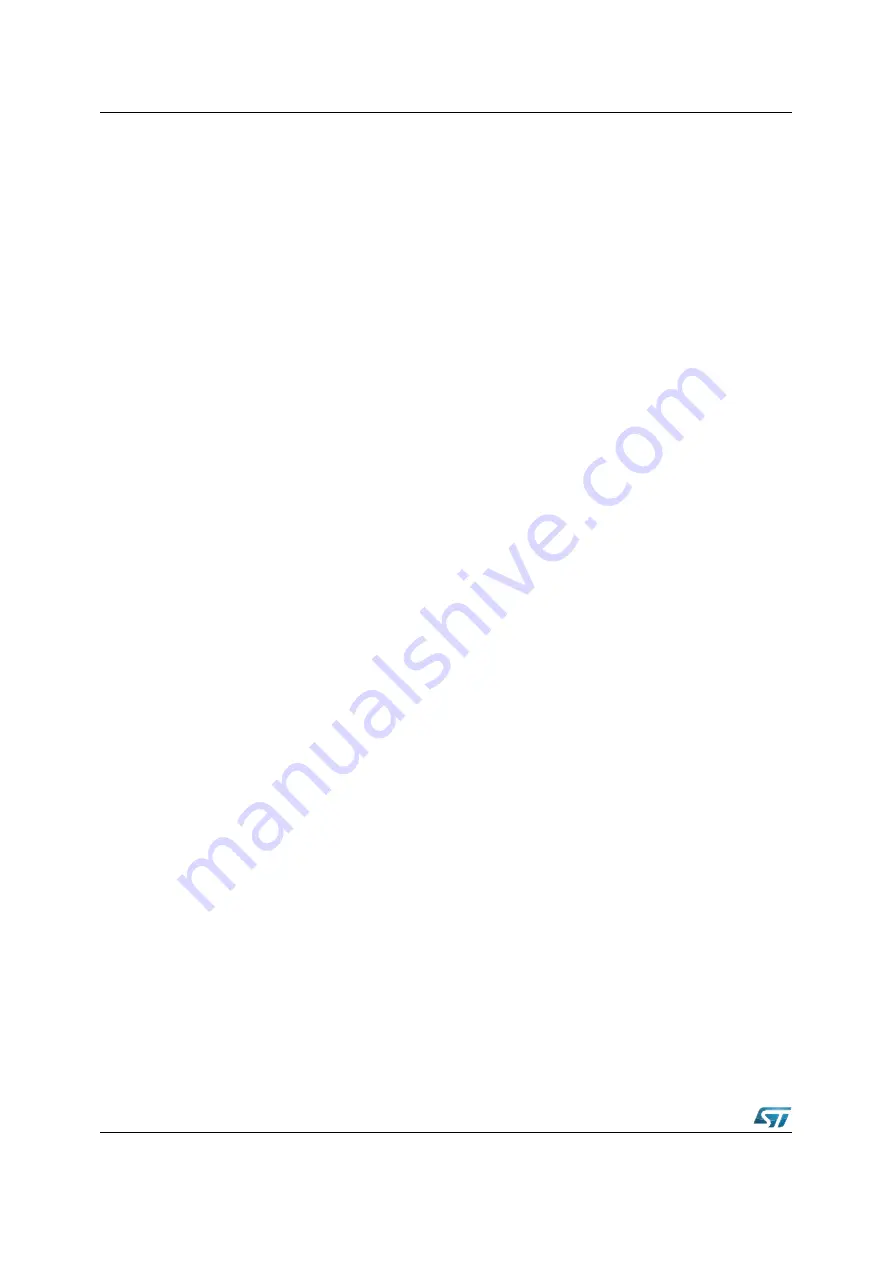
System programming
UM0404
DocID13284 Rev 2
Multiplication or division is simply performed by specifying the correct (signed or unsigned)
version of the multiply or divide instruction. The result is then stored in register MD.
The overflow flag (V) is set if the result from a multiply or divide instruction is greater than
16-bit. This flag can be used to determine whether both word halves must be transferred
from register MD.
The high portion of register MD (MDH) must be moved into the register file or memory first,
in order to ensure that the MDRIU flag reflects the correct state.
The following instruction sequence performs an unsigned 16 by 16-bit multiplication:
The above save sequence and the restore sequence after COPYL are only required if the
current routine could have interrupted a previous routine which contained a MUL or DIV
instruction. Register MDC is also saved because it is possible that a previous routine's
Multiply or Divide instruction was interrupted while in progress. In this case the information
about how to restart the instruction is contained in this register. Register MDC must be
cleared to be correctly initialized for a subsequent multiplication or division. The old MDC
contents must be popped from the stack before the RETI instruction is executed.
For a division the user must first move the dividend into the MD register. If a 16 by 16-bit
division is specified, only the low portion of register MD must be loaded.
The result is also stored into register MD. The low portion (MDL) contains the integer result
of the division, while the high portion (MDH) contains the remainder.
The following instruction sequence performs a 32 by 16-bit division:
...
SAVE:
JNB
MDRIU, START
;Test if MD was in use.
SCXT
MDC, #0010H
;Save and clear control register, leaving MDRIU set
;(only req for interrupted multiply/divide instructions)
BSET
SAVED
;Indicate the save operation
PUSH
MDH
;Save previous MD contents...
PUSH
MDL
;...on system stack
START:
MULU
R1, R2
;Multiply 16·16 unsigned, Sets MDRIU
JMPR
cc_NV, COPYL
;Test for only 16 Bit result
MOV
R3, MDH
;Move high portion of MD
COPYL:
MOV
R4, MDL
;Move low portion of MD, Clears MDRIU
RESTORE:
JNB
SAVED, DONE
;Test if MD registers were saved
POP
MDL
;Restore registers
POP
MDH
POP
MDC
BCLR
SAVED
;Multiplication is completed, program continues
DONE:
...
MOV
MDH, R1
;Move dividend to MD register. Sets MDRIU
MOV
MDL, R2
;Move low portion to MD
DIV
R3
;Divide 32/16 signed, R3 holds the divisor
JMPR
cc_V, ERROR
;Test for divide overflow
MOV
R3, MDH
;Move remainder to R3
MOV
R4, MDL
;Move integer result to R4. Clears MDRIU