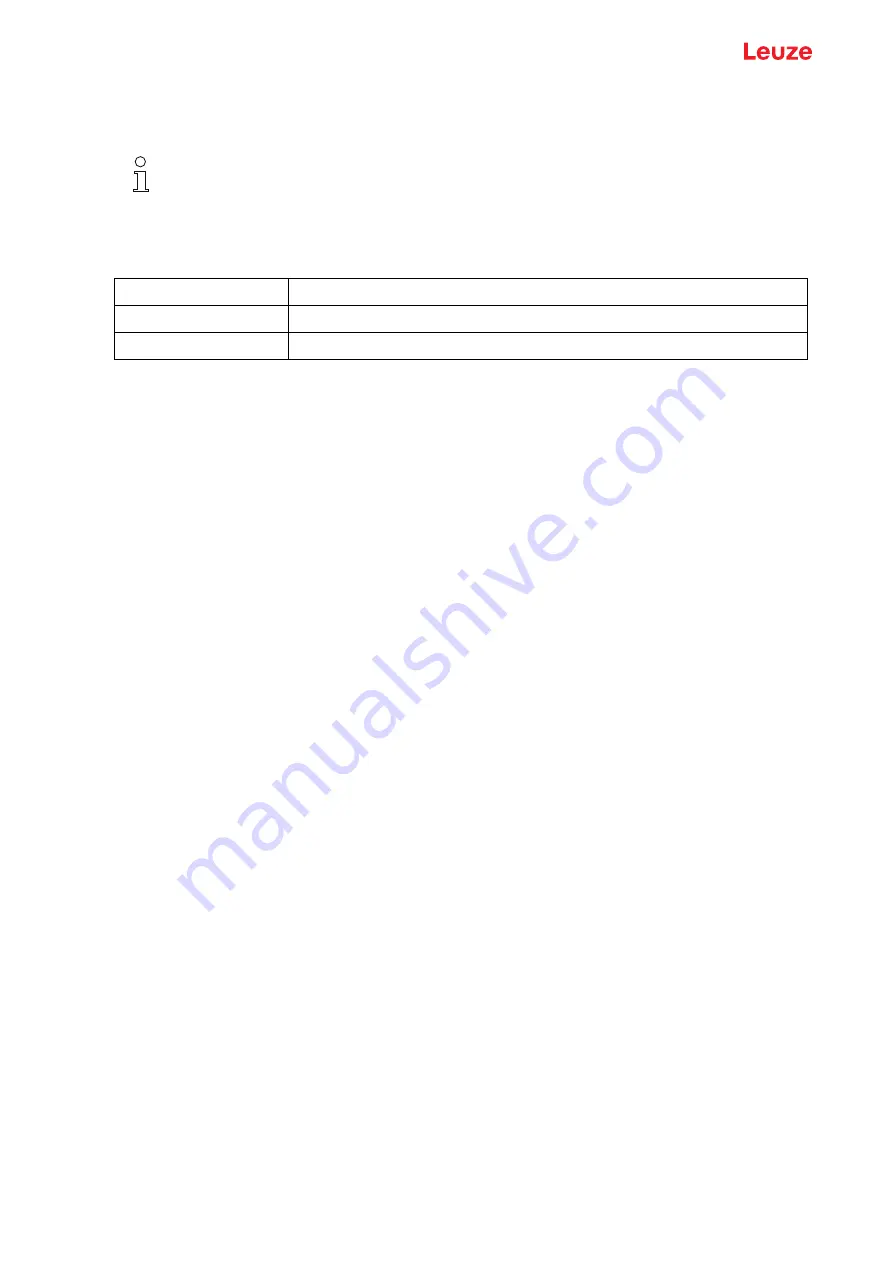
Starting up the device - RS 485 Modbus interface
Leuze electronic GmbH + Co. KG
CML 730i
147
Indexing the CRC in this way provides faster execution than would be achieved by calculating a new CRC
value with each new character from the message buffer.
The function takes two arguments:
CRC generation function:
High-order byte table:
This function performs the swapping of the high/low CRC bytes internally. The bytes are already
swapped in the CRC value that is returned from the function.
Therefore the CRC value returned from the function can be directly placed into the message for
transmission.
Argument
Description
unsigned char *puchMsg;
A pointer to the message buffer containing binary data to be used for generating the CRC
unsigned short usDataLen;
The quantity of bytes in the message buffer.
unsigned short CRC16 (puchMsg, usDataLen);
/* the function returns the CRC as a unsigned short type */
unsigned char *puchMsg;
/* message to calculate CRC upon */
unsigned short usDataLen;
/* quantity of bytes in message */
{
unsigned char uchCRCHi = 0xFF;
/* high byte of CRC initialized */
unsigned char uchCRCLo = 0xFF;
/* low byte of CRC initialized */
unsigned uIndex;
/* will index into CRC lookup table */
while (usDataLen--)
/* pass through message buffer */
{
uIndex = uchCRCLo ^ *p+ ;
/* calculate the CRC */
uchCRCLo = uchCRCHi ^ auchCRCHi[uIndex} ;
uchCRCHi = auchCRCLo[uIndex] ;
}
return (uchCRCHi << 8 | uchCRCLo) ;
}
/* table of CRC values for high-order byte */
static unsigned char auchCRCHi[] = {
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,
0x40,
0x00,
0xC1,
0x81,
0x40,
0x01,
0xC0,
0x80,
0x41,
0x00,
0xC1,
0x81,