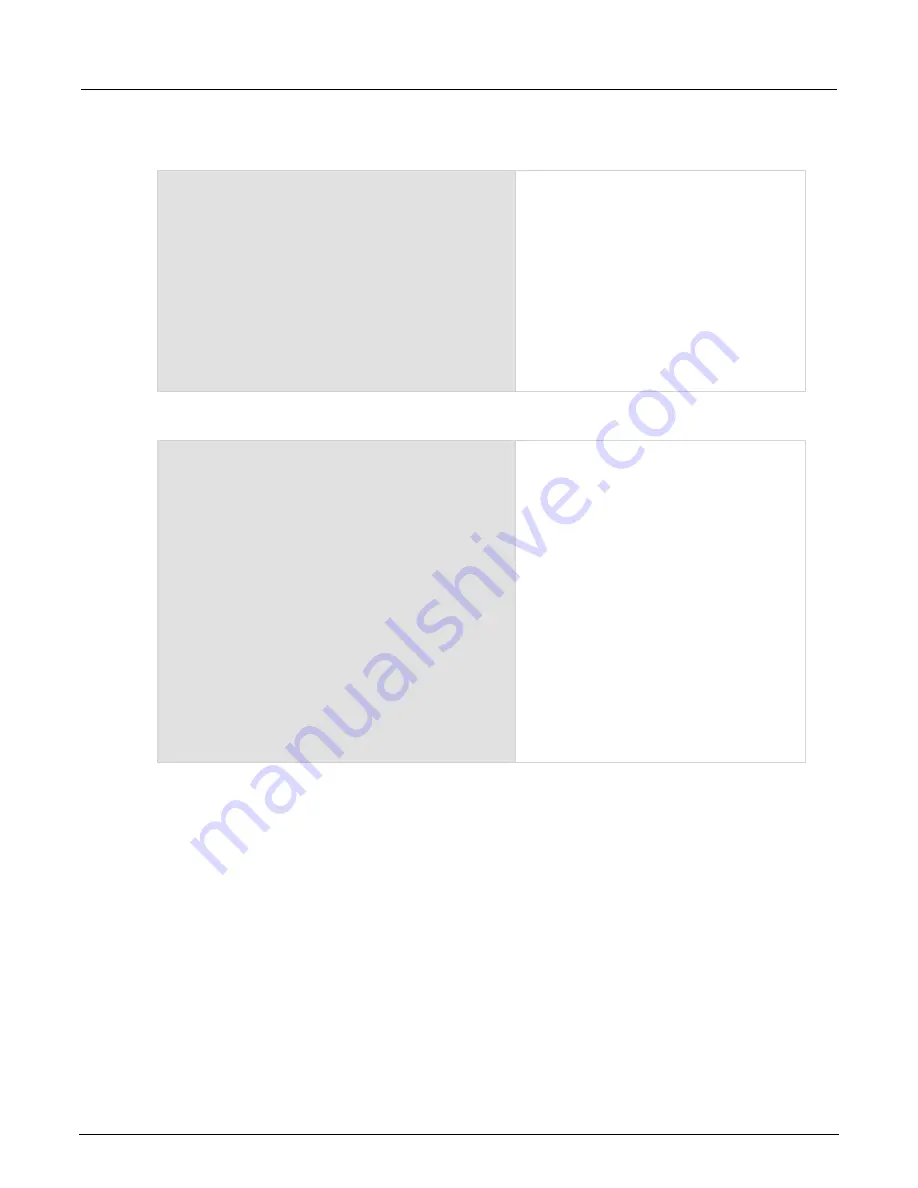
Section 13: Introduction to TSP commands
2470 High Voltage SourceMeter Instrument Reference Manual
13-26
2470-901-01 Rev. A /
May
2019
Example: Break with while statement enclosed by comment delimiters
local numTable = {5, 4, 3, 2, 1}
local k = table.getn(numTable)
--local breakValue = 3
while k > 0 do
if numTable[k] == breakValue then
print("Going to break and k = ", k)
break
end
k = k - 1
end
if k == 0 then
print("Break value not found")
end
This example defines a break value
(
breakValue
), but the break value line is
preceded by comment delimiters so that the
break value is not assigned, and the code
reaches the value
0
to exit the
while
loop.
Output:
Break value not found
Example: Break with infinite loop
a, b = 0, 1
while true do
print(a, b)
a, b = b, a + b
if a > 500 then
break
end
end
This example uses a
break
statement that
causes the while loop to exit if the value of
a
becomes greater than 500.
Output:
0 1
1 1
1 2
2 3
3 5
5 8
8 13
13 21
21 34
34 55
55 89
89 144
144 233
233 377
377 610
Tables and arrays
Lua makes extensive use of the data type table, which is a flexible array-like data type. Table indices
start with 1. Tables can be indexed not only with numbers, but with any value except
nil
. Tables can
be heterogeneous, which means that they can contain values of all types except
nil
.
Tables are the sole data structuring mechanism in Lua. They may be used to represent ordinary
arrays, symbol tables, sets, records, graphs, trees, and so on. To represent records, Lua uses the
field
name
as an index. The language supports this representation by providing
a.name
as an easier
way to express
a["name"]
.