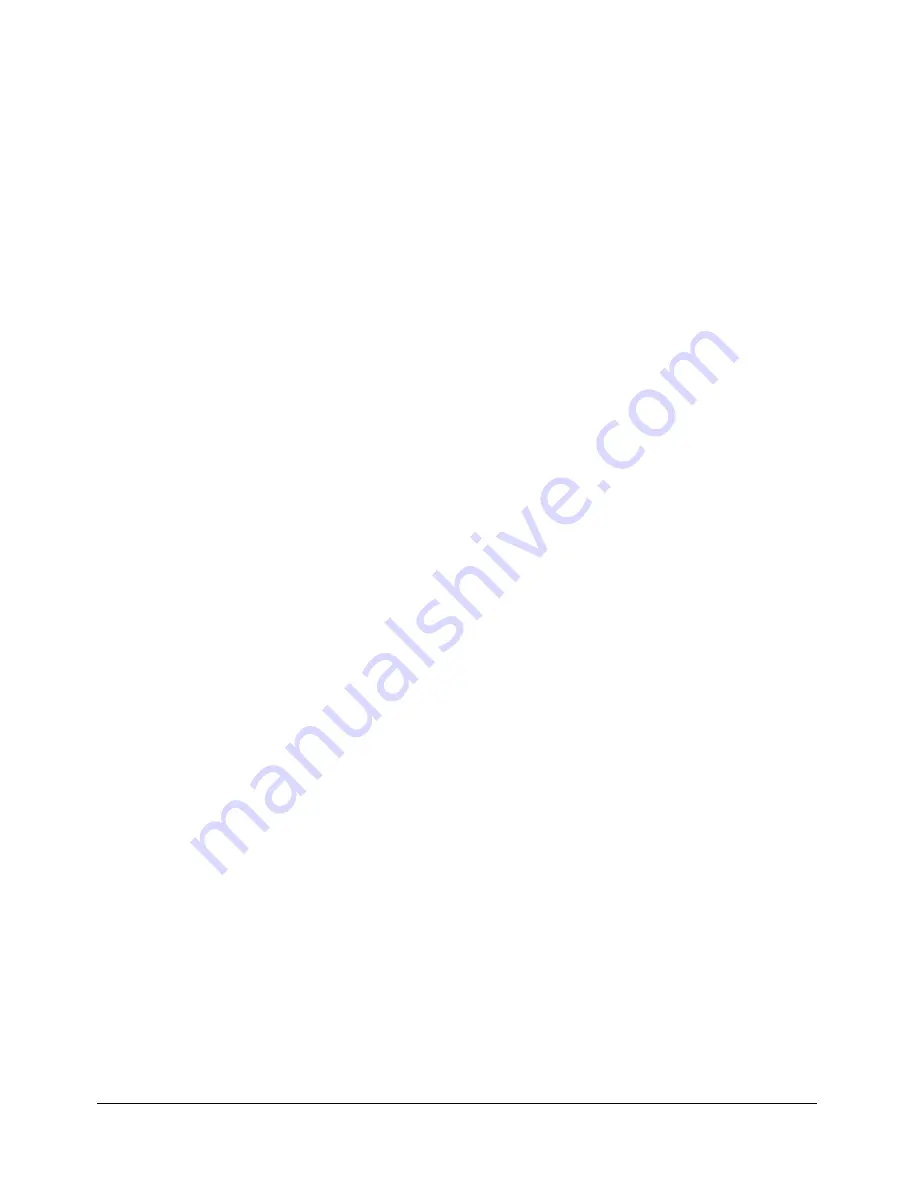
800
Appendix E: Object-Oriented Programming with ActionScript 1
Objects in ActionScript can be pure containers for data, or they can be graphically represented on
the Stage as movie clips, buttons, or text fields. All movie clips are instances of the built-in class
MovieClip, and all buttons are instances of the built-in class Button. Each movie clip instance
contains all the properties (for example,
_height
,
_rotation
,
_totalframes
) and all the
methods (for example,
gotoAndPlay()
,
loadMovie()
,
startDrag()
) of the MovieClip class.
To define a class, you create a special function called a
constructor function
. (Built-in classes have
built-in constructor functions.) For example, if you want information about a bicycle rider in
your application, you could create a constructor function,
Biker()
, with the properties
time
and
distance
and the method
getSpeed()
, which tells you how fast the biker is traveling:
function Biker(t, d) {
this.time = t;
this.distance = d;
this.getSpeed = function() {return this.time / this.distance;};
}
In this example, you create a function that needs two pieces of information, or parameters, to do
its job:
t
and
d
. When you call the function to create new instances of the object, you pass it the
parameters. The following code creates instances of the object Biker called
emma
and
hamish
.
emma = new Biker(30, 5);
hamish = new Biker(40, 5);
In object-oriented scripting, classes can receive properties and methods from each other according
to a specific order; this is called
inheritance
. You can use inheritance to extend or redefine the
properties and methods of a class. A class that inherits from another class is called a
subclass
. A
class that passes properties and methods to another class is called a
superclass
. A class can be both a
subclass and a superclass.
An object is a complex data type containing zero or more properties and methods. Each property,
like a variable, has a name and a value. Properties are attached to the object and contain values
that can be changed and retrieved. These values can be of any data type: String, Number,
Boolean, Object, MovieClip, or undefined. The following properties are of various data types:
customer.name = "Jane Doe";
customer.age = 30;
customer.member = true;
customer.account.currentRecord = 000609;
customer.mcInstanceName._visible = true;
The property of an object can also be an object. In line 4 of the previous example,
account
is a
property of the object
customer
and
currentRecord
is a property of the object
account
. The
data type of the
currentRecord
property is Number.
Creating a custom object in ActionScript 1
To create a custom object, you define a constructor function. A constructor function is always
given the same name as the type of object it creates. You can use the keyword
this
inside the
body of the constructor function to refer to the object that the constructor creates; when you call
a constructor function, Flash passes it
this
as a hidden parameter. For example, the following is a
constructor function that creates a circle with the property
radius
:
function Circle(radius) {
this.radius = radius;
}
Summary of Contents for FLASH MX 2004 - ACTIONSCRIPT
Page 1: ...ActionScript Reference Guide...
Page 8: ...8 Contents...
Page 12: ......
Page 24: ...24 Chapter 1 What s New in Flash MX 2004 ActionScript...
Page 54: ...54 Chapter 2 ActionScript Basics...
Page 80: ...80 Chapter 3 Writing and Debugging Scripts...
Page 82: ......
Page 110: ...110 Chapter 5 Creating Interaction with ActionScript...
Page 112: ......
Page 120: ...120 Chapter 6 Using the Built In Classes...
Page 176: ......
Page 192: ...192 Chapter 10 Working with External Data...
Page 202: ...202 Chapter 11 Working with External Media...
Page 204: ......
Page 782: ...782 Chapter 12 ActionScript Dictionary...
Page 793: ...Other keys 793 221 222 Key Key code...
Page 794: ...794 Appendix C Keyboard Keys and Key Code Values...
Page 798: ...798 Appendix D Writing Scripts for Earlier Versions of Flash Player...
Page 806: ...806 Appendix E Object Oriented Programming with ActionScript 1...
Page 816: ...816 Index...