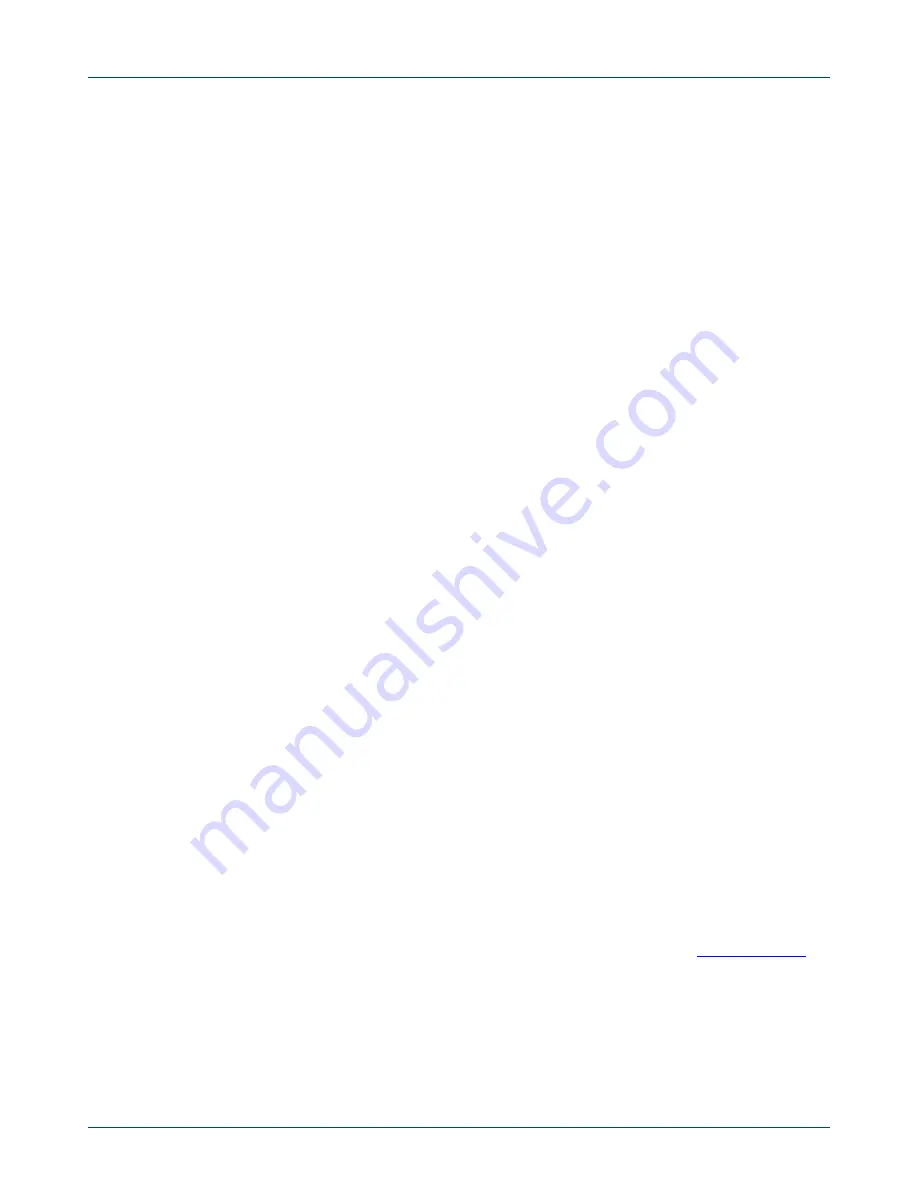
UM10800
All information provided in this document is subject to legal disclaimers.
© NXP Semiconductors N.V. 2016. All rights reserved.
User manual
Rev. 1.2 — 5 October 2016
411 of 487
NXP Semiconductors
UM10800
Chapter 28: LPC82x SPI API ROM driver routines
28.5.2 Example (using DMA)
The DMA sends and receives characters via the SPI in master mode. Use the SPI API
and DMA API as follows:
1. Assign the SCK, MOSI, MISO, and SSEL0 functions to pins in the switch matrix.
2. Global defines:
SPID_API_T * pSpiApi ; //define pointer to type API function addr table
SPI_HANDLE_T* spi_handle; //handle to SPI API
SPI_PARAM_T param;
SPI_CONFIG_T spi_cfg;
SPI_DMA_CFG_T dmc_cfg;
#define RAMBLOCK_H 60
uint32_t start_of_ram_block0[ RAMBLOCK_H ] ;
#define BUFFER_SIZE 100
uint32_t adc_buffer[BUFFER_SIZE];
3. Initialize pointer to the SPI API:
pSpiApi = (SPID_API_T *) (rom_drivers_ptr->pSPID);
4. Initialize SPI:
size_in_bytes = pSpiApi->spi_get_mem_size() ; //size_in_bytes/4 must be
// < RAMBLOCK_H
spi_handle = pSpiApi->spi_setup(LPC_SPI0_BASE, (uint8_ *)start_of_ram_block0);
5. Set up the DMA:
size_in_bytes = pDmaApi->dma_get_mem_size(); //size_in_bytes/4 must be
// < RAMBLOCK_H
pDmaApi = (DMAD_API_T *) (rom_drivers_ptr->pDMAD);
align_location = (uint32_t *)0x02008000;
dma_handle = pDmaApi->dma_setup(LPC_DMA_BASE, (uint8_t *)align_location);
6. Set up the SPI0 in DMA mode by defining the SPI_CONFIG_T structure:
spi_set.delay =
DLY_PREDELAY(0x0)|DLY_POSTDELAY(0x0)|DLY_FRAMEDELAY(0x0)|DLY_INTERDELAY(0x0);
spi_set.divider = 0xFFFF; // divide system clock by 0xFFFF for SCK
spi_set.config = CFG_MASTER; //master mode
spi_set.error_en = STAT_RXOVERRUN | STAT_TXUNDERRUN; //disable
// error interrupts - these are only needed in slave mode
7. Set-up the DMA_CONFIG_T structure for this DMA transfer (see
dma_cfg.dma_txd_num = DMAREQ_SPI0_TX;
dma_cfg.dma_rxd_num = DMAREQ_SPI0_RX;
dma_cfg.dma_handle = (uint32_t)dma_handle;
8. Initialize SPI transfer:
pSpiApi->spi_init(spi_handle, &spi_set);
9. Set up the SPI parameter structure SPI_PARAM_T for the SPI: