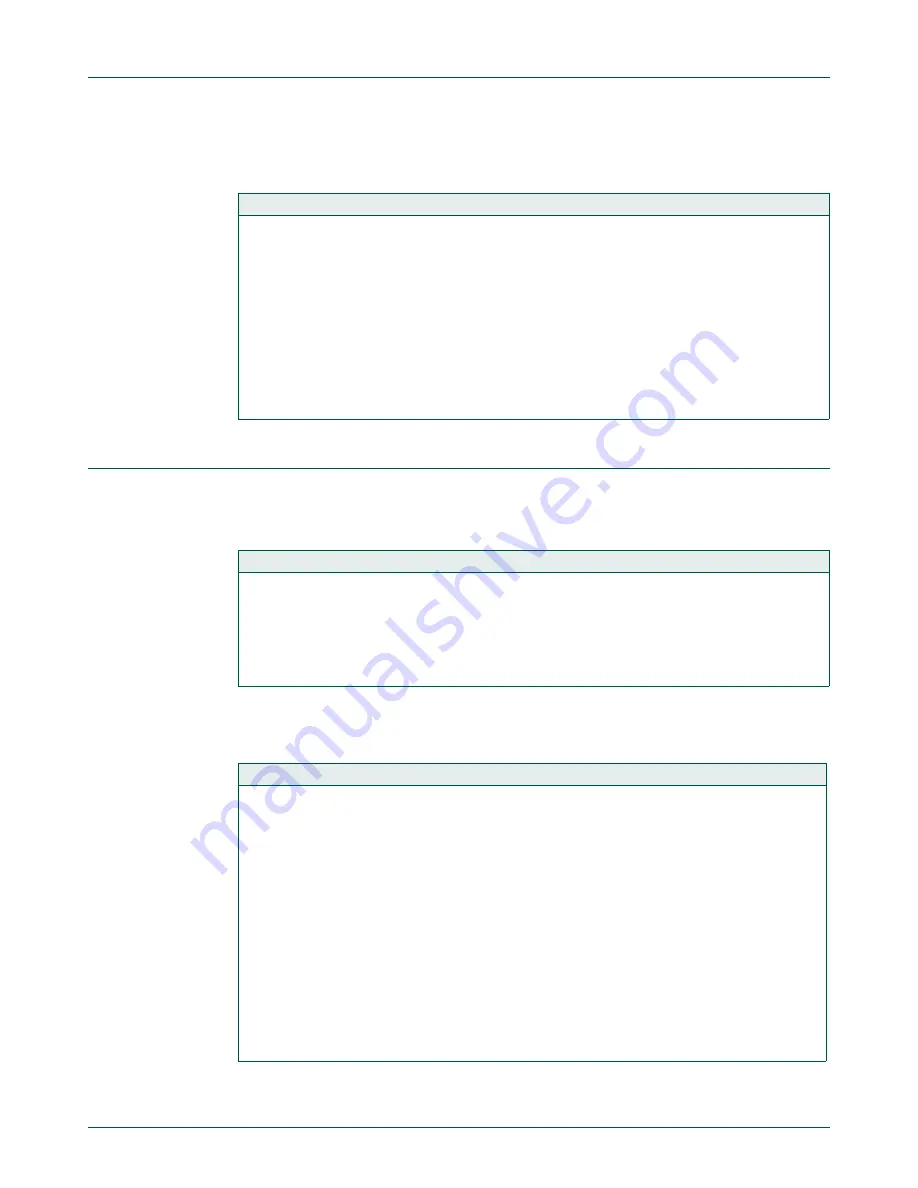
UM10800
All information provided in this document is subject to legal disclaimers.
© NXP Semiconductors N.V. 2016. All rights reserved.
User manual
Rev. 1.2 — 5 October 2016
464 of 487
NXP Semiconductors
UM10800
Chapter 34: LPC82x Code examples
34.3.10 Transmit and receive 24 bits to master
34.4 Code examples UART
34.4.1 Definitions
34.4.2 Interrupt handler
Table 431. SPI Code example
Transmit and receive 24 bits to master.
LPC_SPI->CFG = SPI_CFG_ENABLE;
while(~LPC_SPI->STAT & SPI_STAT_TXRDY);
LPC_SPI->TXDATCTL = SPI_TXDATCTL_FLEN(15) | 0xdddd;
while(~LPC_SPI->STAT & SPI_STAT_TXRDY);
LPC_SPI->TXDATCTL = SPI_TXDATCTL_FLEN(7) | 0xdd;
while(~LPC_SPI->STAT & SPI_STAT_RXRDY);
data = LPC_SPI->RXDAT;
if(data != 0xdddd) abort();
while(~LPC_SPI->STAT & SPI_STAT_RXRDY);
data = LPC_SPI->RXDAT;
if(data != 0xdd) abort();
Table 432. UART Code example
UART defines
#define UART_CFG_ENABLE (0x1 << 0)
#define UART_CFG_DATALEN(d) (((d) - 7) << 2)
#define UART_STAT_RXRDY (0x1 << 0)
#define UART_STAT_TXRDY (0x1 << 2)
#define UART_STAT_TXIDLE (0x1 << 3)
Table 433. UART Code example
Interrupt handler
void Usart_IRQHandler() {
uint32_t intstat = LPC_USART->INTSTAT;
if(intstat & UART_STAT_RXRDY) {
if(!tx_rdy_flag) abort();
tx_rdy_flag = 0;
LPC_USART->TXDAT = LPC_USART->RXDAT;
LPC_USART->INTENSET = UART_STAT_TXRDY;
tx_+;
}
if(intstat & UART_STAT_TXRDY) {
if(tx_rdy_flag) abort();
tx_rdy_flag = 1;
LPC_USART->INTENCLR = UART_STAT_TXRDY;
}
}