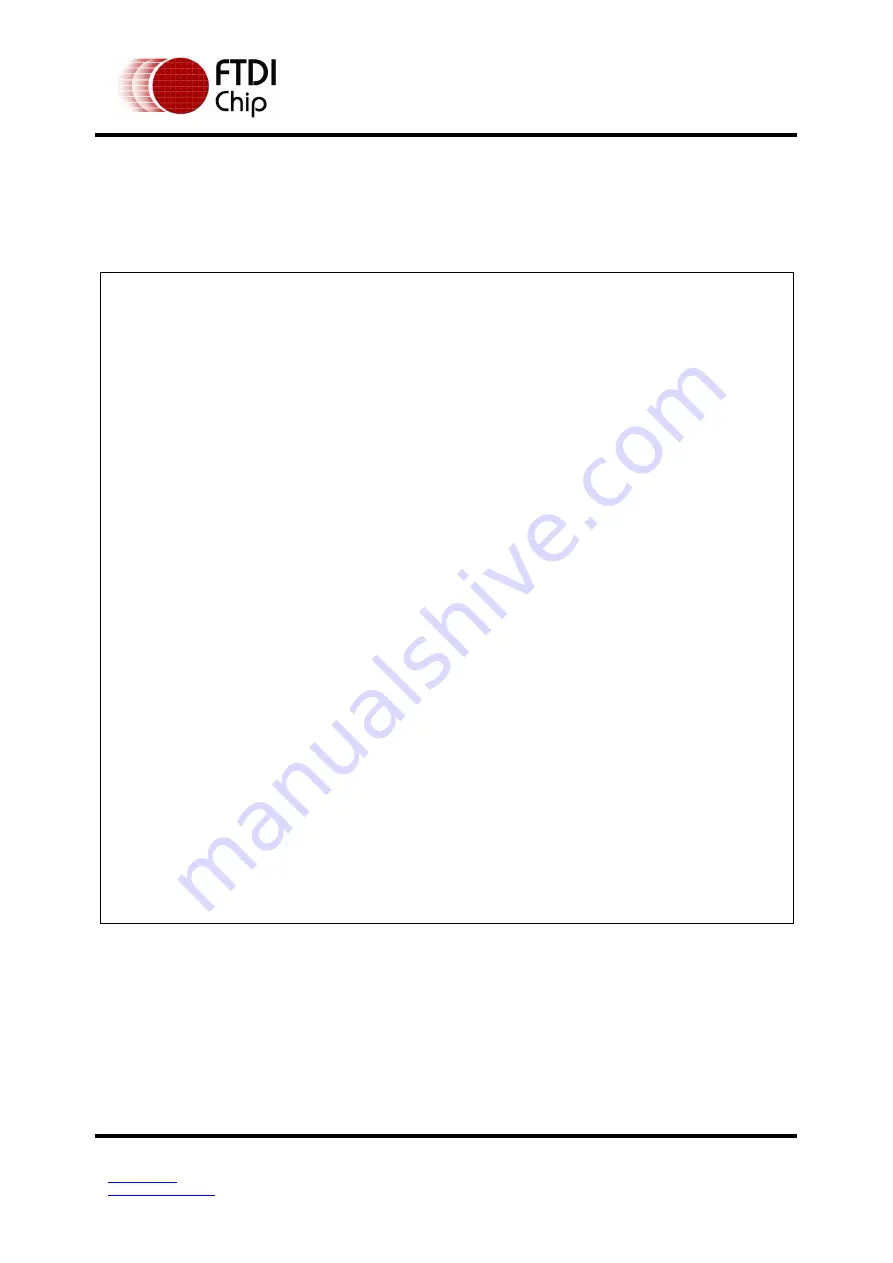
Application Note
AN_289 FT51A Programming Guide
Version 1.0
Document Reference No.: FT_000962 Clearance No.: FTDI# 483
157
Copyright © 2015 Future Technology Devices International Limited
3.2.4
Class and Vendor Requests
Like the standard request handler, class and vendor request handlers must return FT51_OK if the
request was handled by the application or FT51_FAILED if not.
The format of the class and vendor request functions is the same as the standard requests. This
example provides a class handler for a HID keyboard.
uint8_t input_report;
uint8_t report_mode;
FT51_STATUS
class_req_cb
(USB_device_request *req)
{
FT51_STATUS status = FT51_FAILED;
uint8_t interface = LSB(req->wIndex) & 0x0F;
// Ensure the recipient is an interface...
// Can also check if the interface number is correct
if
((req->bmRequestType & USB_BMREQUESTTYPE_RECIPIENT_MASK) ==
USB_BMREQUESTTYPE_RECIPIENT_INTERFACE)
{
// Handle HID class requests
switch
(req->bRequest)
{
case
USB_HID_REQUEST_CODE_SET_IDLE:
// Turn on report mode to start sending data to host
report_mode = 1;
USB_transfer(
USB_EP_0
,
USB_DIR_IN
, NULL, 0);
status =
FT51_OK
;
break
;
case
USB_HID_REQUEST_CODE_SET_PROTOCOL:
USB_transfer(
USB_EP_0
,
USB_DIR_IN
, NULL, 0);
status =
FT51_OK
;
break
;
case
USB_HID_REQUEST_CODE_SET_REPORT:
// dummy read of one byte
USB_transfer(
USB_EP_0
,
USB_DIR_OUT
, &input_report, 1);
// Acknowledge SETUP
USB_transfer(
USB_EP_0
,
USB_DIR_IN
, NULL, 0);
status =
FT51_OK
;
break
;
}
}
}
return
status;
}
3.2.5
Call-backs
The suspend, resume and reset call-backs are optional.
3.2.5.1
Reset Call-back
The reset call-back is needed when the device is running self-powered. The USB state is part of
the library rather than an internal state in the USB Full Speed device controller and therefore
needs to update when the device is reset.