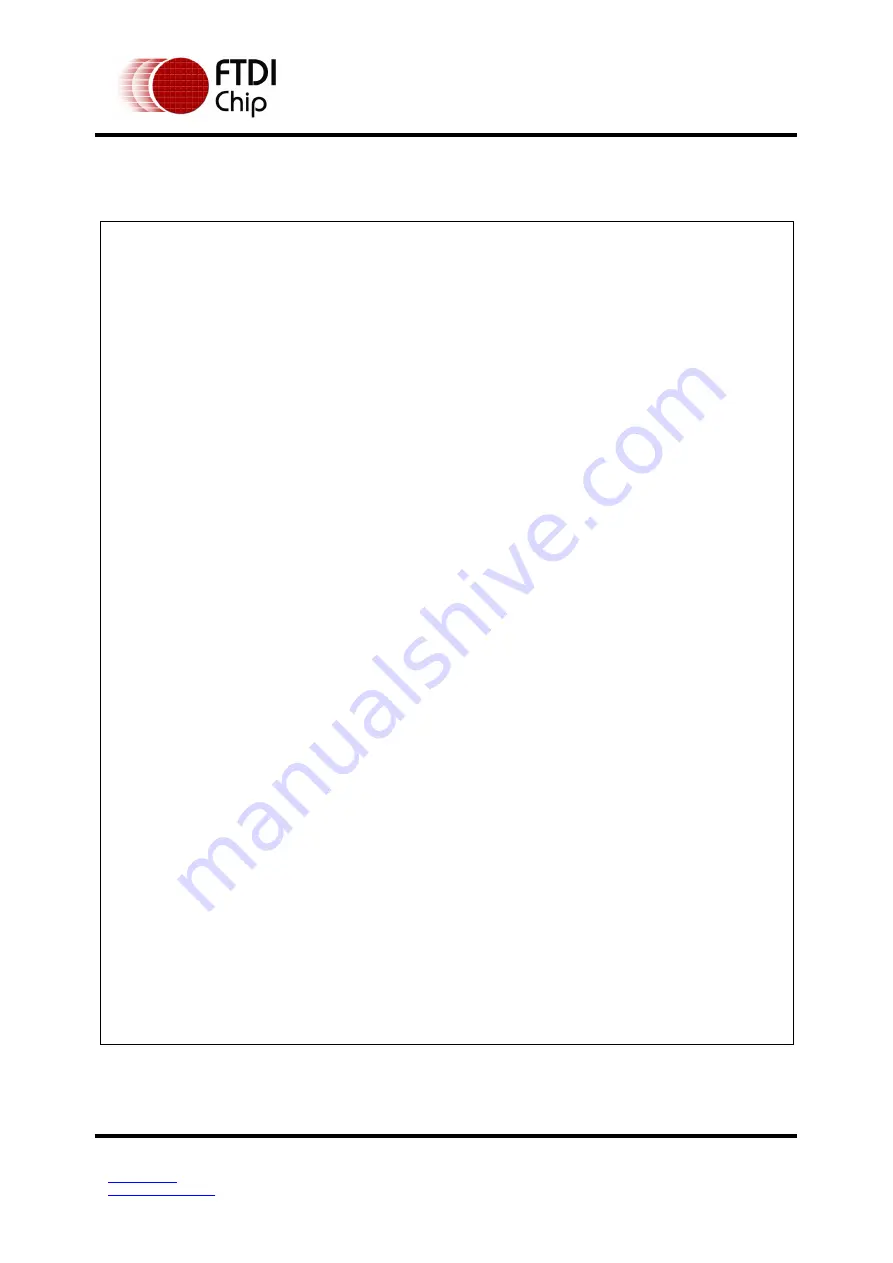
Application Note
AN_289 FT51A Programming Guide
Version 1.0
Document Reference No.: FT_000962 Clearance No.: FTDI# 483
156
Copyright © 2015 Future Technology Devices International Limited
A simple handler for device, configuration and string descriptors would be as follows. It is assumed
that the byte arrays “config_descriptor”, “device_descriptor” and “string_descriptor” are defined.
See sections 3.2.2, 3.2.2.2 and 3.2.2.3.
FT51_STATUS
standard_req_get_descriptor
(USB_device_request *req)
{
// pointer to descriptor (or part of) to send to host
uint8_t *src = NULL;
// length of data requested by the host
uint16_t length = req->wLength;
uint8_t hValue = req->wValue >> 8;
uint8_t lValue = req->wValue & 0x00ff;
uint8_t I, slen;
switch
(hValue)
{
case
USB_DESCRIPTOR_TYPE_DEVICE:
src = (
char
*)&device_descriptor;
if (length > sizeof(USB_device_descriptor)) // too many bytes requested
length = sizeof(USB_device_descriptor); // Entire structure.
break;
break
;
case
USB_DESCRIPTOR_TYPE_CONFIGURATION:
src = (
char
*)&config_descriptor;
if (length > sizeof(config_descriptor)) // too many bytes requested
length = sizeof(config_descriptor); // Entire structure.
break
;
case
USB_DESCRIPTOR_TYPE_STRING:
// Find the nth string in the string descriptor table
i = 0;
while
((slen = string_descriptor[i]) > 0) {
// Point to start of string descriptor in __code segment
src = (uint8_t *)&string_descriptor[i];
if
(lValue == 0) {
break
;
}
i += slen;
lValue--;
}
if
(lValue > 0) {
return
FT51_FAILED
; // String not found
}
// Update the length returned only if it is less than the requested
// size
if
(length > slen) {
length = slen;
}
break
;
default
:
return
FT51_FAILED
;
}
USB_transfer(USB_EP_0, USB_DIR_IN, src, length);
return
FT51_OK
;
}
The device and configuration descriptors are defined as byte arrays in the __code segment, the
string descriptor is an array of byte arrays in the __code segment.