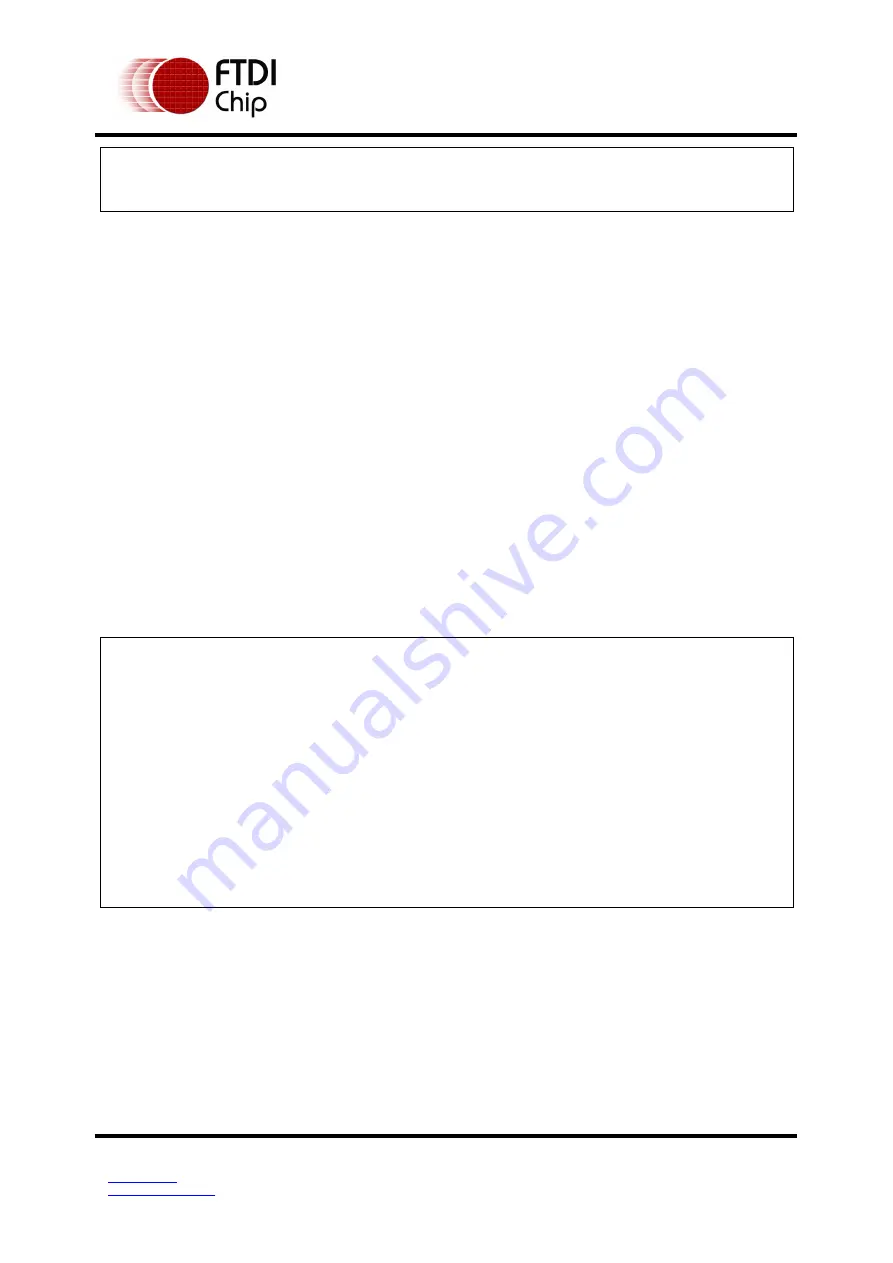
Application Note
AN_289 FT51A Programming Guide
Version 1.0
Document Reference No.: FT_000962 Clearance No.: FTDI# 483
151
Copyright © 2015 Future Technology Devices International Limited
// Perform USB Device initialisation.
USB_initialise(&usb_ctx);
}
The USB_ctx structure passes the required and optional values to the initialization routine. The
only call-back required is the standard_req callback. This handles standard requests.
The ep_size must also be specifically set to match the control endpoint bMaxPacketSize0 in the
device descriptor returned to the host. The default value is 8 bytes that correlate with a value 0 in
the USB_ENDPOINT_SIZE enumeration in ft51_usb.h.
When using the hub functionality, the hub must be configured to use the “compound device”
unless the USB device application can be disabled.
3.2.2
Descriptors
The descriptors for the USB device are used in response to standard requests. There are typedefs
in ft51_usb.h for the common standard descriptors. The typedefs can be used to initialise
descriptors or byte arrays can be used instead.
It is useful to store descriptors in __code space as having them as automatic variables will mean
that they are copied to the __data or __xdata areas. This will use memory resources. Typically the
descriptors will not be changed by an application.
3.2.2.1
Device Descriptors
The device descriptor uses USB_device_descriptor typedef from ft51_usb.h. It provides a structure
that can be initialised with values for the device descriptor.
__code
USB_device_descriptor device_descriptor =
{
.bLength = 0x12,
.bDescriptorType = 0x01,
.bcdUSB = USB_BCD_VERSION_2_0,
.bDeviceClass = USB_CLASS_DEVICE,
.bDeviceSubClass = USB_SUBCLASS_DEVICE,
.bDeviceProtocol = USB_PROTOCOL_DEVICE,
.bMaxPacketSize0 = 8, // MUST match the control endpoint size
.idVendor = USB_VID_FTDI, // idVendor: 0x0403 (FTDI)
.idProduct = USB_PID_DEVICE,// idProduct: User defined.
.bcdDevice = 0x0101,
.iManufacturer = 0x01, // Manufacturer String
.iProduct = 0x02, // Product Stirng
.iSerialNumber = 0x03, // Serial Number String
.bNumConfigurations = 0x01,
};
The idVendor and idProduct values (the USB VID and PID)
must
be unique to the application. FTDI
applications for the FT51A use the FTDI VID and an example PID.
FTDI can assign a valid PID on request. FT51A applications assign PIDs in the range 0x0FE0 to
0x0FEF.
Do not
use these values in a final product.
3.2.2.2
Configuration Descriptors
The ft51_usb.h file has typedefs for several configuration descriptor types. These can be combined
in a struct to make a memory structure which can be initialised in code.
A sample configuration descriptor for a keyboard would be: