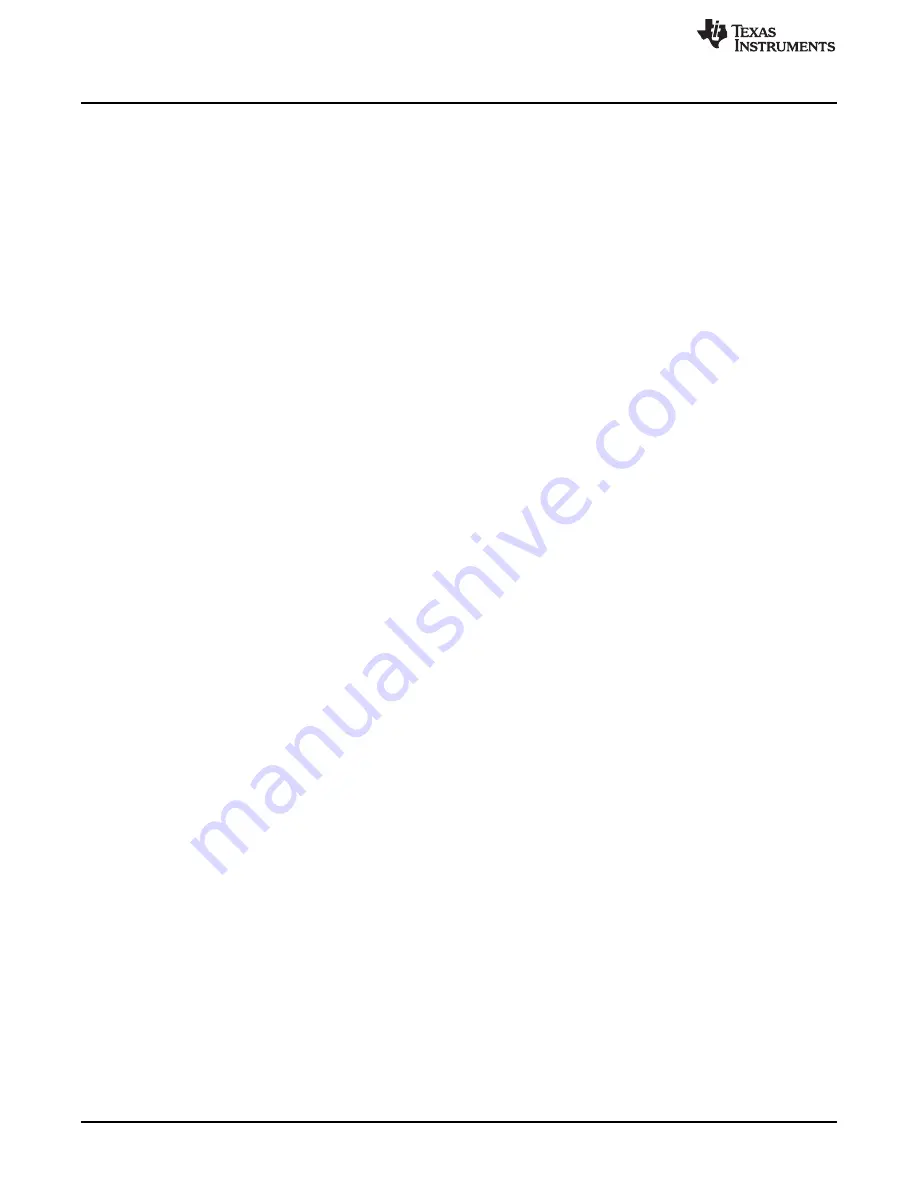
Firmware Examples
524
SNIU028A – February 2016 – Revised April 2016
Copyright © 2016, Texas Instruments Incorporated
Flash Memory Programming, Integrity, and Security
17.5.2 Erasing Flash
The code for erasing flash is very similar, except instead of writing to the program flash word, it actually
writes to the program flash control register and does a mass erase.
The C calling function is very similar. Only the labels for the code which is copied change.
Here is the code to clear the program flash:
void clear_program_flash(void)
{
DecRegs.PFLASHCTRL.bit.MASS_ERASE = 1; //erase it all
while(DecRegs.PFLASHCTRL.bit.BUSY != 0)
{
; //do nothing while it programs
}
return;
}
17.5.3 Serial Port Based Backdoor
Here is the code for a back door based on the serial RX line, as used in the UCD3138 training labs:
void main()
{
if(GioRegs.FAULTIN.bit.TMS_IN == 0) //emergency backdoor -
//TMS is normally pulled up by external resistor
{
clear_integrity_word();
//if it's pulled down, clear checksum (integrity word)
}
17.5.4 I/O Line Based Back Door
Here is code which actually uses 2 I/O lines, used in the LLC EVM:
void main()
{
//Recommended setting
MiscAnalogRegs.CLKTRIM.bit.HFO_LN_FILTER_EN = 0;
//Turn on PMBus address pin current source ASAP to allow time to charge up.
PMBusRegs.PMBCTRL3.bit.IBIAS_B_EN = 1;
//Disable all DPWM outputs.
global_disable();
//Check to see if FAULT2 is pulled high and FAULT0 is pulled low.
//If they are go to ROM.
if (MiscAnalogRegs.GLBIOREAD.bit.FAULT2_IO_READ &&
!MiscAnalogRegs.GLBIOREAD.bit.FAULT3_IO_READ)
{
rom_back_door();
}
Notice that the code is placed very close to the start of the main function. Only time critical elements are
placed before it. This code uses rom_back_door, which first disables all the DPWMs and then calls
clear_integrity_word. In this case the function is the same as the previous example.