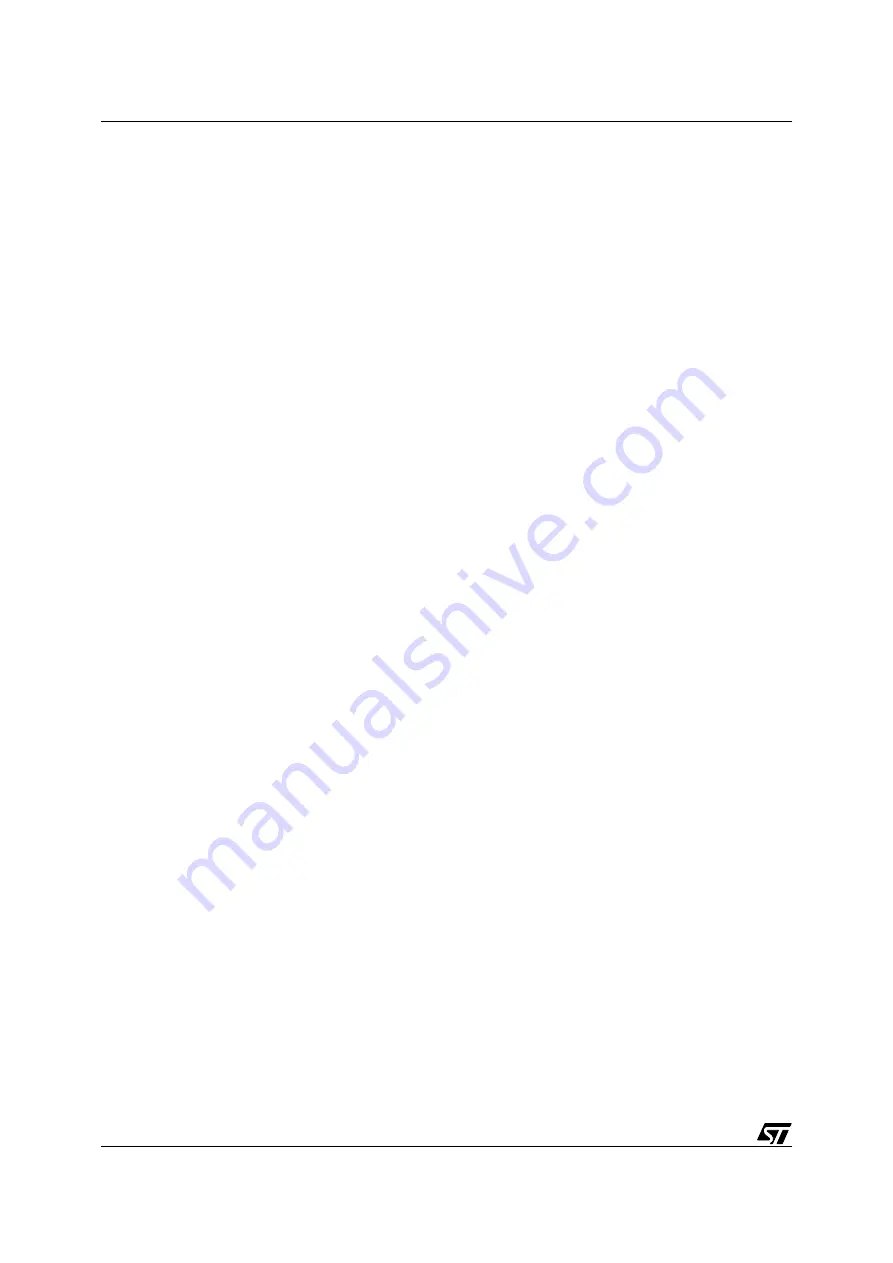
244/317
8 - C Language and the C Compiler
Some registers hold numeric values, like
TAOC2HR
or
TAOC2LR
; in such registers, reading or
writing a value is done using an assignment statement:
TAOC2HR = TimerAPeriod >> 8 ;
/* Set new period as calculated in
the capture */
TAOC2LR = TimerAPeriod & 0xff ;
/* interrupt service function. High
*MUST* be written first. */
or:
CurrentPeriod = TAOC2LR ;
Other registers, on the contrary, are made of a set of 8 independent bits that are grouped to
occupy only one address in the addressable space. This the case for example of configuration
registers like
TACR1
,
TACR2
and
TASR
. For these registers, a set of
#define
statements asso-
ciate the name of each bit (e.g.
OC1E
) with its position within the register (here, 7).
Since the ST7 does not provide for individual bit addresses, to reach a single bit it is necessary
to give the name of the register that holds that bit, and the number of the bit in the register.
To test the state of a single bit, the following syntax is suggested:
if ( TASR & ( 1 << ICF1 ) )
/* Determine which interrupt cause. */
where the expression
( 1 << ICF1 )
yields the value
0x80
(1 shifted left seven times); anding
the whole register with this value yields a non-zero result if the bit is true, a zero value other-
wise. This result is correctly tested using an
if
statement, since C defines a boolean true as
anything different from zero.
To set a particular bit within a register, the following syntax works well:
TACR1 |= ( 1 << OLVL1 ) ;
/* Validate pulse for next interrupt */
The register is ored with the 1, shifted by the appropriate number of bits; the result is written
back into the register.
To reset the bit, this syntax produces the reverse effect:
TACR1 &= ~( 1 << OLVL1 ) ;
/* Invalidate pulse for next interrupt */