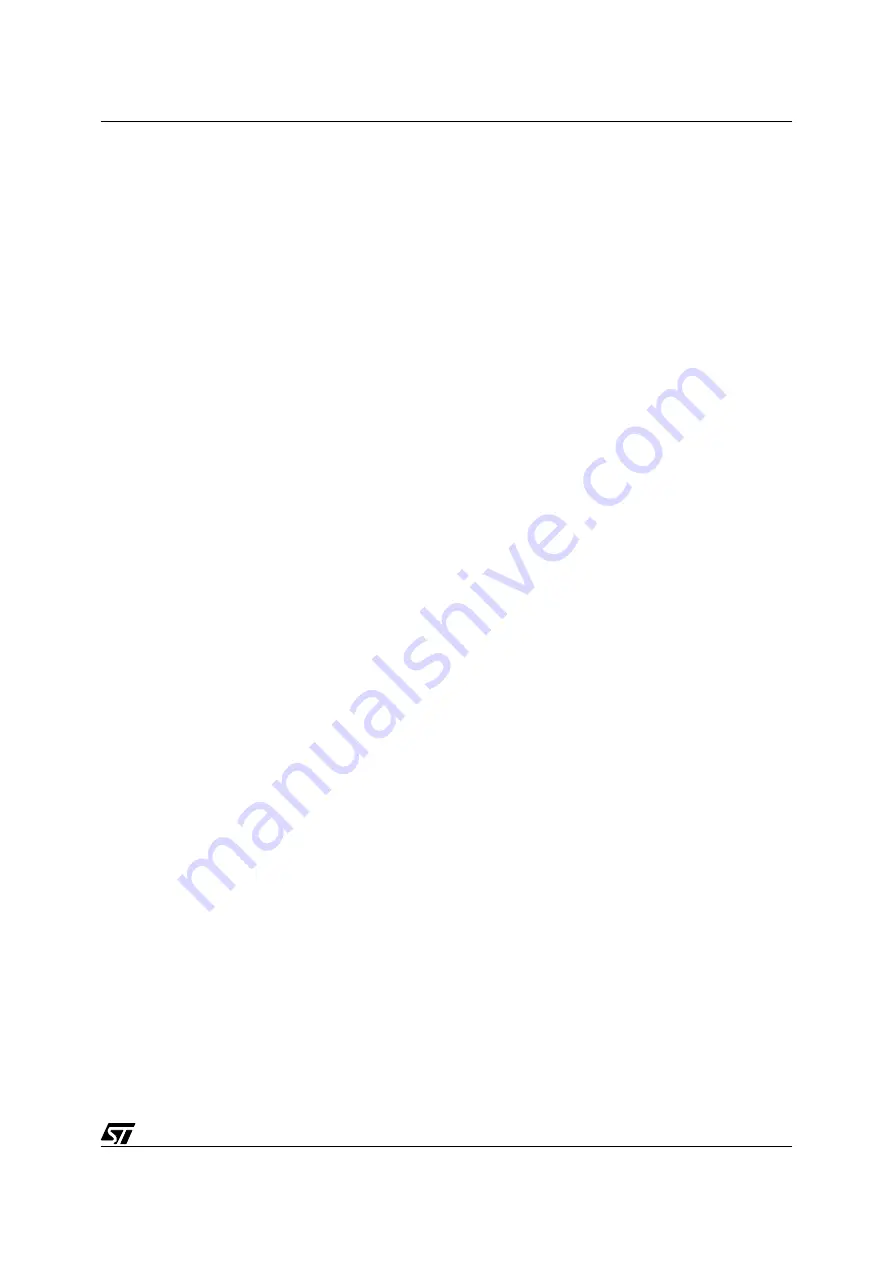
309/317
10 - Second Application: a Sailing Computer
10.5.7 Handling of the serial interface
The serial interface is configured to both transmit and receive under interrupt control. The con-
figuration function is the following:
void InitSCI ( void )
{
SCICR1 = 0 ;
/* 8 bits, one stop */
SCIBRR= ( 3 << 6 ) + ( 0 << 3 ) + 0 ;
/* Send & receive at 9600 bps */
SCICR2 =
( 0 << TIE )
/* Transmit interrupts disabled
(temporarily) */
| ( 1 << RIE )
/* Receive interrupts enabled */
| ( 1 << TE )
/* Transmitter enabled */
| ( 1 << RE ) ;
/* Receiver enabled */
}
Both transm it and receive interrupts are used. However, the transmit interrupt request is
present as soon as the transmit register is empty, which is always the case when nothing is
being sent. Thus, the transmit interrupt is always masked out except when there is something
to send.
The bit rate is supplied by the normal rate generator, applying only a division by 13, which after
the wired divider by 32, gives a bit rate of 9600 from a 4 MHz internal clock. The pins corre-
sponding to the input and output of the SCI are configured as floating inputs.
The interrupt service function is the following:
#pragma TRAP_PROC SAVE_REGS
void SCIInterrupt ( void )
{
char Ch ;
if ( SCISR & ( 1 << RDRF ) )
RemoteCommand = SCIDR ;
/* Receive interrupt: clear request,
keep character */
if ( SCICR2 & ( 1 << TIE ) )
/* If transmit interrupts enabled, */
if ( SCISR & ( 1 << TDRE ) )
{
Ch = TransmitBuffer[+] ;
/* Transmit interrupt:
get next character */
if ( Ch == '\n' )
SCICR2 &= ~( 1 << TIE )
;
/* End of line: disable
transmit interrupt */
SCIDR = Ch ;
/* Send character */
}
}