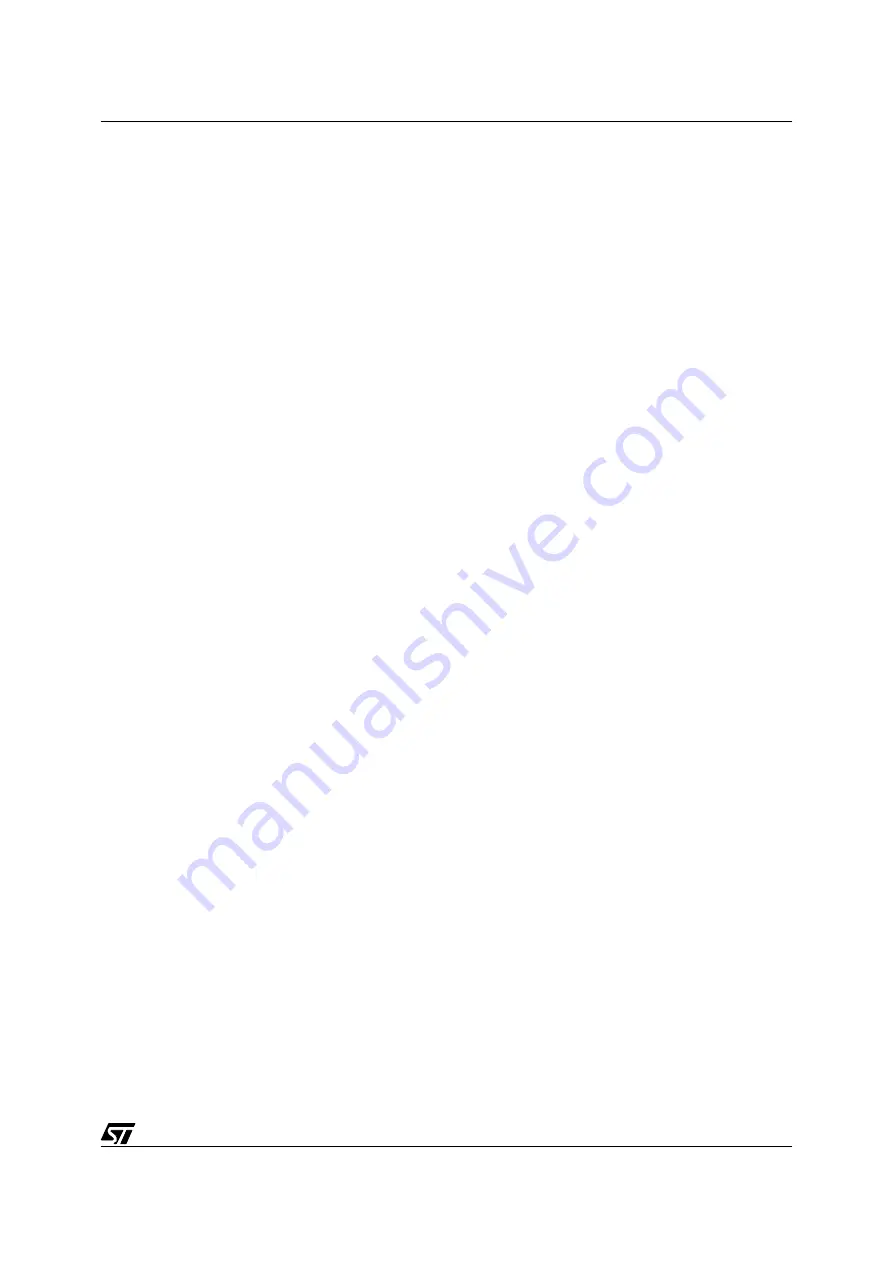
139/317
6 - STMicroelectronics Programming Tools
the position of the data in the memory by hand, and this is susceptible to mistakes; in addition,
inserting an extra variable in the middle of a list implies recalculating the addresses of all those
that follow it.
On the other hand, the absolute position of a variable in memory is virtually irrelevant, pro-
vided it is positioned within the addressable space. For the ST7, there is a little subtlety with
this point. There are addressing modes called Short, or variants thereof, as explained in the
chapter about the instruction set. These modes can only reach variables within the range 0 to
0FFh in memory, called page zero; in exchange, these addressing modes provide faster exe-
cution of the instruction. This means you can optimize your code by allocating the most fre-
quently-used variables to page zero. Coming back to the question of the absolute address of
a variable, we can say that a variable must be defined as either in page zero, or anywhere.
Once this is defined, the absolute position of the variable in memory is of little importance.
Allocating variables in memory is very cumbersome. Luckily, the assembler performs this
task, provided that the programmer specifies the length of each variable and the address of
the first one of the list. This is done using pseudo-ops, that are not machine instructions but
commands that the assembler interprets for specific results.
Syntax: the data storage for variables is specified with the pseudo-ops
DS.B
,
DS.W
and
DS.L
that reserve a byte, a word or a double word of data storage respectively, the address of the
first byte in all cases being associated with the name in the label field. An optional operand
field may exist, that indicates the number of objects of the specified type to be created from the
address associated with the label. Thus,
DS.B 2
is equivalent to
DS.W
and
DS.W 2
is equivalent
to
DS.L
.
For example:
aByte:
DS.B 1
; a one-byte variable
aWord:
DS.W 1
; a one-word variable
Array1:
DS.B 20
; an array of 20 one-byte variables
Array2:
DS.W 40
; an array of 40 one-word variables
here, it is easy to perform any change like insertion, deletion, change in the size of one vari-
able, and still be sure that the addresses are correctly calculated. If the start address is
50h
for
example, the address of
aByte
is
50h
,
aWord
is
51h
,
Array1
is
52h
and
Array2
is
72h
.
These identifiers are now worth their addresses; they can be used in lieu of numeric ad-
dresses in the instructions, like:
LD A,aByte
; aByte = 50h