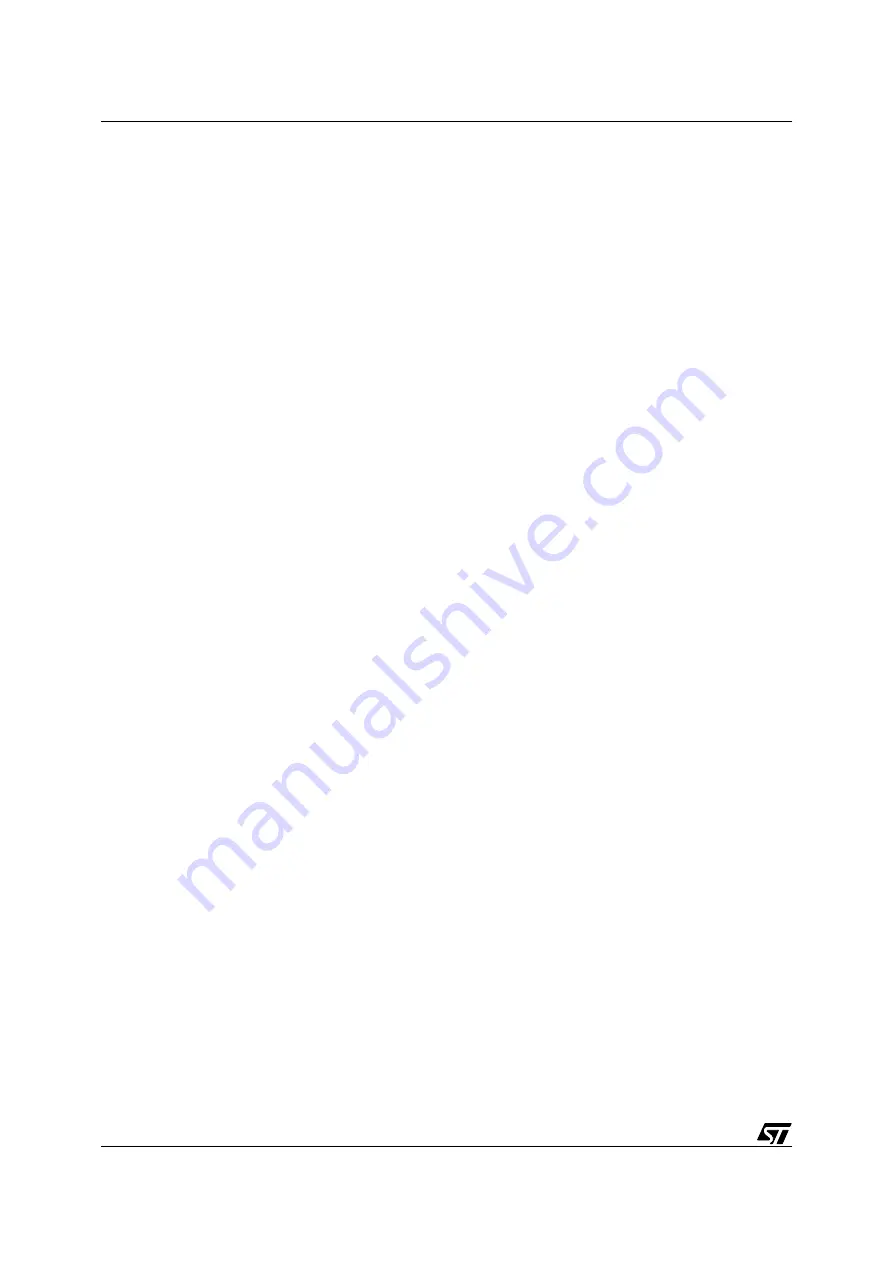
246/317
8 - C Language and the C Compiler
For example, in the project given in Chapter 9, three push-buttons are called CLOSE, OPEN
and TIME. These push-buttons are wired so that pressing one grounds the corresponding pin,
that is otherwise kept high by a pull-up resistor. The following macro yields a zero when no
button is pressed:
#define PUSH_BUTTONS ( ( ~PBDR ) & 0xE0 )
/* upper three bits */
The following constants give the value of the above expression when one of the buttons is
pressed:
#define CLOSE_BUTTON 0x80
#define OPEN_BUTTON
0x40
#define TIME_BUTTON
0x20
These definitions allow you to write simple expressions that are easy to read, like:
if ( PUSH_BUTTONS )
{
/* code to be executed if any button is pressed */
}
More complex expressions may be written, like:
if ( PUSH_BUTTONS == CLOSE_BUTTON )
{
/* code to be executed if the CLOSE button is pressed */
}
if ( PUSH_BUTTONS == ( CLOSE_BUTTON | OPEN_BUTTON ) )
{
/* code to be executed if CLOSE button or OPEN button is pressed */
}
Such expressions both make the code easier to read, and improve the ability of the program
to be changed, when the hardware is modified.
8.8.4 Optimizing resource usage
This chapter has shown that thanks to its intrinsic qualities, C language is suitable for virtually
any microcontroller, since it is a high-level language that still leaves access to the in-depth ma-
chine-specific objects, at least using in-line assembly statements.