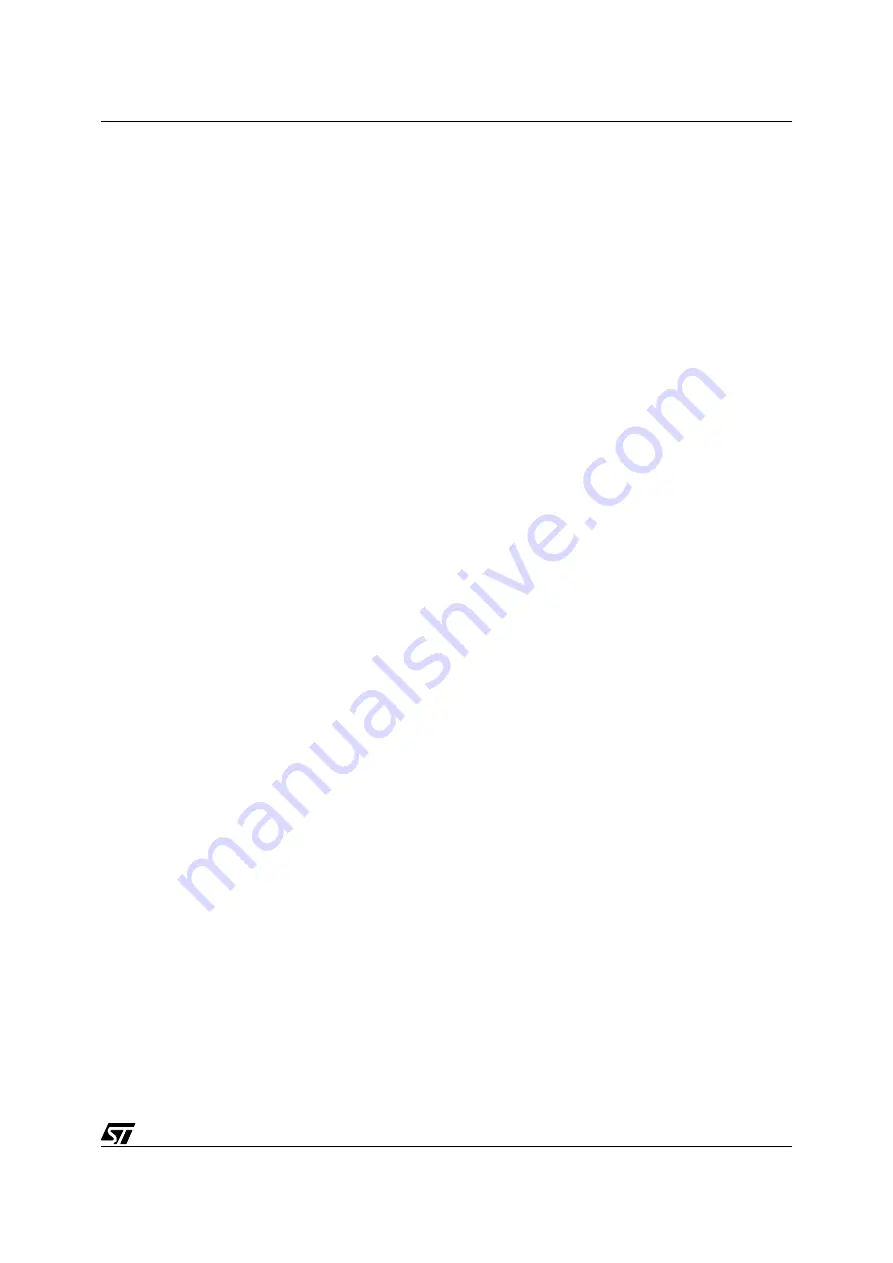
233/317
8 - C Language and the C Compiler
rameters, and allocates local variables to RAM just like global variables. This works the same
way as in a typical implementation, but with the following restrictions:
Functions are neither reentrant nor can they be used recursively.
The compiler uses temporary variables in memory at fixed addresses and thus are not
reentrant. If an interrupt service routine is written in C, these variables must be preserved.
This means the
pragma SAVE_REGS
must be added to
TRAP_PROC
. It pushes these variables
on the stack on entry and restores them on exit:
#pragma TRAP_PROC SAVE_REGS
Long and floating expressions use additional temporary variables in memory. They must
also be saved if long or floating arithmetic is used within the interrupt service function. Four
public functions are available:
SaveLong
and
RestoreLong
, for long arithmetic; and
SaveFloat
and
RestoreFloat
, for
floating arithmetic. The first procedure of each pair must be called at the beginning of the
interrupt service function; the second at the end. Either or both of these pairs must be used
depending on the need. However, there is no stack to save the variables. It is not possible
to nest two levels of interrupt that use long or floating arithmetic.
Other, less important restrictions also apply that are detailed in the compiler manual.
8.4 USING THE ASSEMBLER
There are two ways of using assembly language: using in-line assembler statements and
using the Hiware assembler.
8.4.1 Using In-line assembler statements within a C source text
In-line assembler statements are a very convenient feature of the Hiware C compiler. Since
assembler is the best way of totally controlling the processor, actions that are closely related
to the hardware and the specifics of the core are more easily handled in assembler.
To add assembler lines to a C source text, there are two methods.
8.4.1.1 Single-statement assembler block
If only one statement is used, this syntax can be used:
asm <statement> ;
examples of this are the macros that are predefined in the supplied header file
hidef.h
, and
that allow you to enable or disable interrupts: