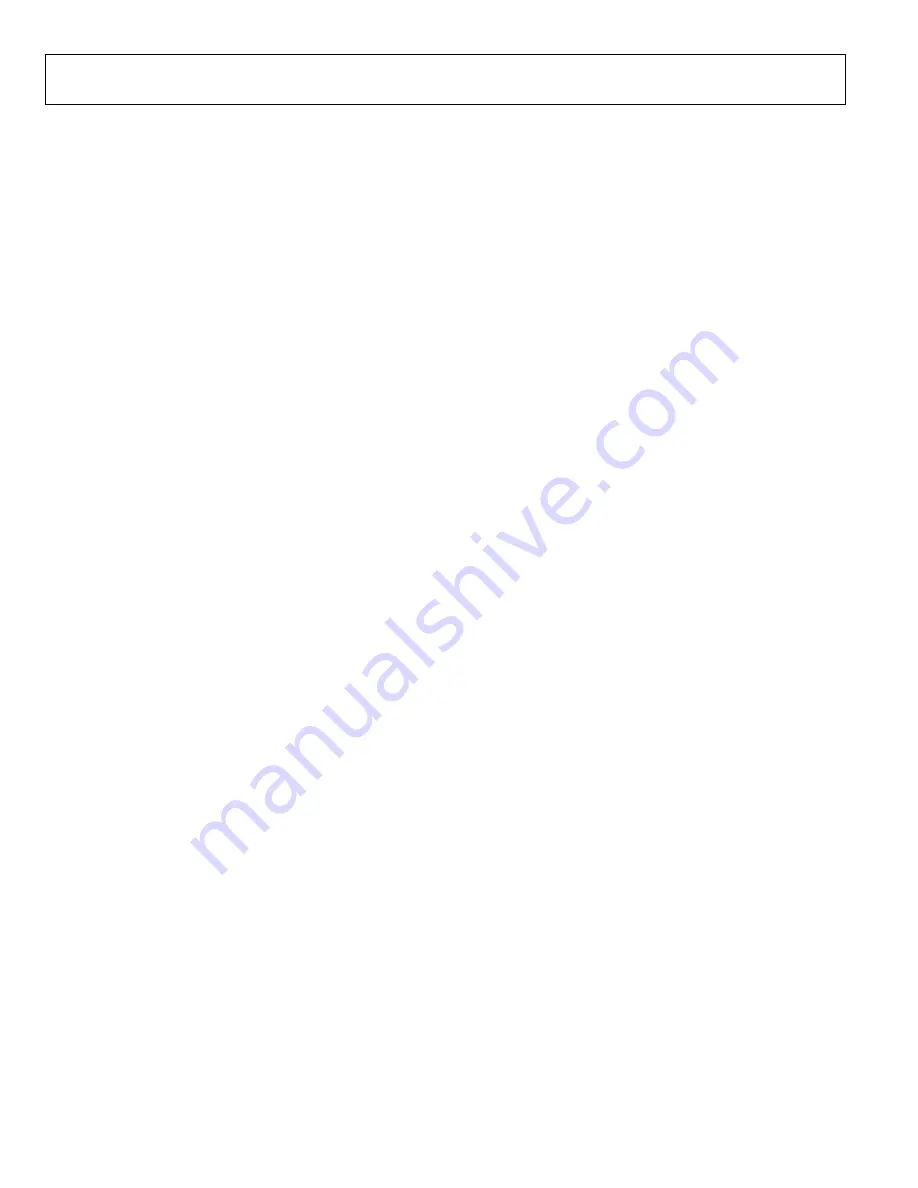
UG-549
ADuCM310 Hardware Reference Manual
Rev. C | Page 86 of 192
Flash DMA Support
Flash controller operations can be supported by DMA. This feature is software configurable. The two flash blocks are independent, meaning
that the user can continue executing from one block while programming another block. The DMA is very useful for this because the core
must only initiate the write to flash and the DMA takes care of it in the background, triggering an interrupt when the operation is
complete. The following code can be used for writing to flash using the DMA.
void FLASHDMAINIT(void)
{
pADI_DMA->DMACFG = 0x1;
// Enable DMA mode in DMA controller
Dma_Init();
NVIC_EnableIRQ(DMA_FLASH_IRQn); // Enable Flash DMA IRQ
FLASHDMAWRITE(uxFlashData, 64);
pADI_DMA->DMAENSET = 0x2000;
pADI_FEE->FEEFLADR = uiAdr;
pADI_FEE->FEEKEY = 0xF123F456;
pADI_FEE->FEECON1 |= (FEECON1_KHDMA_EN); // Enable Flash DMA mode
}
void FLASHDMAWRITE (unsigned char * pucTX_DMA, unsigned int iNumVals)
{
DmaDesc Desc;
// Common configuration of all the descriptors used here
Desc.ctrlCfg.Bits.cycle_ctrl = DMA_BASIC;
desc.ctrlcfg.bits.next_useburst = 0x0;
desc.ctrlcfg.bits.r_power = 1;
desc.ctrlcfg.bits.src_prot_ctrl = 0x0;
Desc.ctrlCfg.Bits.dst_prot_ctrl = 0x0;
Desc.ctrlCfg.Bits.src_size = DMA_SIZE_WORD;
Desc.ctrlCfg.Bits.dst_size = DMA_SIZE_WORD;
// TX Primary Descriptor
Desc.srcEndPtr = (unsigned int)(pu 4*(iNumVals - 0x1) );
Desc.destEndPtr = (unsigned int)&(pADI_FEE->FEEFLDATA1);
Desc.ctrlCfg.Bits.n_minus_1 = iNumVals - 0x1;
Desc.ctrlCfg.Bits.src_inc = DMA_SRCINC_WORD;
Desc.ctrlCfg.Bits.dst_inc = DMA_DSTINC_NO;
*Dma_GetDescriptor(Flash_C) = Desc;
}
void DMA_Flsh_Int_Handler()
{
pADI_FEE->FEEKEY = 0xF123F456;
pADI_FEE->FEECON1 &= (~FEECON1_KHDMA_EN); // Disable Flash DMA mode
dma_done = 1;
}