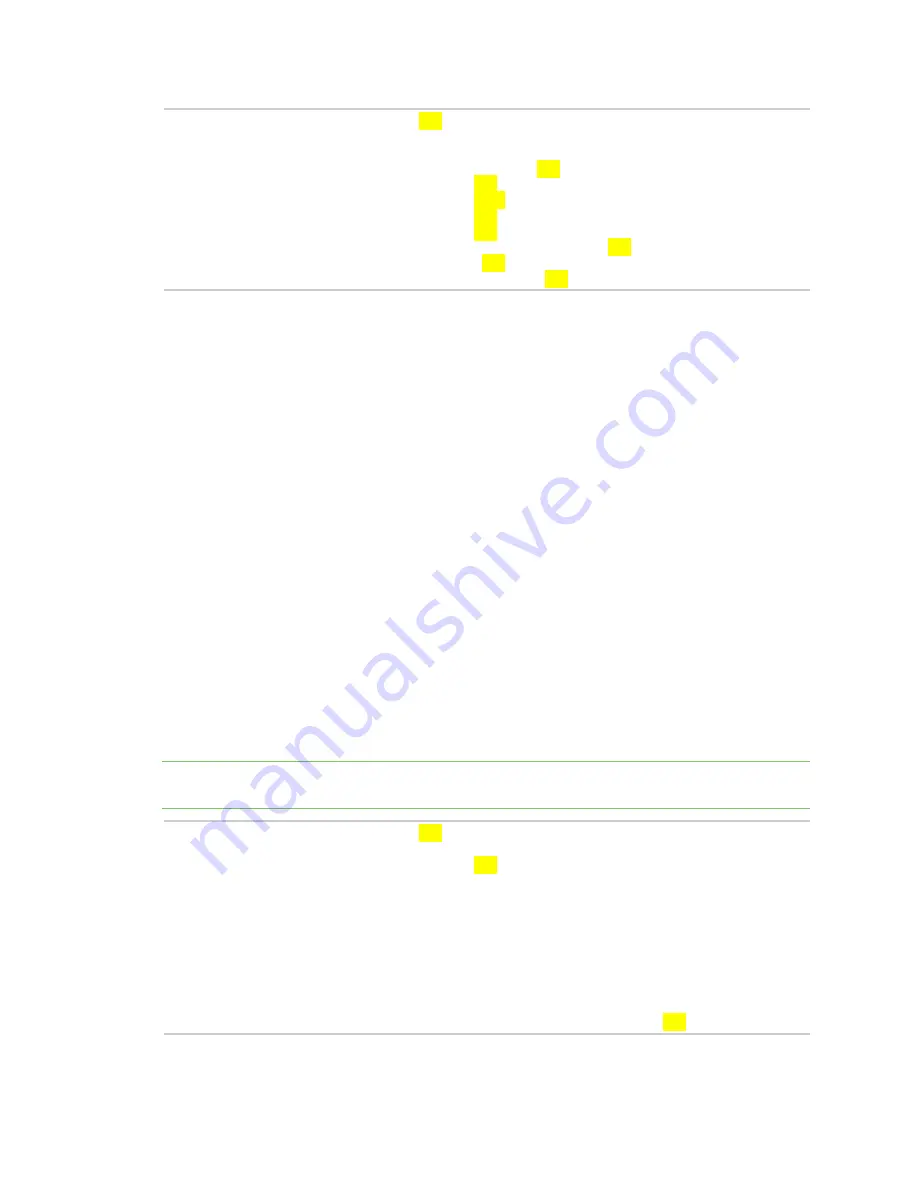
Program XBee Industrial Gateway using Python
Sample programs
Digi XBee Industrial Gateway
57
import watchdog
(1)
import time
w=watchdog.Watchdog('test',20)
(2)
for x in xrange(1,6):
(3)
print "Step ", x
(4)
time.sleep(10.0)
(5)
w.heartbeat()
(6)
print "Step just before the end..."
(7)
time.sleep(60.0)
(8)
print "Step after the end."
(9)
Program notes
1. The watchdog module includes the Watchdog class needed by the program.
2. Create a watchdog object named “test” that will expire in 20 seconds.
3. Loop five times (1-5).
4. Indicate our iteration...
5. ... sleeping less than the timeout on each iteration, but more time than the timeout in total.
6. Reset the watchdog timer to 20 seconds each iteration, allowing all of the loops to complete.
7. Indicate that small loops are complete.
8. Sleep for an interval much longer than the timeout.
9. This print statement never executes, because the system will reboot when the watchdog
timeout expires.
RCI callback
An RCI callback involves two types of actions, demonstrated in the following programs:
n
Making RCI requests from Python applications.
n
Extending RCI to allow Remote Manager to make requests of Python applications. This is
known as an RCI callback.
The following example shows an RCI request.
Note
The highlighted numbers in the sample code correspond to the items in the Program Notes,
below.
import rci
(1)
request_string="""
(2)
<rci_request version="1.1">
<query_state>
<interface_info name="eth0">
<ip/>
</interface_info>
</query_state>
</rci_request>
"""
print rci.process_request(request_string)
(3)
Program notes