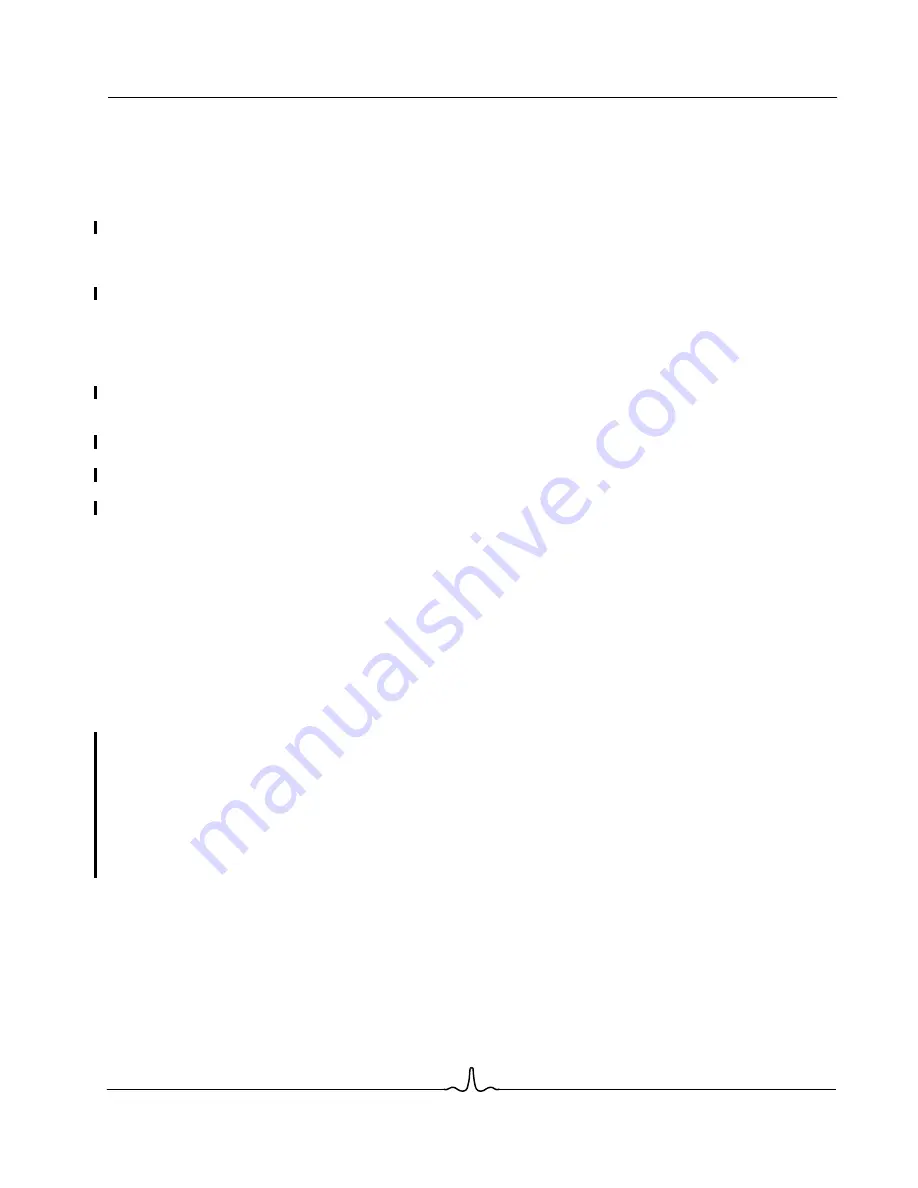
User Manual
BCM1250/BCM1125/BCM1125H
10/21/02
B r o a d c o m C o r p o r a t i o n
Document
1250_1125-UM100CB-R
Section 9: Ethernet MACs Page
279
The value for the hash filter for a particular Ethernet address may be computed using the mchash macro and
standard CRC generator given in the following code:
/********************************************************************
* eth_hwcrc32(buf,len)
*
* Calculate a CRC-32 of the specified bytes. This is used to
* generate the masks for the hash filter on the receive side.
* For the hash filter the bits must be swapped to match the hardware
*
* Input parameters:
* buf - buffer
* len - length of buffer
* Return value:
* CRC as held in MAC hardware
*********************************************************************/
#define mchash(mca) ((eth_hwcrc32(mca, ENET_ADDR_LEN) >> 1) & 0x1FF)
static unsigned eth_hwcrc32(unsigned char *databuf,int datalen)
{
unsigned int idx, crc = 0xFFFFFFFFUL, tmp;
static unsigned int crctab[] = {
0x00000000, 0x1db71064, 0x3b6e20c8, 0x26d930ac,
0x76dc4190, 0x6b6b51f4, 0x4db26158, 0x5005713c,
0xedb88320, 0xf00f9344, 0xd6d6a3e8, 0xcb61b38c,
0x9b64c2b0, 0x86d3d2d4, 0xa00ae278, 0xbdbdf21c
};
for (idx = 0; idx < datalen; idx++) {
crc ^= *+;
crc = (crc >> 4) ^ crctab[crc & 0xf];
crc = (crc >> 4) ^ crctab[crc & 0xf];
}
/*********************************************
** Flip [31:0] to [0:31] to match hardware **
*********************************************/
tmp = 0;
for (idx=0; idx<32;idx++)
tmp |= ((crc<<idx)>>31)<<idx;
return tmp;
}