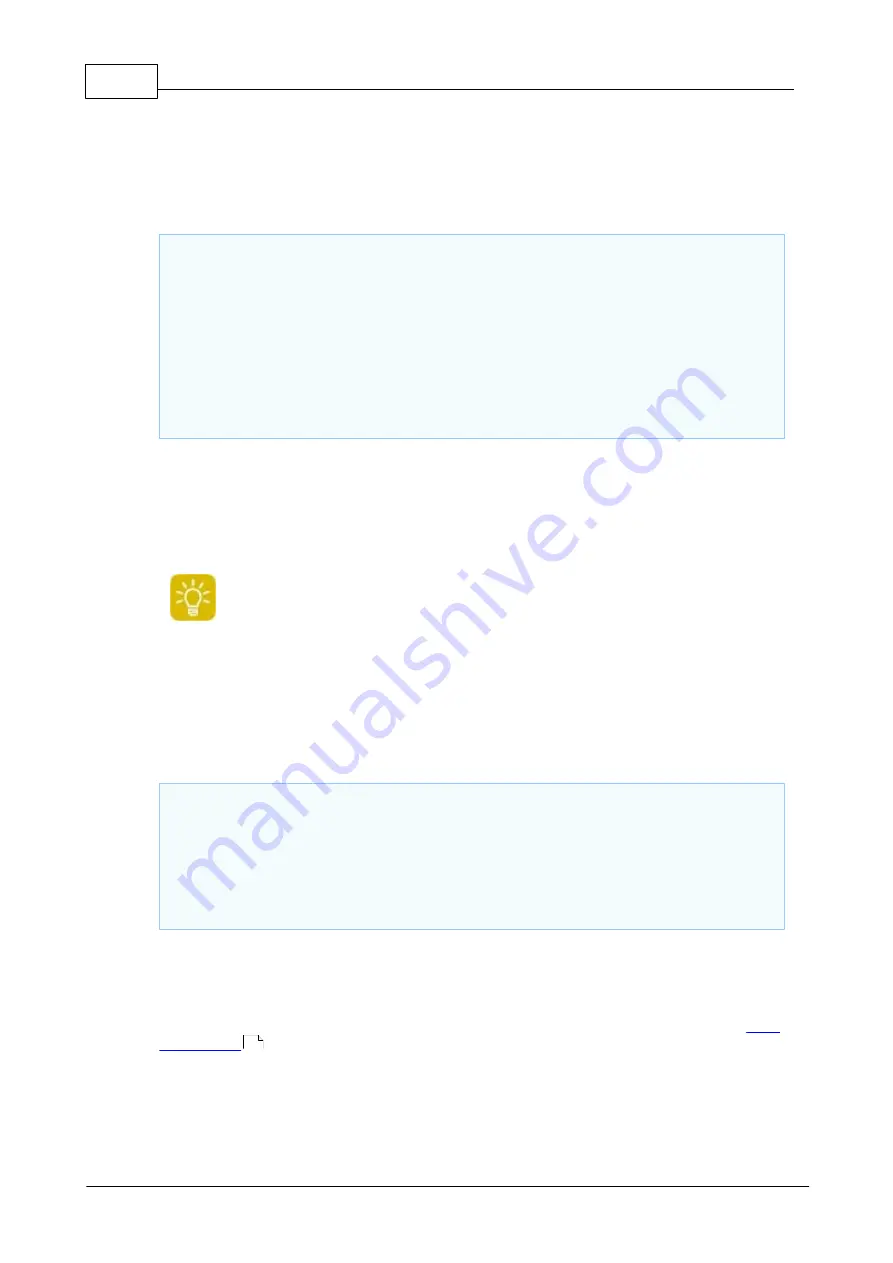
65
TIDE and Tibbo BASIC User Manual
©2000-2008 Tibbo Technology Inc.
accessible. Another example would be when processing large strings -- copying
them would cause significant overhead.
In such cases, arguments are passed by reference. When passing by reference, the
actual values are not copied. Instead, the procedure receives a reference to the
location of the original values in memory -- hence, the name. For example:
sub
foo(
byref
x
as
byte
)
...
x = 1
...
end
sub
sub bar
dim y as byte
y = 2
foo(y)
' at this point in code, y is 1!
end sub
When passing arguments by reference, the code within the procedure will access
these arguments using indirect addressing. This may cause possible overhead. The
only case where it does not cause overhead (relative to passing by value) is when
working with large strings; in this case, passing them by reference saves the need
to copy the whole string to another location in memory.
Here is our advice: when dealing with strings it is usually better (in
terms of performance) to pass them by reference. For all other types,
passing by value yields better performance.
Strict byref argument match is now required!
Beginning with Tibbo Basic release
2.0
, strict match is required between the type
of byref argument and the type of variable being passed. For example, trying to
pass a string for a byte will now cause a compiler error:
sub
bar(
byref
x
as
byte
)
...
end
sub
sub
foo
bar("123")
'attempt to pass a string will generate a compiler error
bar(val("123"))
'this will work!
end
sub
In the above example, sub bar takes a byte argument and we are trying to pass a
string. Wrong! Compiler understands that byref arguments can be manipulated by
the procedure that takes them. Therefore, it is important that actual variables
being passed match the type of argument that procedure expects. Automatic
won't apply here.
45