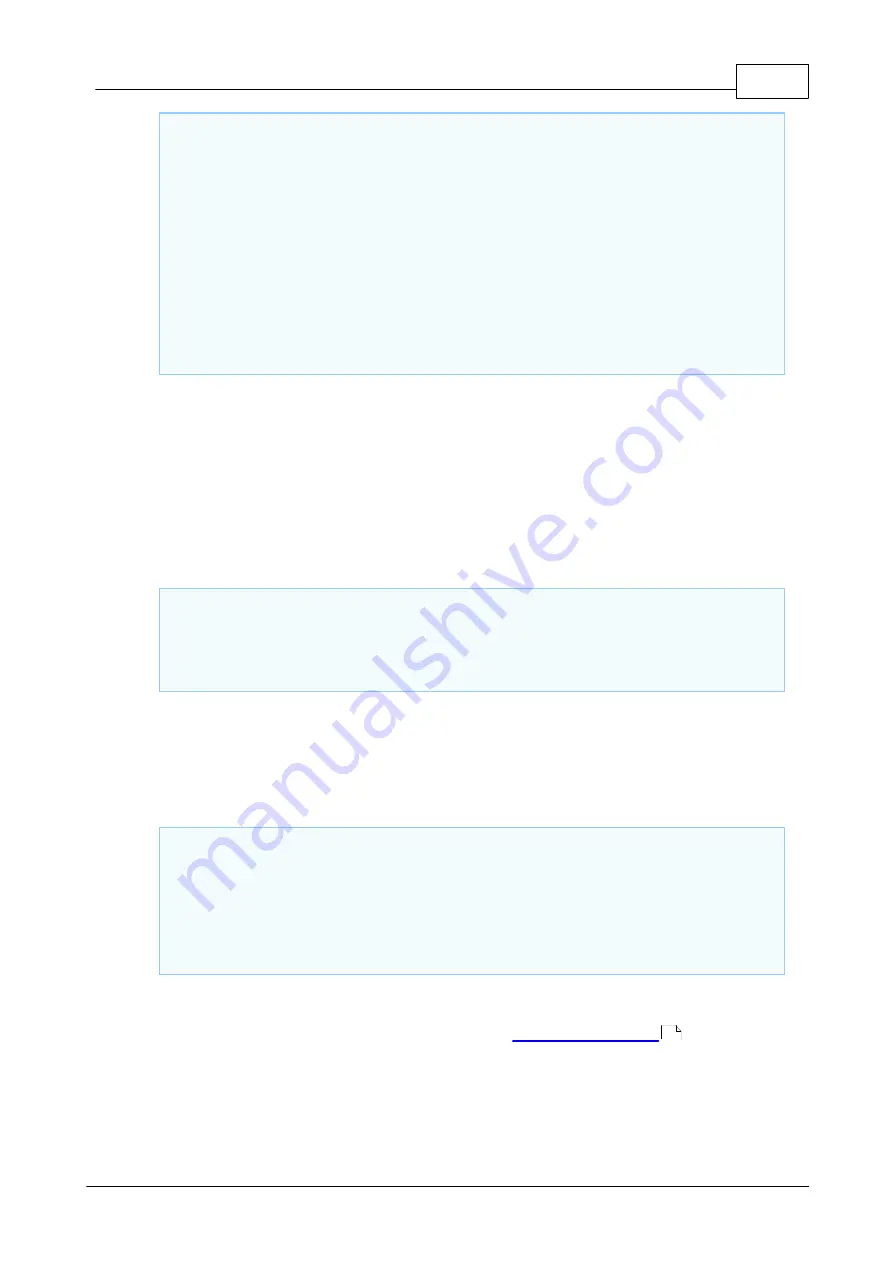
304
Platforms
©2000-2008 Tibbo Technology Inc.
dim
s
as
string
...
l1:
while
sock.rxlen =
0
doevents
'good practice: let other events execute while we are
waiting
wend
sock.nextpacket
'Now we know that we do have data, 'move to' the next UDP
datagram. This is like entering the on_sock_data_arrival.
s = sock.getdata(
255
)
'get data
goto
l1
...
Sending TCP and UDP Data
In the previous sections, we have explained how to handle an incoming stream of
data. You could say it was incoming-data driven. Sometimes you need just the
opposite -- you need to perform operations based on the sending of data.
Sending data with on_sock_data_sent event
Supposing that in a certain system, you need to send out a long string of data
when a button is pressed. A simple code for this would look like this:
sub
on_button_pressed
sock.setdata(
"This is a long string waiting to be sent. Send me
already!"
)
sock.send
end
sub
The code above would work, but only if at the moment of code execution the
necessary amount of free space was available in the TX buffer (otherwise the data
would get truncated). So, obviously, you need to make sure that the TX buffer has
the necessary amount of free space before sending. A simple polling solution would
look like this:
sub
on_button_pressed
dim
s
as
string
s =
"This is a long string waiting to be sent. Send me already!"
while
sock.txfree < len(s)
wend
sock.setdata(s)
sock.send
end
sub
Again, this is not so good, as it would block other event handlers. So, instead of
doing that, we would employ a code that uses
:
342