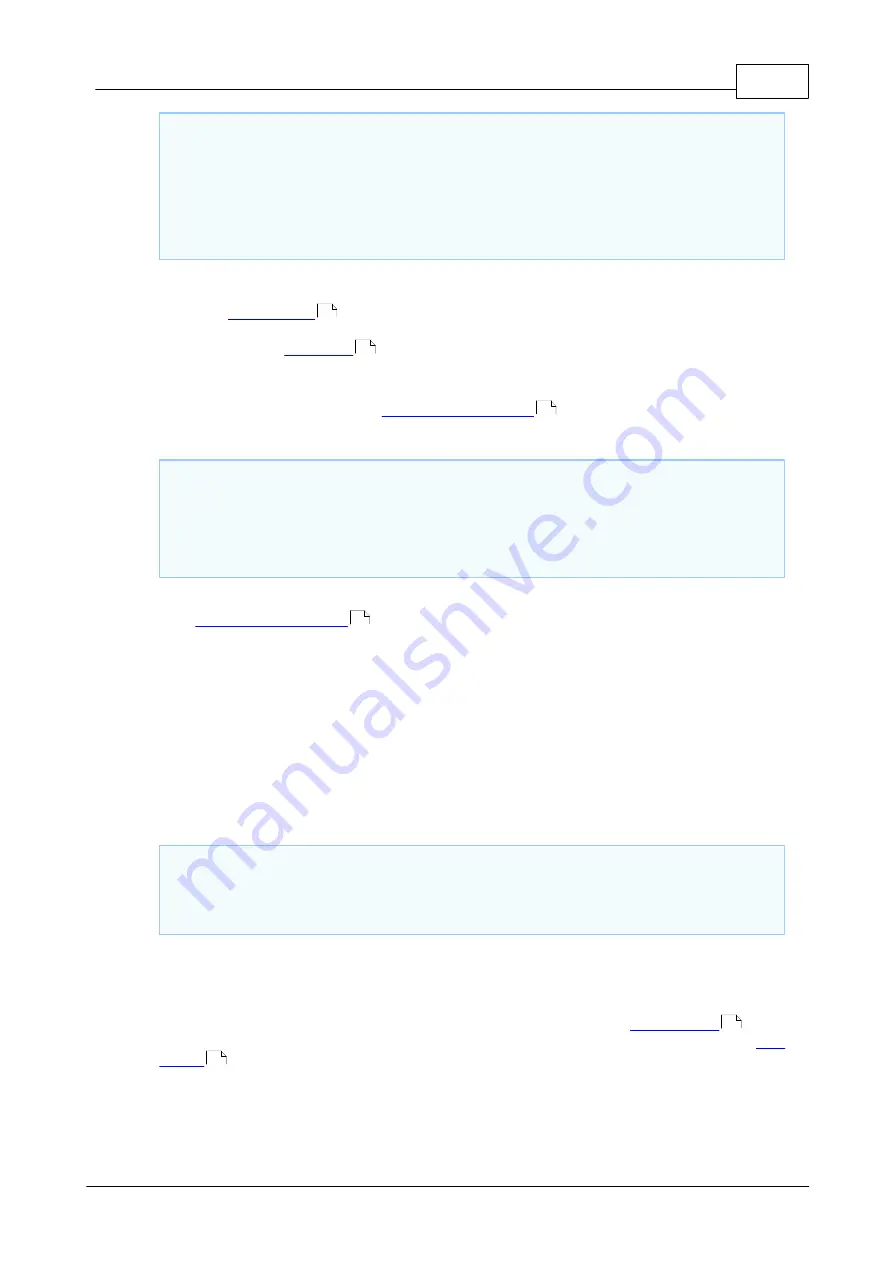
242
Platforms
©2000-2008 Tibbo Technology Inc.
sub
on_sys_init
while
ser.rxlen =
0
wend
' basically keeps executing again and again as long as ser.rxlen = 0
s = ser.getdata(
255
)
' once loop is exited, it means data has arrived. We
extract it.
end
sub
This approach will work, but it will forever keep you in a specific event handler
(such as
) and other events will never get a chance to execute. This
is an example of blocking code which could cause a system to freeze. Of course,
you can use the
statement, but generally we recommend you to avoid
this approach.
Since our platform is event-driven, you should use events to tell you when new
data is available. There is an
event which is generated
whenever there is data in the rx buffer:
sub
on_ser_data_arrival
dim
s
as
string
s = ser.getdata(
255
)
' Extract the data -- but in a non-blocking way.
' .... code to process data ....
end
sub
This
event is generated whenever there is data in the RX
buffer, but only once. There are never two on_ser_data_arrival events waiting in
the queue. The next event is only generated after the previous one has completed
processing, if and when there is any data available in the RX buffer.
This means that when handling this event, you don't have to get all the data in the
RX buffer. You can simply handle a chunk of data and once you leave the event
handler, a new event of the same type will be generated if there is still
unprocessed data left.
Here is a correct example of handling arriving serial data through the
on_ser_data_arrival event. This example implements a data loopback -- whatever
is received by the serial port is immediately sent back out.
sub
on_ser_data_arrival
ser.setdata(ser.getdata(ser.txfree))
ser.send
end
sub
We want to handle this loopback as efficiently as possible, but we must not
overrun the TX buffer. Therefore, we cannot simply copy all arriving data from the
RX buffer into the TX buffer. We need to check how much free space is available in
the TX buffer. The first line of this code implements just that:
method takes as much data from the RX buffer as possible, but not more than
(the available room in the TX buffer). The second line just sends the
data.
220
82
258
258
253
266