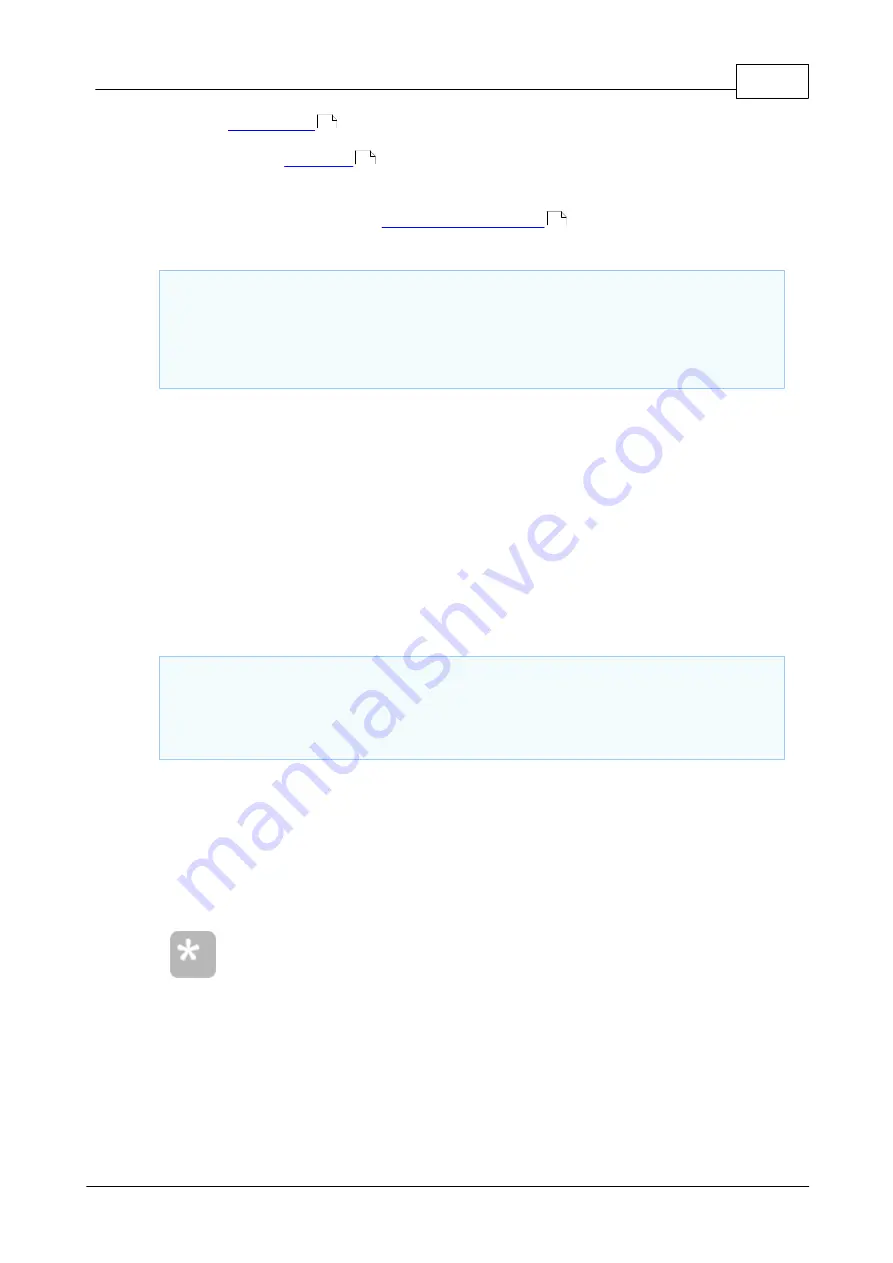
302
Platforms
©2000-2008 Tibbo Technology Inc.
) and other events will never get a chance to execute. This
is an example of blocking code which could cause a system to freeze. Of course,
you can use the
statement, but generally we recommend you to avoid
this blocking approach.
Since our platform is event-driven, you should use events to tell you when new
data is available. There is an
event which is generated
whenever there is data in the RX buffer:
sub
on_sock_data_arrival
dim
s
as
string
s = sock.getdata(
255
)
' Extract the data -- but in a non-blocking way.
' .... code to process data ....
end
sub
The on_sock_data_arrival event is generated whenever there is data in the RX
buffer, but only once. There are never two on_sock_data_arrival events (for the
same socket) waiting in the queue. The next event is only generated after the
previous one has completed processing, if and when there is any data available in
the RX buffer.
This means that when handling this event, you don't have to get all the data in the
RX buffer. You can simply handle a chunk of data and once you leave the event
handler, a new event of the same type will be generated.
Here is a correct example of handling arriving socket data through the
on_sock_data_arrival event. This example implements a data loopback -- whatever
is received by the socket is immediately sent back out.
dim rx_tcp_packet_len as word 'to keep the size of the next incoming TCP
packet
sub on_sys_init
end sub
We want to handle this loopback as efficiently as possible, but we must not
overrun the TX buffer. Therefore, we cannot simply copy all arriving data from the
RX buffer into the TX buffer. We need to check how much free space is available in
the TX buffer. The first line of this code implements just that: sock.getdata takes
as much data from the RX buffer as possible, but not more than sock.txfree (the
available room in the TX buffer). The second line just sends the data.
Actually, this call will handle no more than 255 bytes in one pass. Even
though we seemingly copy the data directly from the RX buffer to the
TX buffer, this is done via a temporary string variable automatically
created for this purpose. In this platform, string variables cannot
exceed 255 bytes.
220
82
342