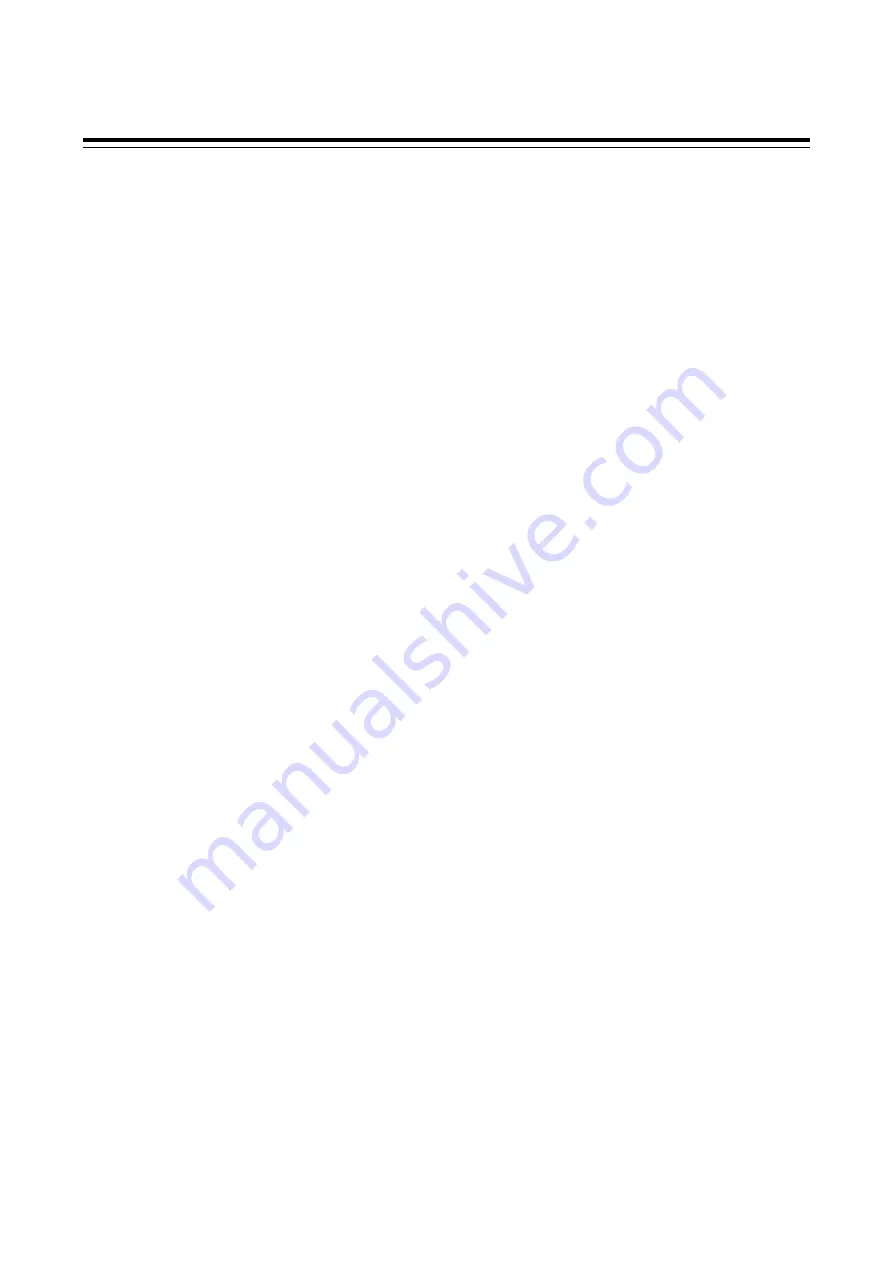
7. MODBUS
IMS01J02-E2
114
Example of a CRC calculation in the ‘C’ language
This routine assumes that the data types ‘uint16’ and ‘uint8’ exists. Theses are unsigned 16-bit integer
(usually an ‘unsigned short int’ for most compiler types) and unsigned 8-bit integer (unsigned char).
‘z_p’ is a pointer to a Modbus message, and z_messaage_length is its length, excluding the CRC. Note
that the Modbus message will probably contain NULL characters and so normal C string handling
techniques will not work.
uint16 calculate_crc (byte *z_p, unit16 z_message_length)
/* CRC runs cyclic Redundancy Check Algorithm on input z_p */
/* Returns value of 16-bit CRC after completion and
*/
/* always adds 2 crc bytes to message
*/
/* returns 0 if incoming message has correct CRC
*/
{
uint16 CRC= 0xffff;
uint16 next;
uint16 carry;
uint16 n;
uint8 crch, crcl;
while (z_messaage_length--) {
next = (uint16) *z_p;
CRC ^= next;
for (n = 0; n < 8; n++) {
carry = CRC & 1;
CRC >>= 1;
if (carry) {
CRC ^= 0xA001;
}
}
z_p++;
}
crch = CRC / 256;
crcl = CRC % 256
z_p [z_messaage+] = crcl;
z_p [z_messaage_length] = crch;
return CRC;
}