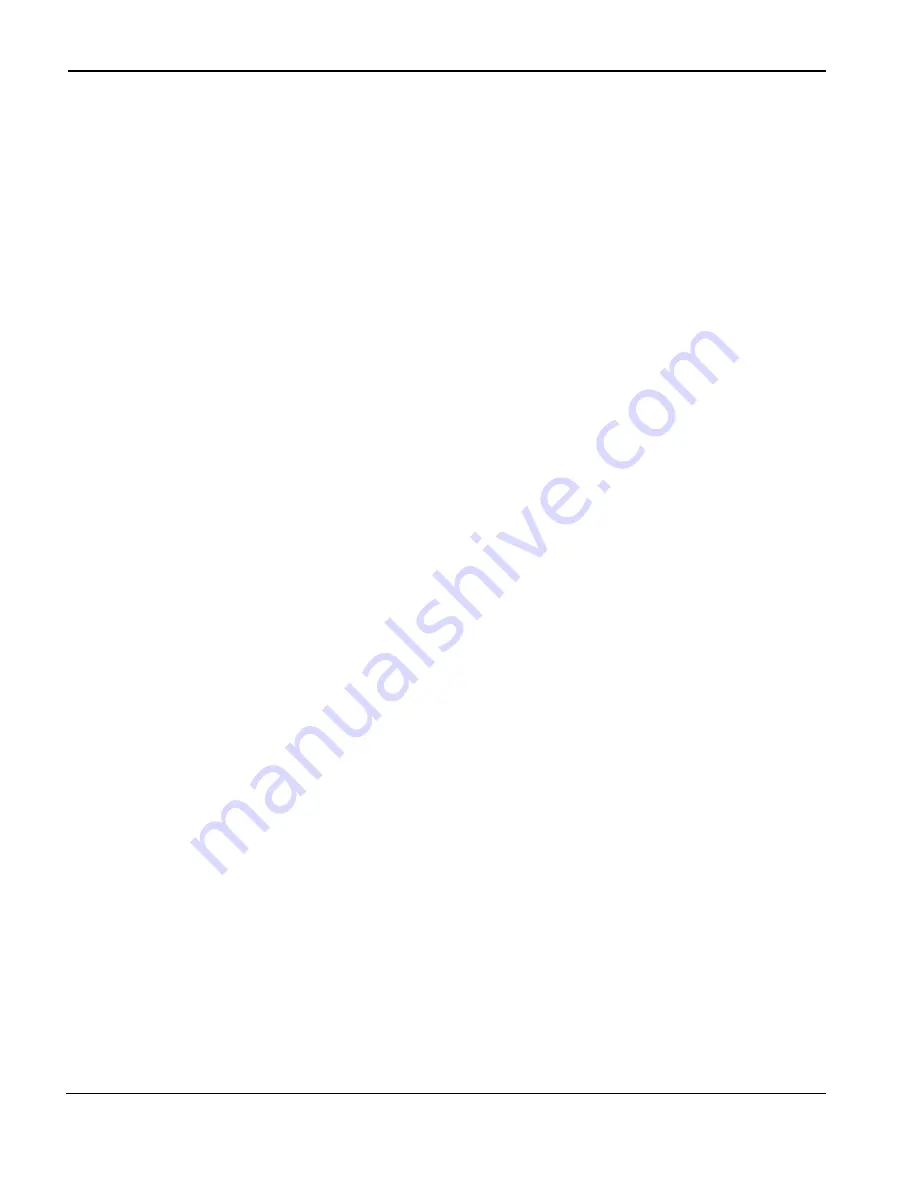
Page 54
Epson Research and Development
Vancouver Design Center
SED1352
Programming Notes and Examples
X16-BG-007-04
Issue Date: 98/10/08
//-------------------------------------------------------------------------
//
// FUNCTION: ShowVerticalBars()
//
// DESCRIPTION: Displays a series of vertical bars, each with a
// different gray shade. For 4 gray levels, each
// vertical bar is 40 pixels wide. For 16 gray levels,
// each vertical bar is 20 pixels wide.
//
// INPUTS: Video address which points to beginning of vertical bars.
// This address must be at the leftmost column of the display.
//
// RETURN VALUE: None.
//
//-------------------------------------------------------------------------
void ShowVerticalBars(unsigned char _far *pVideo)
{
static unsigned int y;
static unsigned int Bar, BarWidth, val;
static unsigned char _far *pVideoStart;
//
// To display vertical bars, this routine assumes that pVideo points
// to the beginning of a scan line. In addition, this routine assumes that
// the Address Pitch Adjustment Register is 0 (no virtual display).
// To write one vertical line, first write one pixel to the first byte
// pointed to by pVideo. Write the next pixel to the byte on the next scan
// line pointed to by BytesPerScanLine (this only works if the
// Address Pitch Adjustment Register is 0). Continue writing pixels by
// going down each scan line.
//
pVideoStart = pVideo;
for (y = 0; y < PanelY; ++y)
{
for (Bar = 0; Bar < PanelGrayLevel; ++Bar)
{
for (BarWidth = 0; BarWidth < 10; ++BarWidth)
{
if (PanelGrayLevel == 4)
{
//
// In the 4 gray level mode, each pixel is stored as two bits.
// Since a byte holds 8 bits, there are 4 pixels per byte.
// The variable "val" represents the pixel value.
//
val = Bar % 4;
*+ = (unsigned char) ((val << 6) | (val << 4) |
(val << 2) | val);
}
else
{