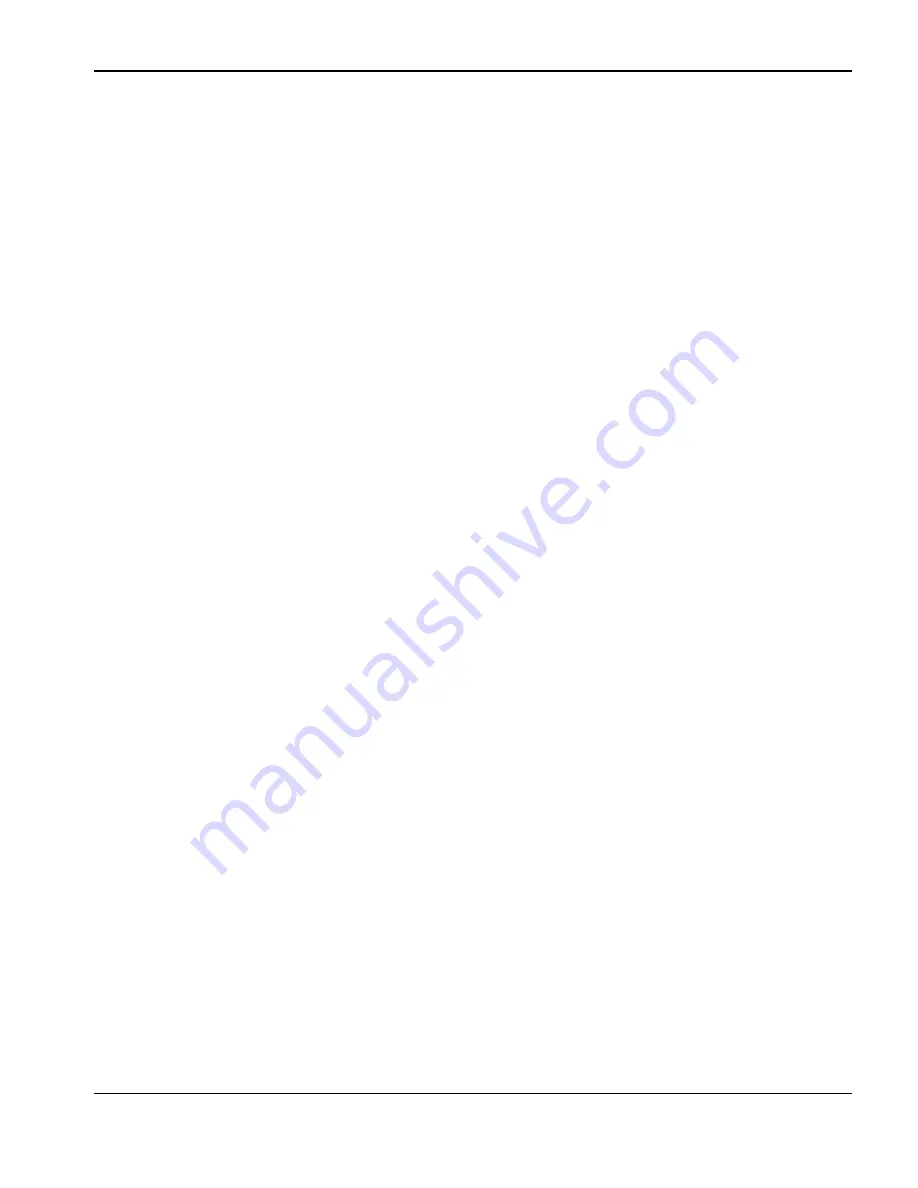
Epson Research and Development
Page 49
Vancouver Design Center
Programming Notes and Examples
SED1352
Issue Date: 98/10/08
X16-BG-007-04
// Bits 0-7 are in AUX[2], Bit 8 is in AUX[3].
//
// Because the Memory Interface is set to 16 bits, the
// Line Byte/Word Count Register counts in words. In addition,
// there are 2 pixels/byte since there are 16 gray levels.
// To calculate the number of words in a scan line, use the following
// formula:
//
// number of pixels per scan line
// -------------------------------- - 1
// (2 pixels/byte) * (2 bytes/word)
//
val = (PanelX / 4) - 1; // For 16 gray shades only
WriteRegister(2, val & 0xff); // Line Byte/Word Count Register
WriteRegister(3, (val >> 8) & 0x01); // Line Byte/Word Count/Power Save Reg
//
// BytesPerScanLine is a global variable
//
BytesPerScanLine = (PanelX / 2); // For 16 gray shades only
//--------------------------------------
//
// Total Display Line Count Register
// Screen 1 Display Line Count Register
//
// To show a full image on Screen 1, copy the Total Display Line Count
// into the Screen 1 Display Line Count.
//
//
// Assume that all panels smaller than 400 lines are in 4 bit mode
//
if (PanelY < 400)
{
val = ReadRegister(1);
val &= ~0x04;
WriteRegister(1, val); // Write to Mode Register; LCD Data Width = 4 bits
}
val = PanelY;
//
// A dual panel LCD will, of course, have two panels. Each panel will
// show either the top or bottom half of the image, which is half of the
// vertical resolution.
//
if (PanelType == TYPE_DUAL)
val /= 2;