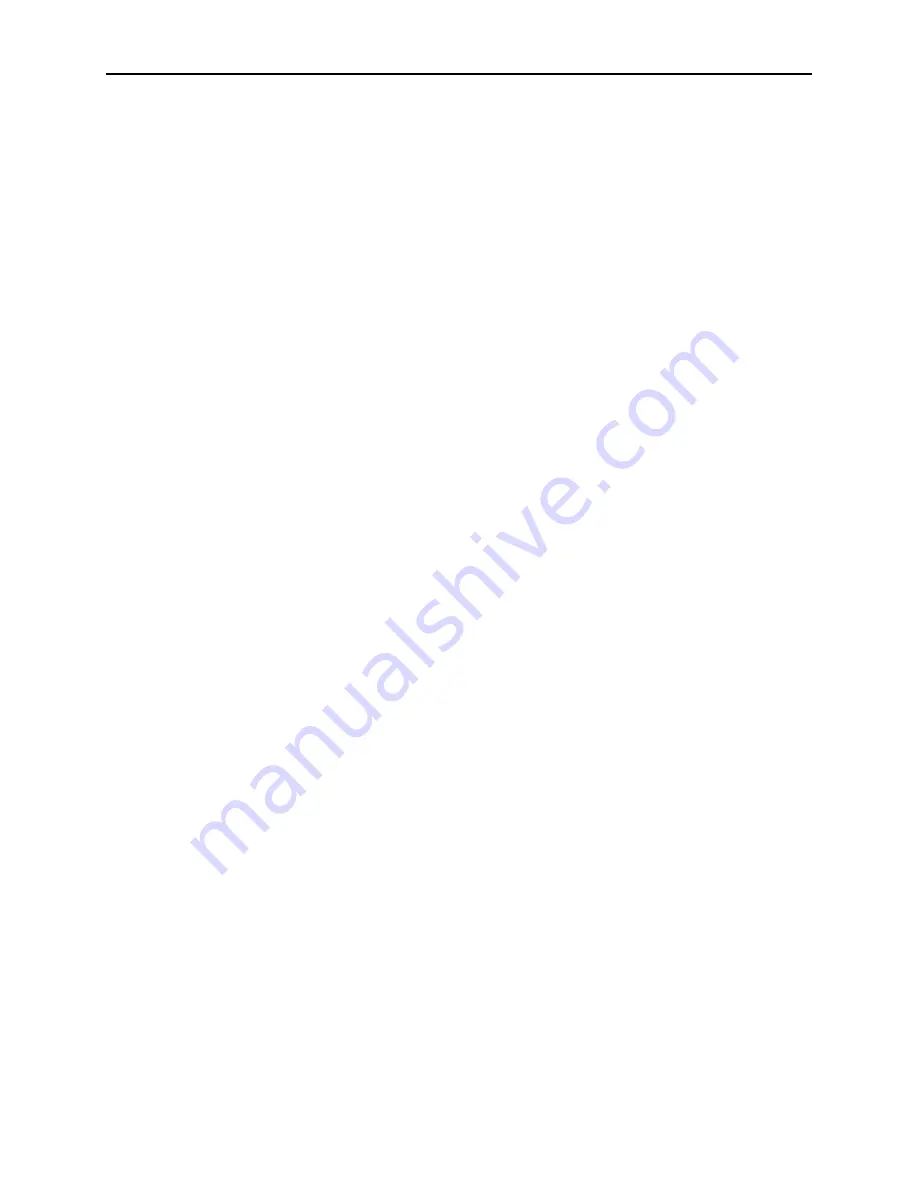
Programming
VL-486-4 Reference Manual
CIO Chip – 103
I
NTERRUPT
C
ODE
E
XAMPLE
The following code example demonstrates the generation of an interrupt when a specific bit
pattern (55h) is detected on Port B. It is assumed that a hardware loopback cable is installed
causing values written to Port A to "come back in" on Port B.
// =======================================================================
//
VersaLogic Test Program for 486-4 I/O to the Z8536 Chip
// =======================================================================
#include <dos.h>
#include <stdio.h>
void main(void)
{
unsigned char in_val1, in_val2; /* temp. values */
/* Configure all Ports A,B, & C */
printf ("\nStart configuration sequence\n");
outportb(0xE7, 0x00);
// Dummy write to Port E7 to clear reset
in_val1 = inportb(0xE7);
printf ("Make Port A bits outputs\n");
outportb(0xE7, 0x23);
// Set all Port A bits to output
outportb(0xE7, 0x00);
outportb(0xE7, 0x23);
// Test if values are written to Z8536
in_val1 = inportb(0xE7);
printf ("Output E7, 23h and E7, 00h; returned value = %x\n", in_val1);
printf ("Make Port B bits inputs\n");
outportb(0xE7, 0x2B);
// Set all Port B bits to input
outportb(0xE7, 0xFF);
outportb(0xE7, 0x2B);
// Test if values are written to Z8536
in_val1 = inportb(0xE7);
printf ("Output E7, 2Bh and E7, FFh; returned value = %x\n", in_val1);
printf ("Enable ports A,B, & C\n");
outportb(0xE7, 0x01);
// Enbale ports A,B, & C
outportb(0xE7, 0x94);
outportb(0xE7, 0x01);
// Test if values are written to Z8536
in_val1 = inportb(0xE7);
printf ("Output E7, 01h and E7, 94h; returned value = %x\n", in_val1);
printf ("End of configuraition... \n");
// End configuration
// Initilize Port B for pattern matching
printf ("Write to Port B Mode register\n");
outportb(0xE7, 0x28);
//
outportb(0xE7, 0x0B);
outportb(0xE7, 0x28);
// Test if values are written to Z8536
in_val1 = inportb(0xE7);
printf ("Output E7, 28h and E7, 0Bh; returned value = %x\n", in_val1);
// Setup pattern to match as 55h, through the next 3 registers
printf ("Write to Port B pattern polarity\n");
outportb(0xE7, 0x2D);
//
outportb(0xE7, 0x55);
outportb(0xE7, 0x2D);
// Test if values are written to Z8536