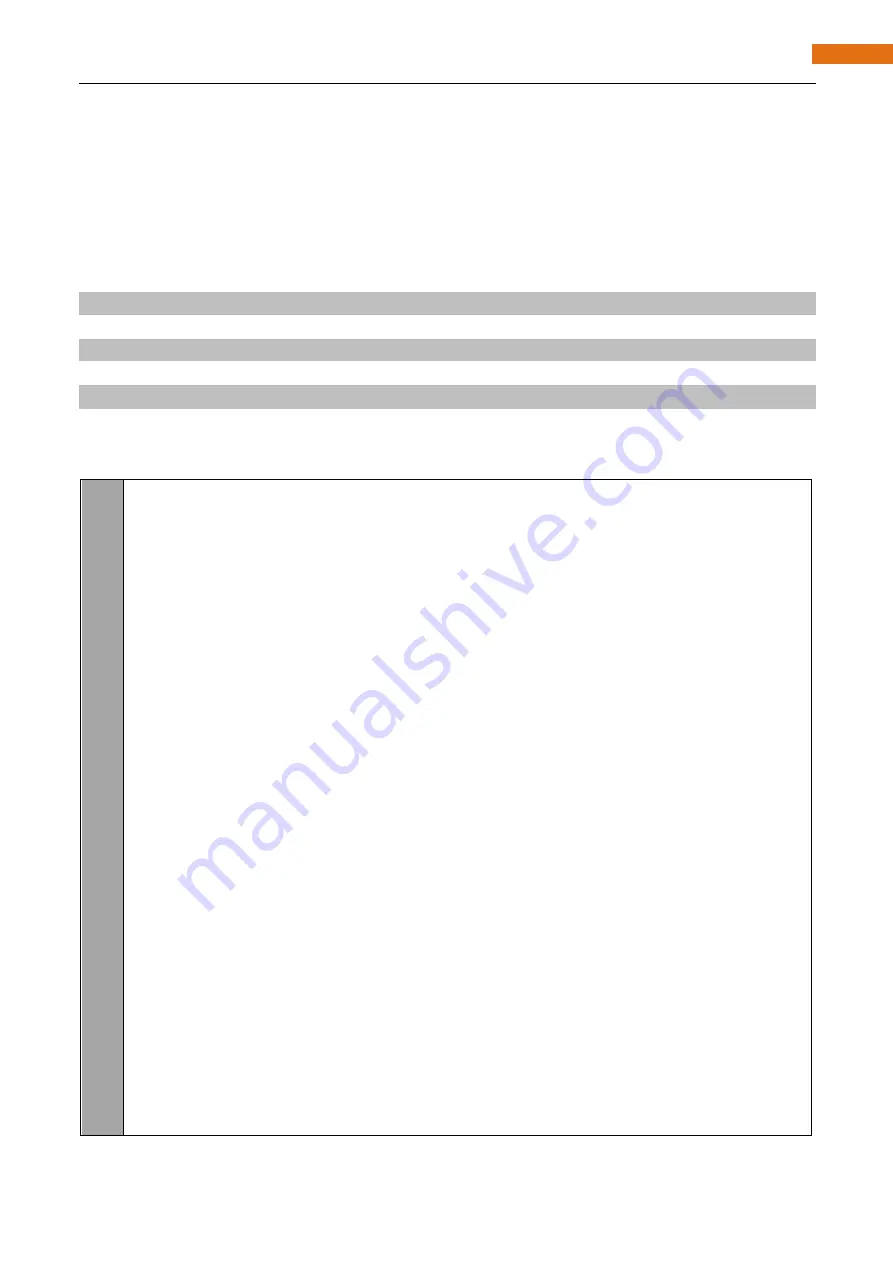
163
Chapter 15 Servo
Code
In this project, we make the servo rotate from 0 degrees to 180 degrees, and then from 180 degrees to 0
degrees.
C Code 15.1.1 Sweep
First observe the project result, and then analyze the code.
1.
Use cd command to enter 15.1.1_Sweep directory of C code.
cd ~/Freenove_Ultimate_Starter_Kit_for_Raspberry_Pi/Code/C_Code/15.1.1_Sweep
2.
Use following command to compile "Sweep.c" and generate executable file "Sweep".
gcc Sweep.c -o Sweep -lwiringPi
3.
Run the generated file "Sweep".
sudo ./Sweep
After the program is executed, the servo will rotate from 0 degrees to 180 degrees, and then from 180 degrees
to 0 degrees, circularly.
The following is the program code:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
#include <wiringPi.h>
#include <softPwm.h>
#include <stdio.h>
#define OFFSET_MS 3
//Define the unit of servo pulse offset: 0.1ms
#define SERVO_MIN_MS 5+OFFSET_MS
//define the pulse duration for minimum angle of
servo
#define SERVO_MAX_MS 25+OFFSET_MS
//define the pulse duration for maximum angle of
servo
#define servoPin 1
//define the GPIO number connected to servo
long
map
(
long
value
,
long
fromLow
,
long
fromHigh
,
long
toLow
,
long
toHigh
){
return
(
toHigh
-
toLow
)*(
value
-
fromLow
)
/
(
fromHigh
-
fromLow
)
+
toLow
;
}
void
servoInit
(
int
pin
){
//initialization function for servo PWM pin
softPwmCreate
(
pin
,
0
,
200
);
}
void
servoWrite
(
int
pin
,
int
angle
){
//Specif a certain rotation angle (0-180) for the
servo
if
(
angle
>
180
)
angle
=
180
;
if
(
angle
<
0
)
angle
=
0
;
softPwmWrite
(
pin
,
map
(
angle
,
0
,
180
,
SERVO_MIN_MS
,
SERVO_MAX_MS
));
}
void
servoWriteMS
(
int
pin
,
int
ms
){
//specific the unit for pulse(5-25ms) with
specific duration output by servo pin: 0.1ms
if
(
ms
>
SERVO_MAX_MS
)
ms
=
SERVO_MAX_MS
;
Содержание Ultimate Starter Kit
Страница 1: ...Free your innovation Freenove is an open source electronics platform www freenove com ...
Страница 116: ...Chapter 9 Potentiometer RGBLED 116 www freenove com support freenove com Circuit Schematic diagram ...
Страница 117: ...117 Chapter 9 Potentiometer RGBLED www freenove com support freenove com Hardware connection ...
Страница 136: ...Chapter 12 Joystick 136 www freenove com support freenove com Circuit Schematic diagram Hardware connection ...
Страница 155: ...155 Chapter 14 Relay Motor www freenove com support freenove com Hardware connection OFF 3 3V ...
Страница 173: ...173 Chapter 16 Stepping Motor www freenove com support freenove com Hardware connection ...
Страница 182: ...Chapter 17 74HC595 LEDBar Graph 182 www freenove com support freenove com Circuit Schematic diagram Hardware connection ...
Страница 197: ...197 Chapter 18 74HC595 7 segment display www freenove com support freenove com Circuit Schematic diagram ...
Страница 198: ...Chapter 18 74HC595 7 segment display 198 www freenove com support freenove com Hardware connection ...
Страница 239: ...239 Chapter 22 Matrix Keypad www freenove com support freenove com Circuit Schematic diagram ...
Страница 240: ...Chapter 22 Matrix Keypad 240 www freenove com support freenove com Hardware connection ...
Страница 270: ...Chapter 26 WebIOPi IOT 270 www freenove com support freenove com Circuit Schematic diagram Hardware connection ...