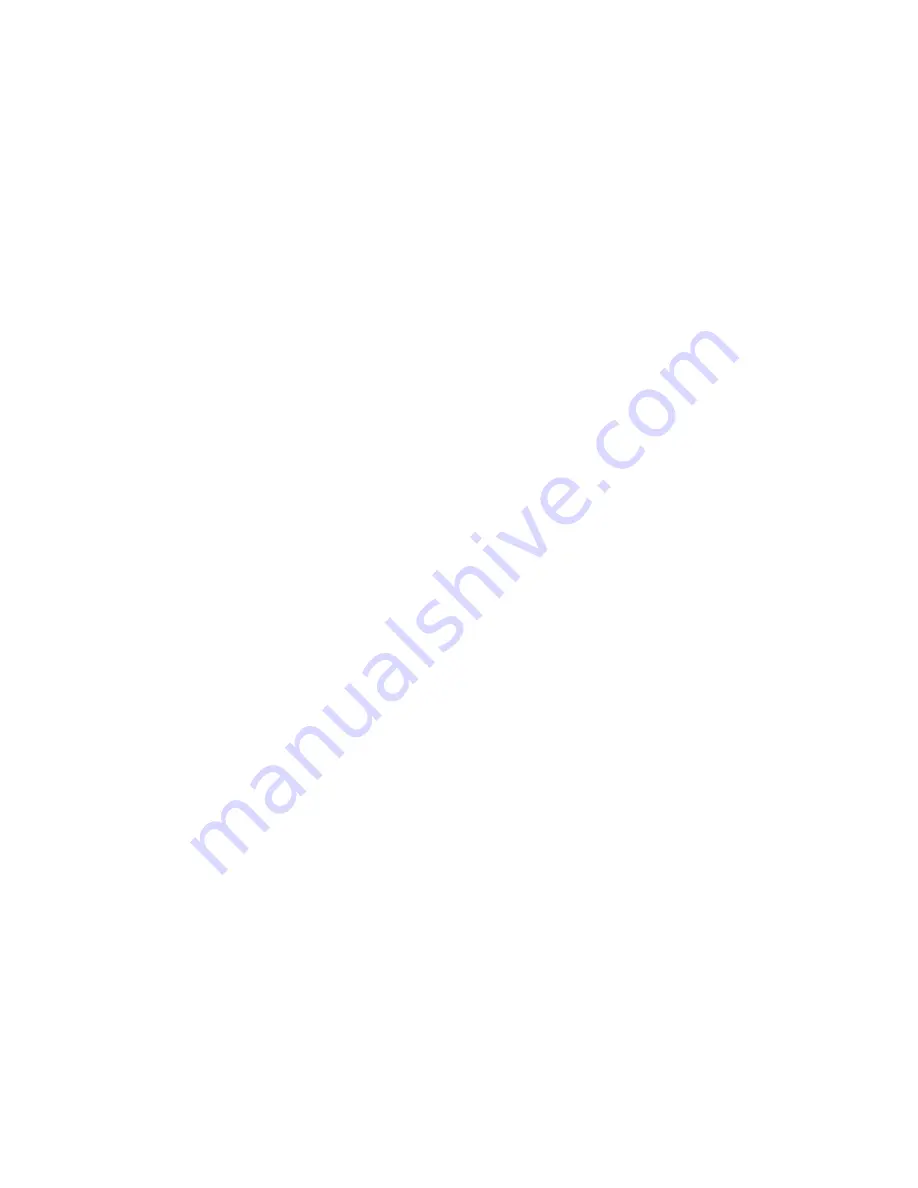
3. Using and understanding the
Valgrind core: Advanced Topics
This chapter describes advanced aspects of the Valgrind core services, which are mostly of interest to power users who
wish to customise and modify Valgrind’s default behaviours in certain useful ways. The subjects covered are:
• The "Client Request" mechanism
• Debugging your program using Valgrind’s gdbserver and GDB
• Function Wrapping
3.1. The Client Request mechanism
Valgrind has a trapdoor mechanism via which the client program can pass all manner of requests and queries to
Valgrind and the current tool.
Internally, this is used extensively to make various things work, although that’s not
visible from the outside.
For your convenience, a subset of these so-called client requests is provided to allow you to tell Valgrind facts about
the behaviour of your program, and also to make queries. In particular, your program can tell Valgrind about things
that it otherwise would not know, leading to better results.
Clients need to include a header file to make this work. Which header file depends on which client requests you use.
Some client requests are handled by the core, and are defined in the header file
valgrind/valgrind.h
. Tool-
specific header files are named after the tool, e.g.
valgrind/memcheck.h
. Each tool-specific header file includes
valgrind/valgrind.h
so you don’t need to include it in your client if you include a tool-specific header. All
header files can be found in the
include/valgrind
directory of wherever Valgrind was installed.
The macros in these header files have the magical property that they generate code in-line which Valgrind can spot.
However, the code does nothing when not run on Valgrind, so you are not forced to run your program under Valgrind
just because you use the macros in this file. Also, you are not required to link your program with any extra supporting
libraries.
The code added to your binary has negligible performance impact: on x86, amd64, ppc32, ppc64 and ARM, the
overhead is 6 simple integer instructions and is probably undetectable except in tight loops. However, if you really
wish to compile out the client requests, you can compile with
-DNVALGRIND
(analogous to
-DNDEBUG
’s effect on
assert
).
You are encouraged to copy the
valgrind/*.h
headers into your project’s include directory, so your program
doesn’t have a compile-time dependency on Valgrind being installed. The Valgrind headers, unlike most of the rest
of the code, are under a BSD-style license so you may include them without worrying about license incompatibility.
Here is a brief description of the macros available in
valgrind.h
, which work with more than one tool (see the
tool-specific documentation for explanations of the tool-specific macros).
RUNNING_ON_VALGRIND
:
Returns 1 if running on Valgrind, 0 if running on the real CPU. If you are running Valgrind on itself, returns the
number of layers of Valgrind emulation you’re running on.
29