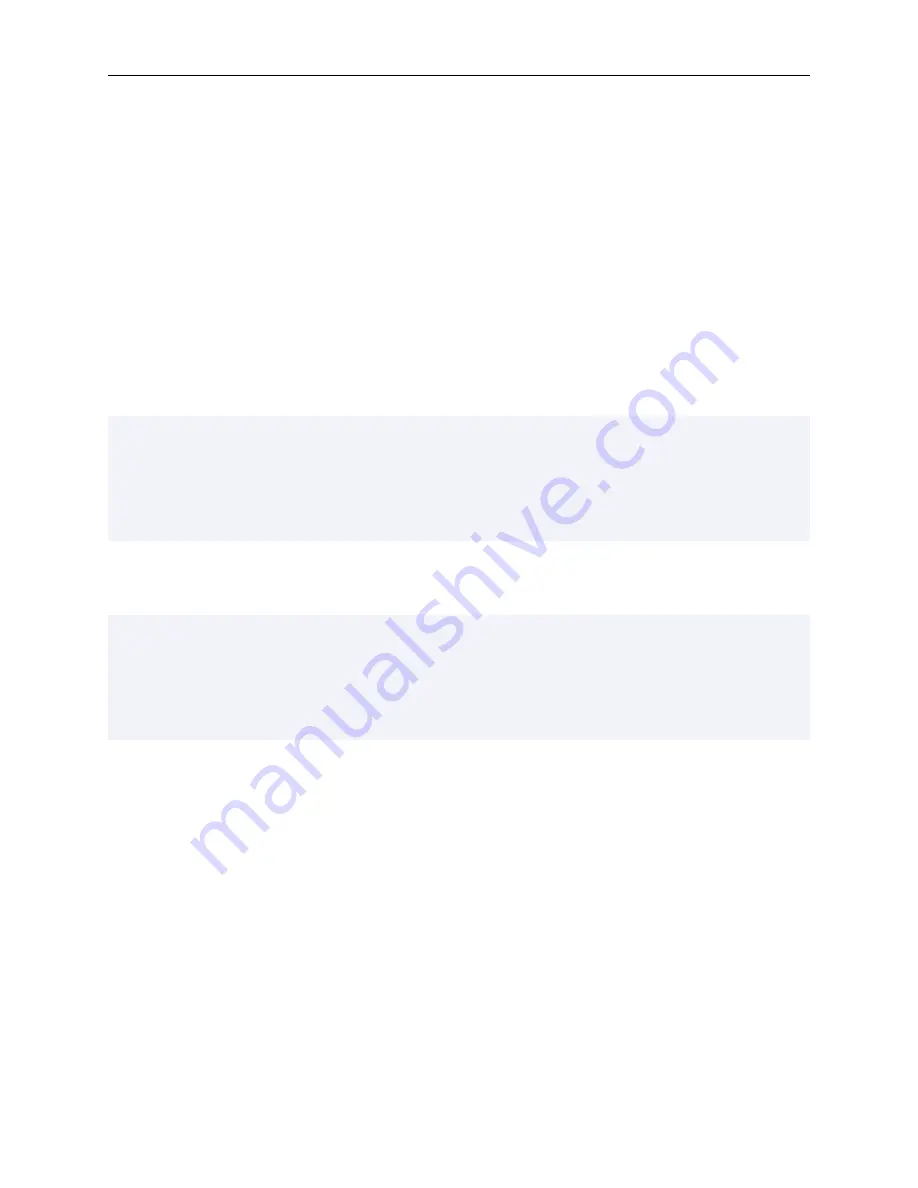
Memcheck: a memory error detector
freed.
Likewise, if it should turn out to be just off the end of a heap block, a common result of off-by-one-
errors in array subscripting, you’ll be informed of this fact, and also where the block was allocated.
If you use
the
--read-var-info
option Memcheck will run more slowly but may give a more detailed description of any
illegal address.
In this example, Memcheck can’t identify the address. Actually the address is on the stack, but, for some reason, this
is not a valid stack address -- it is below the stack pointer and that isn’t allowed. In this particular case it’s probably
caused by GCC generating invalid code, a known bug in some ancient versions of GCC.
Note that Memcheck only tells you that your program is about to access memory at an illegal address. It can’t stop the
access from happening. So, if your program makes an access which normally would result in a segmentation fault,
you program will still suffer the same fate -- but you will get a message from Memcheck immediately prior to this. In
this particular example, reading junk on the stack is non-fatal, and the program stays alive.
4.2.2. Use of uninitialised values
For example:
Conditional jump or move depends on uninitialised value(s)
at 0x402DFA94: _IO_vfprintf (_itoa.h:49)
by 0x402E8476: _IO_printf (printf.c:36)
by 0x8048472: main (tests/manuel1.c:8)
An uninitialised-value use error is reported when your program uses a value which hasn’t been initialised -- in other
words, is undefined.
Here, the undefined value is used somewhere inside the
printf
machinery of the C library.
This error was reported when running the following small program:
int main()
{
int x;
printf ("x = %d\n", x);
}
It is important to understand that your program can copy around junk (uninitialised) data as much as it likes.
Memcheck observes this and keeps track of the data, but does not complain.
A complaint is issued only when
your program attempts to make use of uninitialised data in a way that might affect your program’s externally-visible
behaviour. In this example,
x
is uninitialised.
Memcheck observes the value being passed to
_IO_printf
and
thence to
_IO_vfprintf
, but makes no comment. However,
_IO_vfprintf
has to examine the value of
x
so it
can turn it into the corresponding ASCII string, and it is at this point that Memcheck complains.
Sources of uninitialised data tend to be:
• Local variables in procedures which have not been initialised, as in the example above.
• The contents of heap blocks (allocated with
malloc
,
new
, or a similar function) before you (or a constructor) write
something there.
51