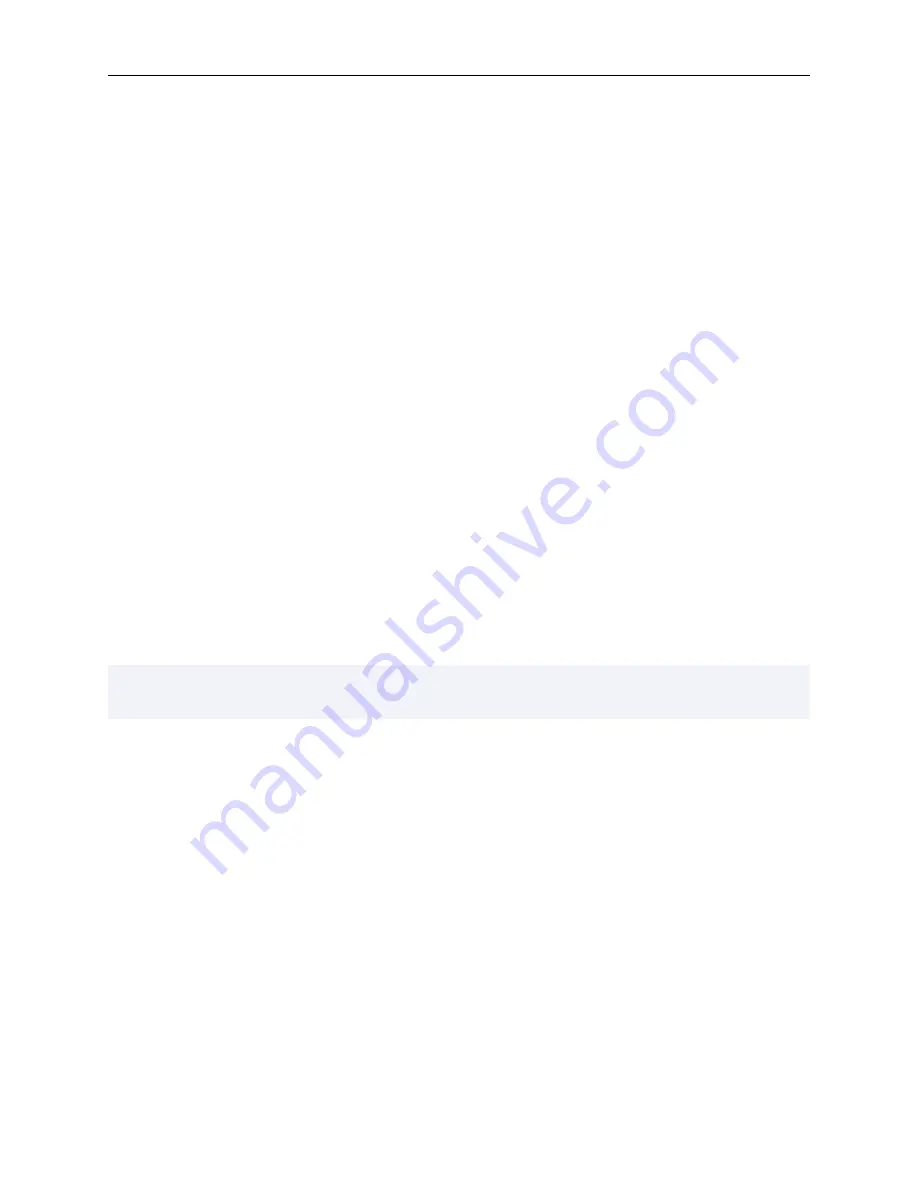
Writing a New Valgrind Tool
The files
include/pub_tool_*.h
contain all the types, macros, functions, etc. that a tool should (hopefully)
need, and are the only
.h
files a tool should need to
#include
. They have a reasonable amount of documentation
in it that should hopefully be enough to get you going.
Note that you can’t use anything from the C library (there are deep reasons for this, trust us). Valgrind provides an
implementation of a reasonable subset of the C library, details of which are in
pub_tool_libc*.h
.
When writing a tool, in theory you shouldn’t need to look at any of the code in Valgrind’s core, but in practice it might
be useful sometimes to help understand something.
The
include/pub_tool_basics.h
and
VEX/pub/libvex_basictypes.h
files have some basic types
that are widely used.
Ultimately, the tools distributed (Memcheck, Cachegrind, Lackey, etc.) are probably the best documentation of all, for
the moment.
The
VG_
macro is used heavily.
This just prepends a longer string in front of names to avoid potential namespace
clashes. It is defined in
include/pub_tool_basics.h
.
There are some assorted notes about various aspects of the implementation in
docs/internals/
. Much of it isn’t
that relevant to tool-writers, however.
2.3. Advanced Topics
Once a tool becomes more complicated, there are some extra things you may want/need to do.
2.3.1. Debugging Tips
Writing and debugging tools is not trivial. Here are some suggestions for solving common problems.
If you are getting segmentation faults in C functions used by your tool, the usual GDB command:
gdb <prog> core
usually gives the location of the segmentation fault.
If you want to debug C functions used by your tool, there are instructions on how to do so in the file
README_DEVELOPERS
.
If you are having problems with your VEX IR instrumentation, it’s likely that GDB won’t be able to help at all. In
this case, Valgrind’s
--trace-flags
option is invaluable for observing the results of instrumentation.
If you just want to know whether a program point has been reached,
using the
OINK
macro (in
include/pub_tool_libcprint.h
) can be easier than using GDB.
The other debugging command line options can be useful too (run
valgrind --help-debug
for the list).
2.3.2. Suppressions
If your tool reports errors and you want to suppress some common ones, you can add suppressions to the suppression
files. The relevant files are
*.supp
; the final suppression file is aggregated from these files by combining the relevant
.supp
files depending on the versions of linux, X and glibc on a system.
5