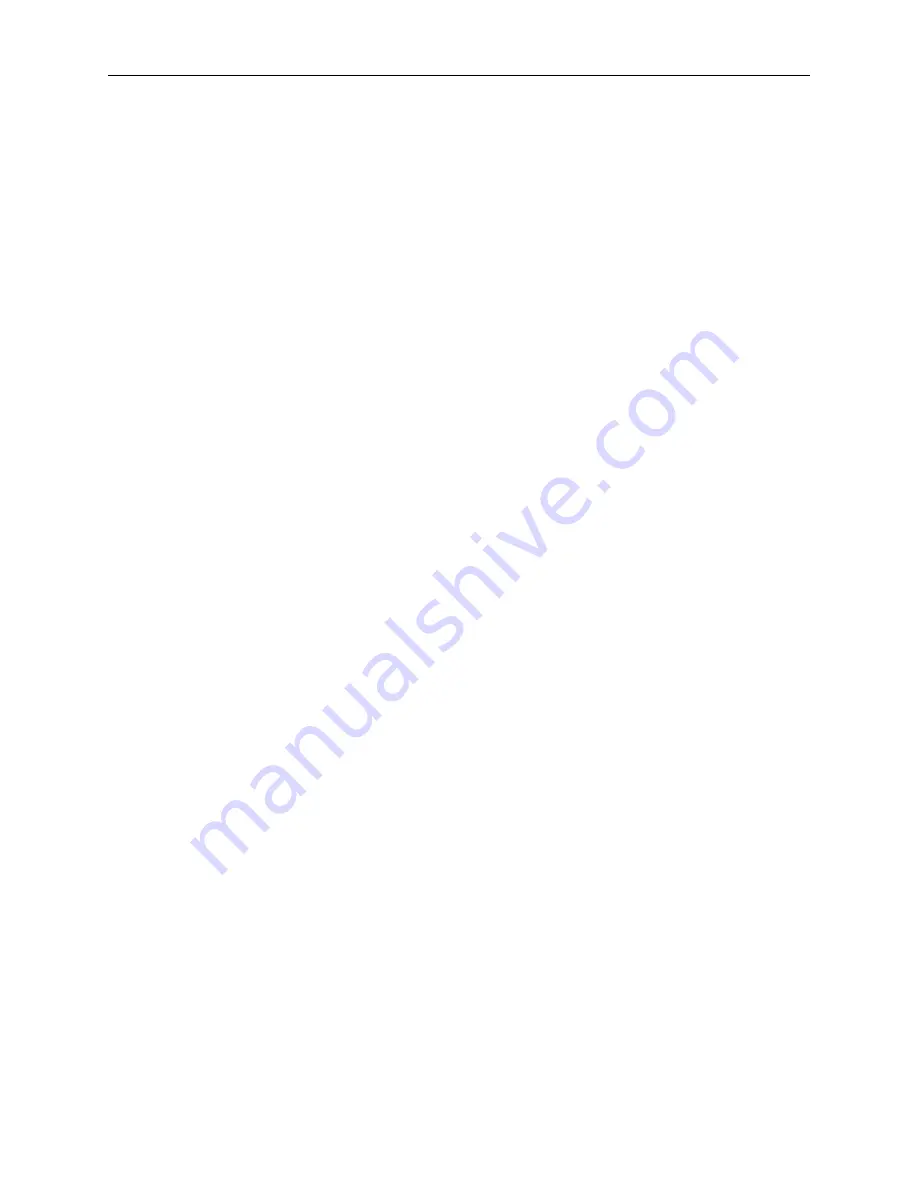
Memcheck: a memory error detector
•
VALGRIND_COUNT_LEAKS
: fills in the four arguments with the number of bytes of memory found by
the previous leak check to be leaked (i.e.
the sum of direct leaks and indirect leaks), dubious, reach-
able and suppressed.
This is useful in test harness code, after calling
VALGRIND_DO_LEAK_CHECK
or
VALGRIND_DO_QUICK_LEAK_CHECK
.
•
VALGRIND_COUNT_LEAK_BLOCKS
: identical to
VALGRIND_COUNT_LEAKS
except that it returns the number
of blocks rather than the number of bytes in each category.
•
VALGRIND_GET_VBITS
and
VALGRIND_SET_VBITS
: allow you to get and set the V (validity) bits for an
address range. You should probably only set V bits that you have got with
VALGRIND_GET_VBITS
. Only for
those who really know what they are doing.
•
VALGRIND_CREATE_BLOCK
and
VALGRIND_DISCARD
.
VALGRIND_CREATE_BLOCK
takes an address, a
number of bytes and a character string.
The specified address range is then associated with that string.
When
Memcheck reports an invalid access to an address in the range, it will describe it in terms of this block rather than
in terms of any other block it knows about. Note that the use of this macro does not actually change the state of
memory in any way -- it merely gives a name for the range.
At some point you may want Memcheck to stop reporting errors in terms of the block named by
VALGRIND_CREATE_BLOCK
.
To make this possible,
VALGRIND_CREATE_BLOCK
returns a "block
handle", which is a C
int
value.
You can pass this block handle to
VALGRIND_DISCARD
. After doing so,
Valgrind will no longer relate addressing errors in the specified range to the block.
Passing invalid handles to
VALGRIND_DISCARD
is harmless.
4.8. Memory Pools: describing and working
with custom allocators
Some programs use custom memory allocators, often for performance reasons.
Left to itself, Memcheck is unable
to understand the behaviour of custom allocation schemes as well as it understands the standard allocators, and so
may miss errors and leaks in your program.
What this section describes is a way to give Memcheck enough of a
description of your custom allocator that it can make at least some sense of what is happening.
There are many different sorts of custom allocator, so Memcheck attempts to reason about them using a loose, abstract
model. We use the following terminology when describing custom allocation systems:
• Custom allocation involves a set of independent "memory pools".
• Memcheck’s notion of a a memory pool consists of a single "anchor address" and a set of non-overlapping "chunks"
associated with the anchor address.
• Typically a pool’s anchor address is the address of a book-keeping "header" structure.
• Typically the pool’s chunks are drawn from a contiguous "superblock" acquired through the system
malloc
or
mmap
.
69