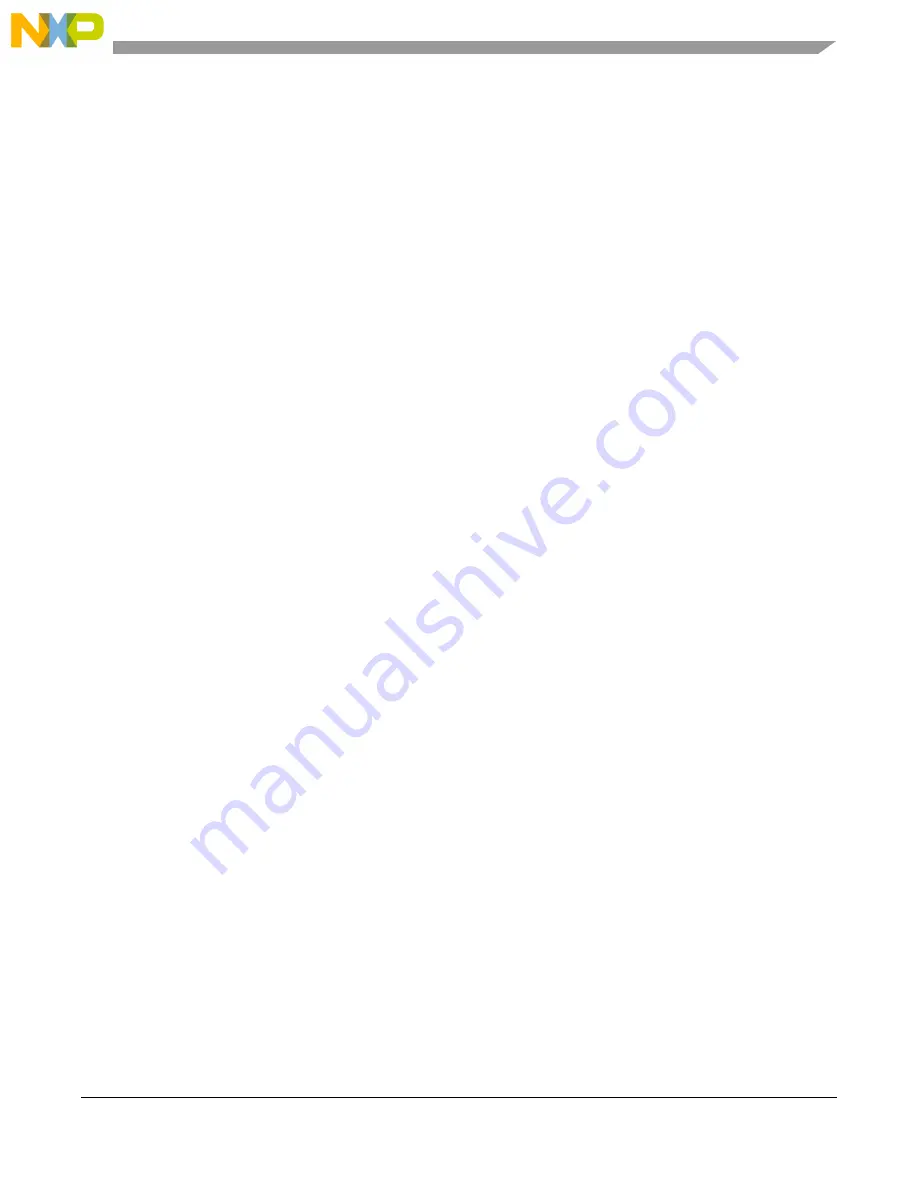
Using the Serial Peripheral Interface (SPI) for the QE Microcontrollers
QE128 Quick Reference User Guide, Rev. 1.0
Freescale Semiconductor
11-7
void main(void) {
UINT8 counter = 0;
MCU_Init(); // Function that initializes the MCU
GPIO_Init(); // Function that initializes the Ports of the MCU
SPI_Init(); // Function that initializes the SPI module
EnableInterrupts; // enable interrupts
for(;;) {
delay(60000); // Delay function
while (!SPI2S_SPTEF && !PTDD_PTDD3); // Wait until transmit buffer is empty
PTDD_PTDD3 = 0; // Slave Select set in low
SPI2D = counter; // Put in SPI buffer a data to send
PTED = (UINT8) (counter & 0xC0); // Move the adquired ADC value to port E
PTCD = (UINT8) (counter & 0x3F); // Move the adquired ADC value to port C
+; // Increment counter
} // loop forever
// please make sure that you never leave this function
}
11.3.1.2
SPI Slave Project
The project SPI_Slave configures the SPI module in slave mode. The main functions are:
•
main — Waits for the SPI interrupt to occur.
•
MCU_Init – MCU initialization, watchdog disable and the SPI clock module enabled.
•
GPIO_Init – Configure PTE port as output.
•
SPI_Init – SPI module configuration.
•
SPI_ISR — Display the received data in eight LEDs
The firmware for this project is much similar to SPI_master project. The differences are that the device is
configured as slave and only Receives a byte and displays it on the PTE port.
This is the initialization code for Serial Peripheral Interface module used for the QE MCU. This
application configures the SPI module to work in slave mode.
void SPI_Init (void) {
SPI2BR = 0x75; // Select the highest baud rate prescaler divisor and the
// highest baud rate divisor
SPI2C1 = 0xC4; // SPI Interrupt enable, system enable and slave mode selected
SPI2C2 = 0x00; // Different pins for data input and data output
}
NOTE
This is the SPI interrupt service routine. This routine is used when a byte is
sent by the master to the slave.
void interrupt VectorNumber_Vspi2 SPI_ISR(void) {
UINT8 temp, buffer;
while (PTDD_PTDD0);
temp = SPI2S; // Clear register flag
buffer = ~SPI2D; // Read data register to clear receive flag
// on the LEDs (The LEDs turn on with 0's)
PTED = (UINT8) (temp & 0xC0); // Move the adquired ADC value to port E
PTCD = (UINT8) (temp & 0x3F); // Move the adquired ADC value to port C