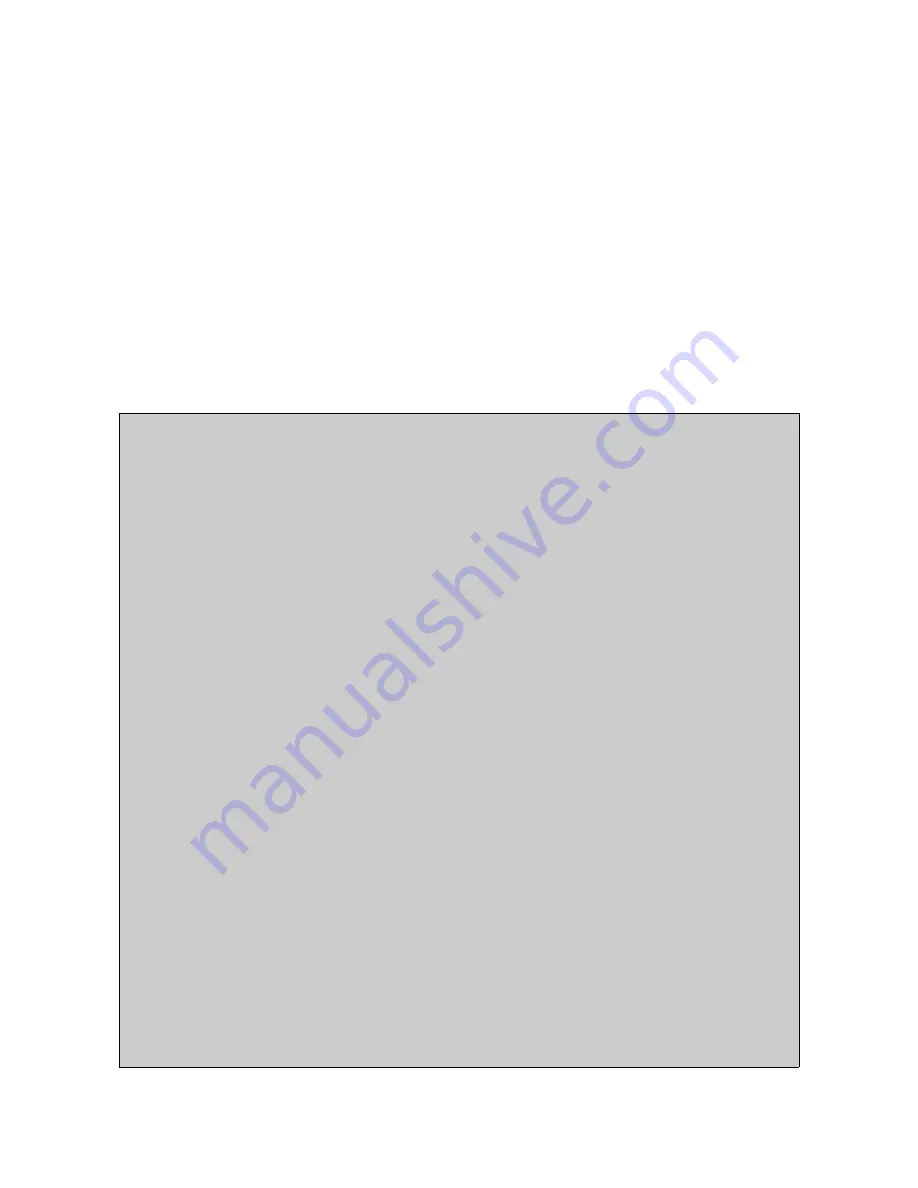
QuercusVL Programming Manual
2.2. C
The method used by the C library to generate events, so that the client application is in charge
of the implementation, is forcing the client application to implement a class according to a
specific interface. When an event occurs in a detector (for instance, generation of a real time
event), the library will call the corresponding method (OnRealTimeInformation in this case).
Take into account that these methods will be called from execution threads which are different
from the main one, so code that is not safe about threads or unable to be executed out of the
main thread, cannot not be used for the implementation.
A short C program is shown next, which is capable of capturing events.
It corresponds to the basic C sample located at Samples folder in the installation directory.
/*------------------------------------------,
| Copyright (C) 2011 Quercus Technologies |
| All rights reserved. |
`------------------------------------------*/
#include <stdio.h>
#include "VLWrapperC.h"
typedef enum
{
DT_ALL=0,
DT_SPEED=1,
DT_QUEUE=2,
DT_PRESENCE=3,
DT_RED_LIGHT=4,
DT_STOPPED_CAR=5
} VLDetectorType;
void __stdcall OnRealTimeInformation(int info)
{
printf("OnRealTimeInformation (%d ,%d) ",
VL_Unit_get_Id(VL_RealTimeInformation_get_Unit(info)),
VL_RealTimeInformation_get_DetectorId(info));
switch(VL_RealTimeInformation_get_DetectorType(info))
{
case 1: printf("DT_SPEED\n");
break;
case 2: printf("DT_QUEUE\n");
break;
case 3: printf("DT_PRESENCE\n");
break;
default: break;
}
}
void __stdcall OnIncidence(int inc)
{
printf("OnIncidence (%d ,%d), %d, ",
VL_Unit_get_Id(VL_Incidence_get_Unit(inc)),
VL_Incidence_get_DetectorId(inc),VL_Incidence_get_Id(inc));
switch(VL_Incidence_get_Type(inc))
{
case 1: printf("IT_RED_LIGHT_VIOLATION\n");
break;
case 2: printf("IT_STOPPED_CAR_VIOLATION\n"); break;
default: break;
}
}
void __stdcall OnSummary(int sum)
{
printf("OnSummary (%d ,%d) ",
VL_Unit_get_Id(VL_Summary_get_Unit(sum)),VL_Summary_get_DetectorId(sum));
Quercus Technologies
20