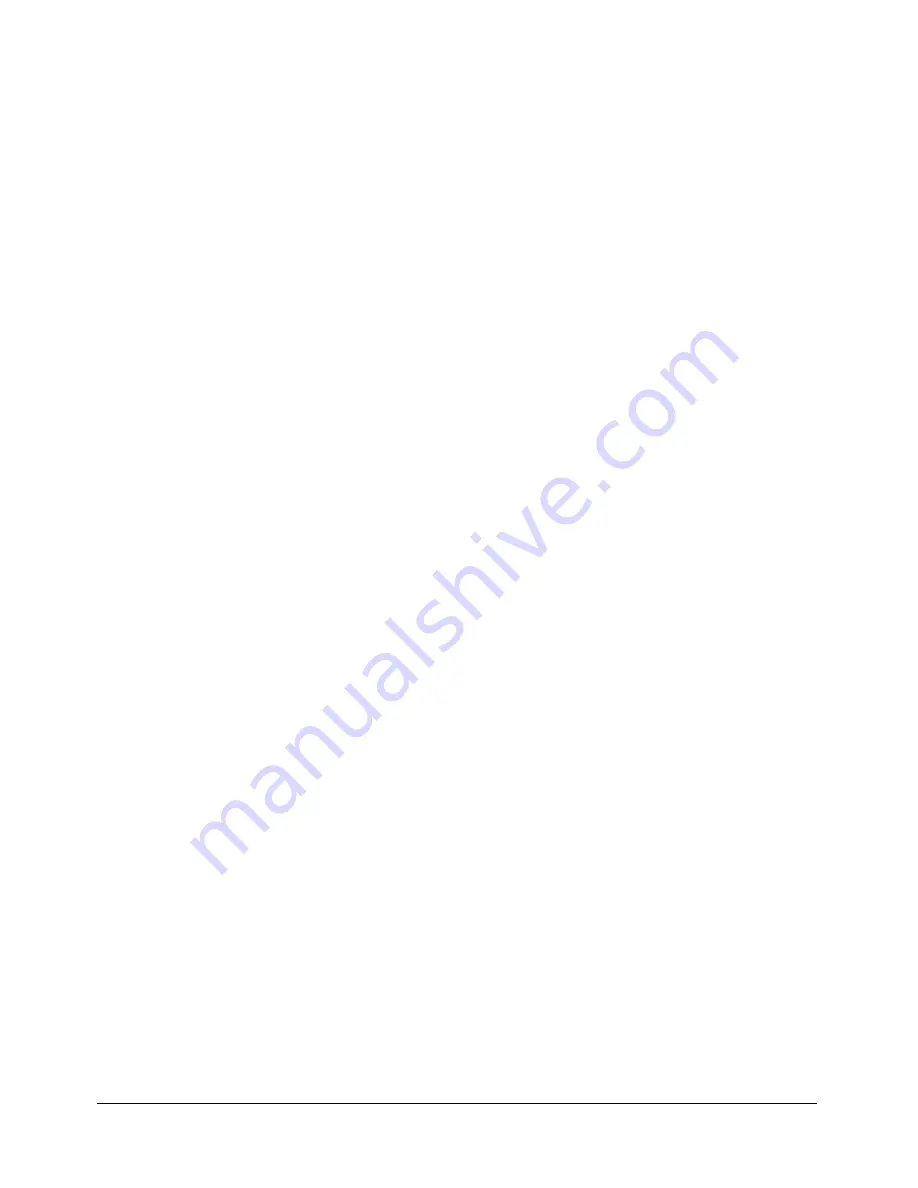
70
Chapter 3: Writing Scripts in Director
The following example defines a function named
Car_increaseSpeed()
. The function name is
then assigned to the
increaseSpeed
property of the
Car
class’s prototype object.
// increase the speed of a Car
function Car_increaseSpeed(x) {
this.speed += x;
return this.speed;
}
Car.prototype.increaseSpeed = Car_increaseSpeed;
An object instance of
Car
could then access the
increaseSpeed()
method and assign its value to
a variable by using the following syntax.
var objCar = new Car("Subaru", "Forester", "silver");
var newSpeed = objCar.increaseSpeed(30);
You can also create an instance method by using function literal syntax. Using function literal
syntax eliminates the need to define a function, and the need to assign a property name to the
function name.
The following example uses function literal syntax to define an
increaseSpeed()
method that
contains the same functionality as the
increaseSpeed()
function defined previously.
// increase the speed of a Car
Car.prototype.increaseSpeed = function(x) {
this.speed += x;
return this.speed;
}
Class variables
Also known as
static
variables, these are any variables (properties) that are associated with a class,
and not an object instance. There is always only one copy of a class variable, regardless of the
number of object instances that are created from that class. Class variables do not use the
prototype object to implement inheritance. You access a class variable directly through the class,
and not through an object instance; you must define a class in a constructor function before you
can define class variables.
The following example defines two class variables—
MAX_SPEED
and
MIN_SPEED
.
function Car() { // define a Car class
...
}
Car.MAX_SPEED = 165;
Car.MIN_SPEED = 45;
You would access the
MAX_SPEED
and
MIN_SPEED
class variables directly from the
Car
class.
var carMaxSpeed = Car.MAX_SPEED; // carMaxSpeed = 165
var carMinSpeed = Car.MIN_SPEED; // carMinSpeed = 45
Class methods
Also known as
static
methods, these are any methods that are associated with a class, and not an
object instance. There is always only one copy of a class method, regardless of the number of
object instances that are created from that class. Class methods do not use the prototype object to
implement inheritance. You access a class method directly through the class, and not through
an object instance; you must define a class in a constructor function before you can define
class methods.
Summary of Contents for DIRECTOR MX 2004-DIRECTOR SCRIPTING
Page 1: ...DIRECTOR MX 2004 Director Scripting Reference...
Page 48: ...48 Chapter 2 Director Scripting Essentials...
Page 100: ...100 Chapter 4 Debugging Scripts in Director...
Page 118: ...118 Chapter 5 Director Core Objects...
Page 594: ...594 Chapter 12 Methods...
Page 684: ...684 Chapter 14 Properties See also DVD...
Page 702: ...702 Chapter 14 Properties See also face vertices vertices flat...
Page 856: ...856 Chapter 14 Properties JavaScript syntax sprite 15 member member 3 4...
Page 1102: ...1102 Chapter 14 Properties...