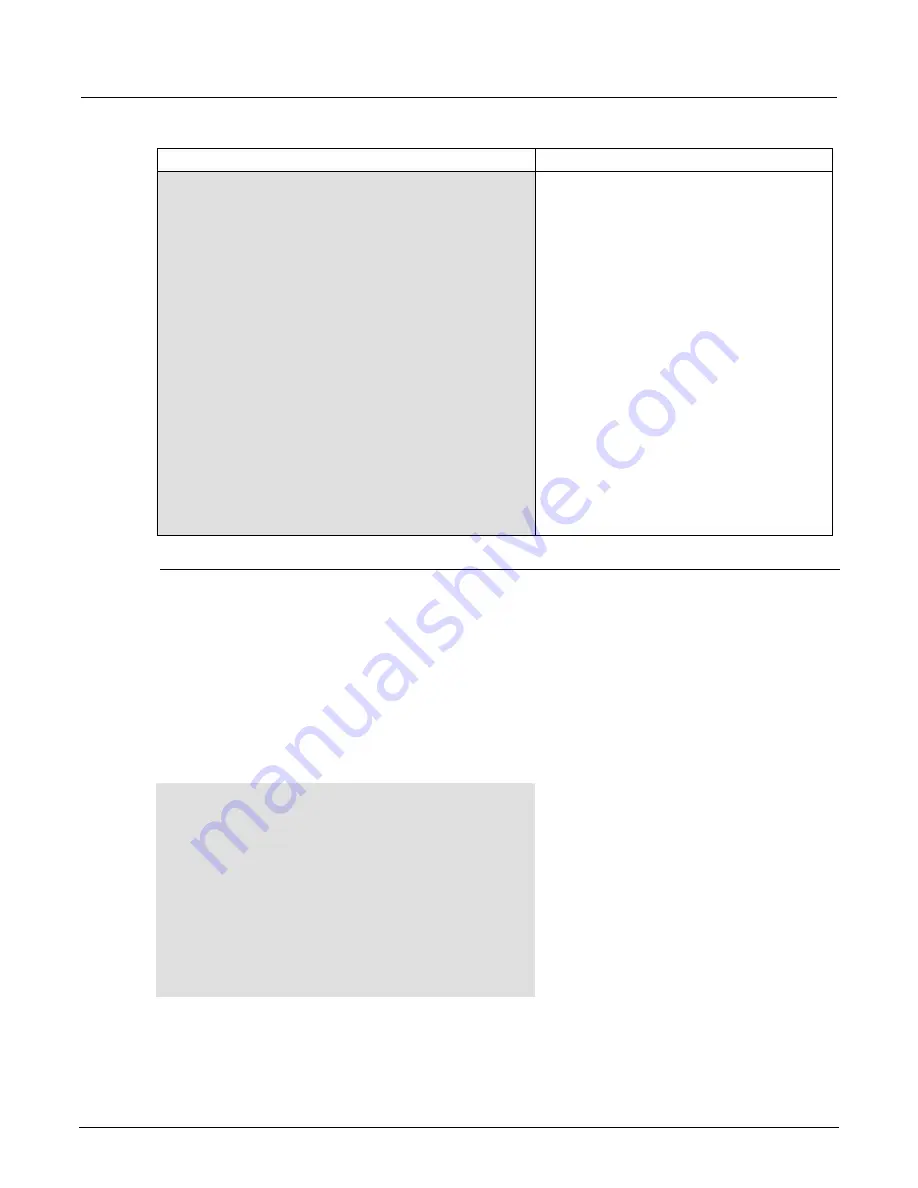
Models 707B and 708B Switching Matrix Reference Manual
Section 6: Instrument programming
707B-901-01 Rev. A / August 2010
6-29
Example: Break with infinite loop
Code Notes
a, b = 0, 1
while true do
print(a,b)
a, b = b, a + b
if a > 500 then
break
end
end
This example uses a
break
statement that
causes the while loop to exit if the value of
a
becomes greater than 500.
Output:
0.000 1.000
1.000 1.000
1.000 2.000
2.000 3.000
3.000 5.000
5.000 8.000
8.000 1.301
1.301 2.101
2.101 3.401
3.401 5.501
5.501 8.901
8.901 1.402
1.402 2.302
2.302 3.702
3.702 6.102
Tables and arrays
Lua makes extensive use of the data type table, which is a flexible array-like data type. Table indices
start with 1. Tables can be indexed not only with numbers, but with any value (except
nil
). Tables
can be heterogeneous, which means that they can contain values of all types (except
nil
).
Tables are the sole data structuring mechanism in Lua; they may be used to represent ordinary
arrays, symbol tables, sets, records, graphs, trees, and so on. To represent records, Lua uses the
field
name
as an index. The language supports this representation by providing
a.name
as an easier
way to express
a["name"]
.
Example: Loop array
Code Notes
and
output
atable = {1, 2, 3, 4}
i = 1
while atable[i] do
print(atable[i])
i = i + 1
end
Defines a table with four numeric elements.
Loops through the array and prints each
element.
The Boolean value of
atable[index]
evaluates to
true
if there is an element at that
index. If there is no element at that index,
nil
is returned (
nil
is considered to be
false
).
Output:
1.000
2.000
3.000
4.000