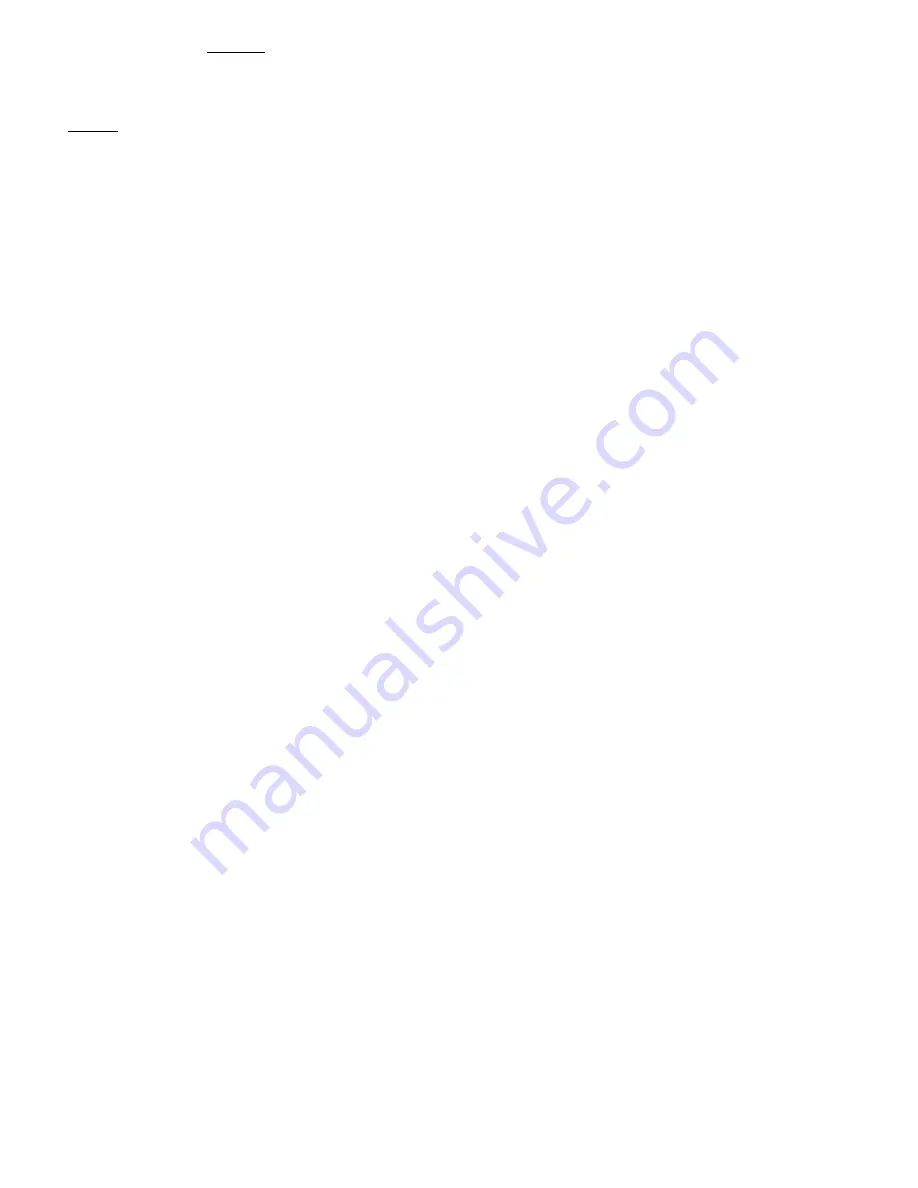
See the low-level function description in Section 5.2.19 for more information about these parameters:
LJ_chI2C_ADDRESS_BYTE
LJ_chI2C_SCL_PIN_NUM // 0-19. Pull-up resistor usually required.
LJ_chI2C_SDA_PIN_NUM // 0-19. Pull-up resistor usually required.
LJ_chI2C_OPTIONS
LJ_chI2C_SPEED_ADJUST
The LJTick-DAC is an accessory from LabJack with an I²C 24C01C EEPROM chip. Following is example pseudocode to
configure I²C to talk to that chip:
//The AddressByte of the EEPROM on the LJTick-DAC is 0xA0 or decimal 160.
AddRequest(lngHandle, LJ_ioPUT_CONFIG, LJ_chI2C_ADDRESS_BYTE,160,0,0);
//SCL is FIO0
AddRequest(lngHandle, LJ_ioPUT_CONFIG, LJ_chI2C_SCL_PIN_NUM,0,0,0);
//SDA is FIO1
AddRequest(lngHandle, LJ_ioPUT_CONFIG, LJ_chI2C_SDA_PIN_NUM,1,0,0);
//See description of low-level I2C function.
AddRequest(lngHandle, LJ_ioPUT_CONFIG, LJ_chI2C_OPTIONS,0,0,0);
//See description of low-level I2C function. 0 is max speed of about 150 kHz.
AddRequest(lngHandle, LJ_ioPUT_CONFIG, LJ_chI2C_SPEED_ADJUST,0,0,0);
//Execute the configuration requests.
GoOne(lngHandle);
Following is pseudocode to read 4 bytes from the EEPROM:
//Initial read of EEPROM bytes 0-3 in the user memory area.
//We need a single I2C transmission that writes the address and then reads
//the data. That is, there needs to be an ack after writing the address,
//not a stop condition. To accomplish this, we use Add/Go/Get to combine
//the write and read into a single low-level call.
numWrite = 1;
array[0] = 0; //Memory address. User area is 0-63.
AddRequest(lngHandle, LJ_ioI2C_COMMUNICATION, LJ_chI2C_WRITE, numWrite, array, 0);
numRead = 4;
AddRequest(lngHandle, LJ_ioI2C_COMMUNICATION, LJ_chI2C_READ, numRead, array, 0);
//Execute the requests.
GoOne(lngHandle);
For more example code, see the I2C.cpp example in the VC6_LJUD archive.
4.3.12 - Asynchronous Serial Communication
The U3 (hardware version 1.21+ only) has a UART available that supports asynchronous serial communication. On hardware
version 1.30 the TX (transmit) and RX (receive) lines appear on FIO/EIO after any timers and counters, so with no timers/counters
enabled, and pin offset set to 4, TX=FIO4 and RX=FIO5. On hardware version 1.21, the UART uses SDA for TX and SCL for RX.
Communication is in the common 8/n/1 format. Similar to RS-232, except that the logic is normal CMOS/TTL. Connection to an
RS-232 device will require a converter chip such as the MAX233, which inverts the logic and shifts the voltage levels.
This serial link is not an alternative to the USB connection. Rather, the host application will write/read data to/from the U3 over
USB, and the U3 communicates with some other device using the serial protocol. Using this serial protocol is considered an
advanced topic. A good knowledge of the protocol is recommended, and a logic analyzer or oscilloscope might be needed for
troubleshooting. Also consider that a better way to do RS-232 (or RS-485 or RS-422) communication is with a standard
USB<=>RS-232 adapter/converter/dongle, so the user should have a particular reason to not use that and use a U3 instead.
There is one IOType used to write/read asynchronous data:
LJ_ioASYNCH_COMMUNICATION
The following are special channels used with the asynch IOType above:
LJ_chASYNCH_ENABLE // Enables UART to begin buffering rx data.
LJ_chASYNCH_RX // Value= returns pre-read buffer size. x1= array.
LJ_chASYNCH_TX // Value= number to send (0-56), number in rx buffer. x1= array.
LJ_chASYNCH_FLUSH // Flushes the rx buffer. All data discarded. Value ignored.
When using
LJ_chASYNCH_RX
, the Value parameter returns the size of the Asynch buffer before the read. If the size is 32 bytes
or less, that is how many bytes were read. If the size is more than 32 bytes, then the call read 32 this time and there are still bytes
left in the buffer.
When using
LJ_chASYNCH_TX
, specify the number of bytes to send in the Value parameter. The Value parameter returns the
size of the Asynch read buffer.
The following is a special channel, used with the get/put config IOTypes, to specify the baud rate for the asynchronous
communication:
LJ_chASYNCH_BAUDFACTOR // 16-bit value for hardware V1.30. 8-bit for V1.21.
With hardware revision 1.30 this is a 16-bit value that sets the baud rate according the following formula: BaudFactor16 = 2^16 –
48000000/(2 * Desired Baud). For example, a BaudFactor16 = 63036 provides a baud rate of 9600 bps. With hardware revision
1.21, the value is only 8-bit and the formula is BaudFactor8 = 2^8 – TimerClockBase/(Desired Baud).
Following is example pseudocode for asynchronous communication:
//Set data rate for 9600 bps communication.
ePut(lngHandle, LJ_ioPUT_CONFIG, LJ_chASYNCH_BAUDFACTOR, 63036, 0);
//Enable UART.
ePut(lngHandle, LJ_ioASYNCH_COMMUNICATION, LJ_chASYNCH_ENABLE, 1, 0);
//Write data.
eGet(lngHandle, LJ_ioASYNCH_COMMUNICATION, LJ_chASYNCH_TX, &numBytes, array);
//Read data. Always initialize array to 32 bytes.
eGet(lngHandle, LJ_ioASYNCH_COMMUNICATION, LJ_chASYNCH_RX, &numBytes, array);
4.3.13 - Watchdog Timer
The U3 (hardware version 1.21+ only) has firmware based watchdog capability. Unattended systems requiring maximum up-time
might use this capability to reset the U3 or the entire system. When any of the options are enabled, an internal timer is enabled
which resets on any incoming USB communication. If this timer reaches the defined TimeoutPeriod before being reset, the
specified actions will occur. Note that while streaming, data is only going out, so some other command will have to be called
40