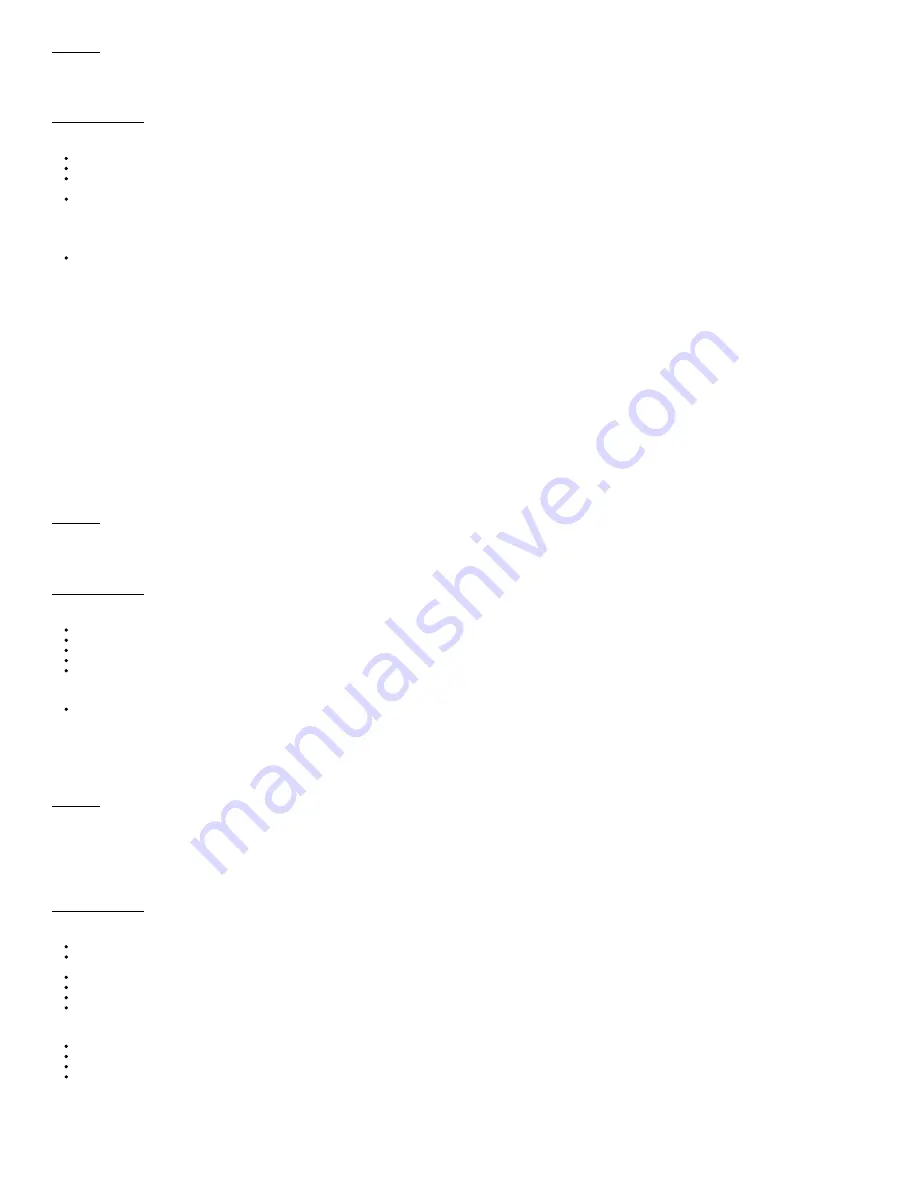
Declaration:
LJ_ERROR _stdcall OpenLabJack ( long DeviceType,
long ConnectionType,
const char *pAddress,
long FirstFound,
LJ_HANDLE *pHandle)
Parameter Description:
Returns: LabJack errorcodes or 0 for no error.
Inputs:
DeviceType
– The type of LabJack to open. Constants are in the labjackud.h file.
ConnectionType
– Enter the constant for the type of connection, USB or Ethernet.
pAddress
– For USB, pass the local ID or serial number of the desired LabJack. For Ethernet pass the IP address of the
desired LabJack. If FirstFound is true, Address is ignored.
FirstFound
– If true, then the Address and ConnectionType parameters are ignored and the driver opens the first LabJack
found with the specified DeviceType. Generally only recommended when a single LabJack is connected. Currently only
supported with USB. If a USB device is not found, it will try Ethernet but with the given Address.
Outputs:
pHandle
– A pointer to a handle for a LabJack.
4.2.3 - eGet() and ePut()
The eGet and ePut functions do AddRequest, Go, and GetResult, in one step.
The eGet versions are designed for inputs or retrieving parameters as they take a pointer to a double where the result is placed,
but can be used for outputs if pValue is preset to the desired value. This is also useful for things like StreamRead where a value is
input and output (number of scans requested and number of scans returned).
The ePut versions are designed for outputs or setting configuration parameters and will not return anything except the errorcode.
eGetS() and ePutS() are special versions of these functions where IOType is a string rather than a long. This is useful for passing
string constants in languages that cannot include the header file, and is generally used with all IOTypes except put/get config. The
string should contain the constant name as indicated in the header file (such as “LJ_ioANALOG_INPUT”). The declarations for the
S versions are the same as the normal versions except for (…, const char *pIOType, …).
eGetSS() and ePutSS() are special versions of these functions where IOType and Channel are strings rather than longs. This is
useful for passing string constants in languages that cannot include the header file, and is generally only used with the put/get
config IOTypes. The strings should contain the constant name as indicated in the header file (such as “LJ_ioPUT_CONFIG” and
“LJ_chLOCALID”). The declaration for the SS versions are the same as the normal versions except for (…, const char *pIOType,
const char *pChannel, …).
The declaration for ePut is the same as eGet except that Value is not a pointer (…, double Value, …), and thus is an input only.
Declaration:
LJ_ERROR _stdcall eGet ( LJ_HANDLE Handle,
long IOType,
long Channel,
double *pValue,
long x1)
Parameter Description:
Returns: LabJack errorcodes or 0 for no error.
Inputs:
Handle
– Handle returned by OpenLabJack().
IOType
– The type of request. See Section 4.3.
Channel
– The channel number of the particular IOType.
pValue
– Pointer to Value sends and receives data.
x1
– Optional parameter used by some IOTypes.
Outputs:
pValue
– Pointer to Value sends and receives data.
4.2.4 - eAddGoGet()
This function passes multiple requests via arrays, then executes a GoOne() and returns all the results via the same arrays.
The parameters that start with “*a” are arrays, and all must be initialized with at least a number of elements equal to NumRequests.
Declaration:
LJ_ERROR _stdcall eAddGoGet ( LJ_HANDLE Handle,
long NumRequests,
long *aIOTypes,
long *aChannels,
double *aValues,
long *ax1s,
long *aRequestErrors,
long *GoError,
long *aResultErrors)
Parameter Description:
Returns: LabJack errorcodes or 0 for no error.
Inputs:
Handle
– Handle returned by OpenLabJack().
NumRequests
– This is the number of requests that will be made, and thus the number of results that will be returned. All the
arrays must be initialized with at least this many elements.
aIOTypes
– An array which is the list of IOTypes.
aChannels
– An array which is the list of Channels.
aValues
– An array which is the list of Values to write.
ax1s
– An array which is the list of x1s.
Outputs:
aValues
– An array which is the list of Values read.
aRequestErrors
– An array which is the list of errorcodes from each AddRequest().
GoError
– The errorcode returned by the GoOne() call.
aResultErrors
– An array which is the list of errorcodes from each GetResult().
4.2.5 - AddRequest()
Adds an item to the list of requests to be performed on the next call to Go() or GoOne().
27