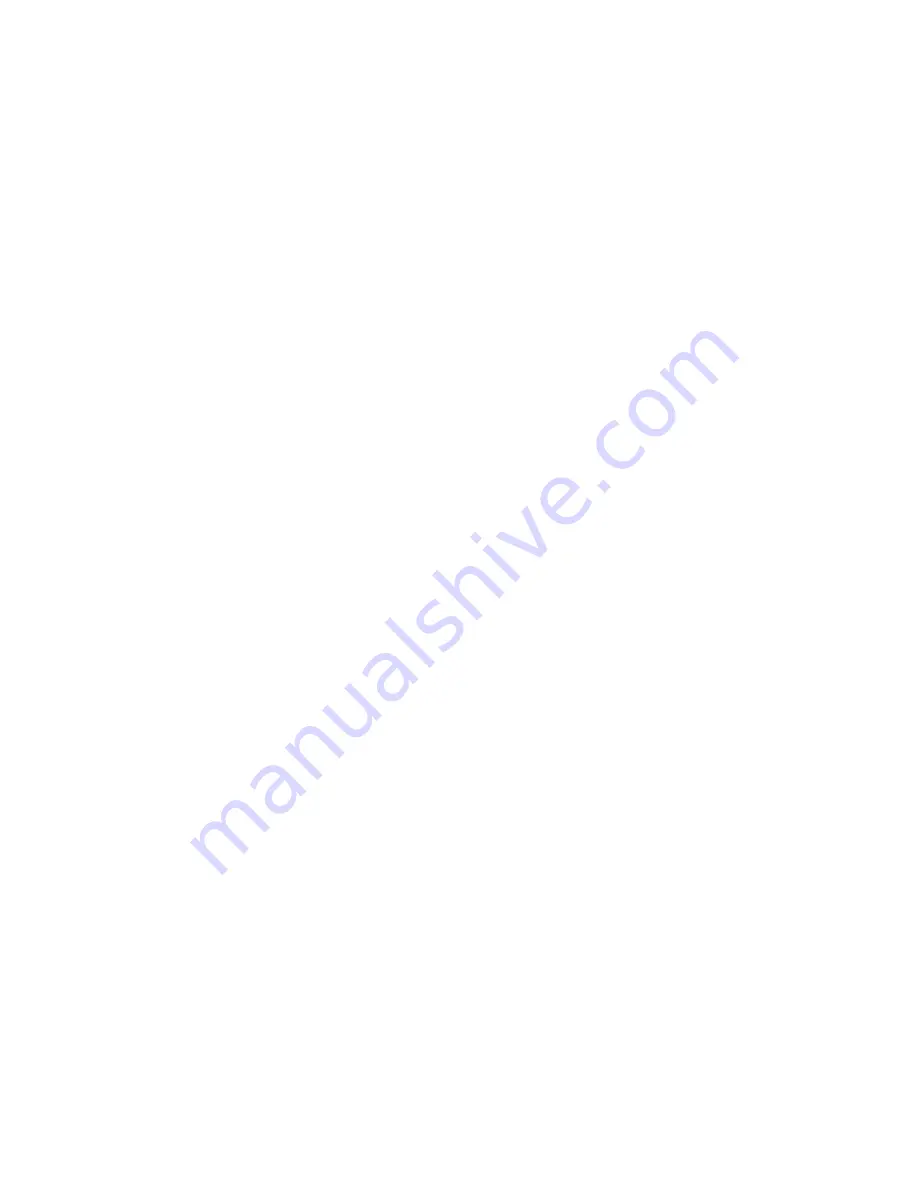
//pin assignments are in the factory default condition.
//The ePut function is used, which combines the add/go/get.
ePut (lngHandle, LJ_ioPIN_CONFIGURATION_RESET, 0, 0, 0);
//First, an add/go/get block to configure the timers and counters.
//Set the pin offset to 4, which causes the timers to start on FIO4.
AddRequest (lngHandle, LJ_ioPUT_CONFIG, LJ_chTIMER_COUNTER_PIN_OFFSET, 4, 0, 0);
//Enable both timers. They will use FIO4-FIO5
AddRequest (lngHandle, LJ_ioPUT_CONFIG, LJ_chNUMBER_TIMERS_ENABLED, 2, 0, 0);
//Make sure Counter0 is disabled.
AddRequest (lngHandle, LJ_ioPUT_COUNTER_ENABLE, 0, 0, 0, 0);
//Enable Counter1. It will use the next available line, FIO6.
AddRequest (lngHandle, LJ_ioPUT_COUNTER_ENABLE, 1, 1, 0, 0);
//All output timers use the same timer clock, configured here. The
//base clock is set to 48MHZ_DIV, meaning that the clock divisor
//is supported and Counter0 is not available. Note that this timer
//clock base is not valid with U3 hardware version 1.20.
AddRequest (lngHandle, LJ_ioPUT_CONFIG, LJ_chTIMER_CLOCK_BASE, LJ_tc48MHZ_DIV, 0, 0);
//Set the timer clock divisor to 48, creating a 1 MHz timer clock.
AddRequest (lngHandle, LJ_ioPUT_CONFIG, LJ_chTIMER_CLOCK_DIVISOR, 48, 0, 0);
//Configure Timer0 as 8-bit PWM. It will have a frequency
//of 1M/256 = 3906.25 Hz.
AddRequest (lngHandle, LJ_ioPUT_TIMER_MODE, 0, LJ_tmPWM8, 0, 0);
//Initialize the 8-bit PWM with a 50% duty cycle.
AddRequest (lngHandle, LJ_ioPUT_TIMER_VALUE, 0, 32768, 0, 0);
//Configure Timer1 as duty cycle input.
AddRequest (lngHandle, LJ_ioPUT_TIMER_MODE, 1, LJ_tmDUTYCYCLE, 0, 0);
//Execute the requests.
GoOne (lngHandle);
The following pseudocode demonstrates reading input timers/counters and updating the values of output timers. The e-functions
are used in the following pseudocode, but some applications might combine the following calls into a single add/go/get block so
that a single low-level call is used.
//Change Timer0 PWM duty cycle to 25%.
ePut (lngHandle, LJ_ioPUT_TIMER_VALUE, 0, 49152, 0);
//Read duty-cycle from Timer1.
eGet (lngHandle, LJ_ioGET_TIMER, 1, &dblValue, 0);
//The duty cycle read returns a 32-bit value where the
//least significant word (LSW) represents the high time
//and the most significant word (MSW) represents the low
//time. The times returned are the number of cycles of
//the timer clock. In this case the timer clock was set
//to 1 MHz, so each cycle is 1 microsecond.
dblHighCycles = (double)(((unsigned long)dblValue) % (65536));
dblLowCycles = (double)(((unsigned long)dblValue) / (65536));
dblDutyCycle = 100 * dblHighCycles / (dblHigh dblLowCycles));
dblHighTime = 0.000001 * dblHighCycles;
dblLowTime = 0.000001 * dblLowCycles;
//Read the count from Counter1. This is an unsigned 32-bit value.
eGet (lngHandle, LJ_ioGET_COUNTER, 1, &dblValue, 0);
Following is pseudocode to reset the input timer and the counter:
//Reset the duty-cycle measurement (Timer1) to zero, by writing
//a value of zero. The duty-cycle measurement is continuously
//updated, so a reset is normally not needed, but one reason
//to reset to zero is to detect whether there has been a new
//measurement or not.
ePut (lngHandle, LJ_ioPUT_TIMER_VALUE, 1, 0, 0);
//Read & reset Counter1. Note that with the U3 reset is just
//setting a driver flag to reset on the next read, so reset
//is generally combined with a read in an add/go/get block.
//The order of the read & reset within the block does not
//matter ... the read will always happen right before the reset.
AddRequest (lngHandle, LJ_ioGET_COUNTER, 1, &dblValue, 0, 0);
AddRequest (lngHandle, LJ_ioPUT_COUNTER_RESET, 1, 1, 0, 0);
GoOne (lngHandle);
GetResult (lngHandle, LJ_ioGET_COUNTER, 1, &dblValue);
GetResult (lngHandle, LJ_ioPUT_COUNTER_RESET, 1, 0);
Note that if a timer/counter is read and reset at the same time (in the same Add/Go/Get block), the read will return the value just
before reset.
4.3.7 - Stream Mode
The highest input data rates are obtained in stream mode, which is supported with U3 hardware version 1.21 or higher. See
Section 3.2 for more information about stream mode.
There are five IOTypes used to control streaming:
LJ_ioCLEAR_STREAM_CHANNELS
LJ_ioADD_STREAM_CHANNEL
LJ_ioADD_STREAM_CHANNEL_DIFF //Put negative channel in x1.
LJ_ioSTART_STREAM //Value returns actual scan rate.
LJ_ioSTOP_STREAM
LJ_ioGET_STREAM_DATA
The following constant is passed in the Channel parameter with the get stream data IOType to specify a read returning all scanned
channels, rather than retrieving each scanned channel separately:
LJ_chALL_CHANNELS
The following are special channels, used with the get/put config IOTypes, to write or read various stream values:
LJ_chSTREAM_SCAN_FREQUENCY
LJ_chSTREAM_BUFFER_SIZE //UD driver stream buffer size in samples.
LJ_chSTREAM_WAIT_MODE
LJ_chSTREAM_DISABLE_AUTORECOVERY
LJ_chSTREAM_BACKLOG_COMM //Read-only. 0=0% and 256=100%.
LJ_chSTREAM_BACKLOG_UD //Read-only. Number of samples.
LJ_chSTREAM_SAMPLES_PER_PACKET //Default 25. Range 1-25.
LJ_chSTREAM_READS_PER_SECOND //Default 25.
36