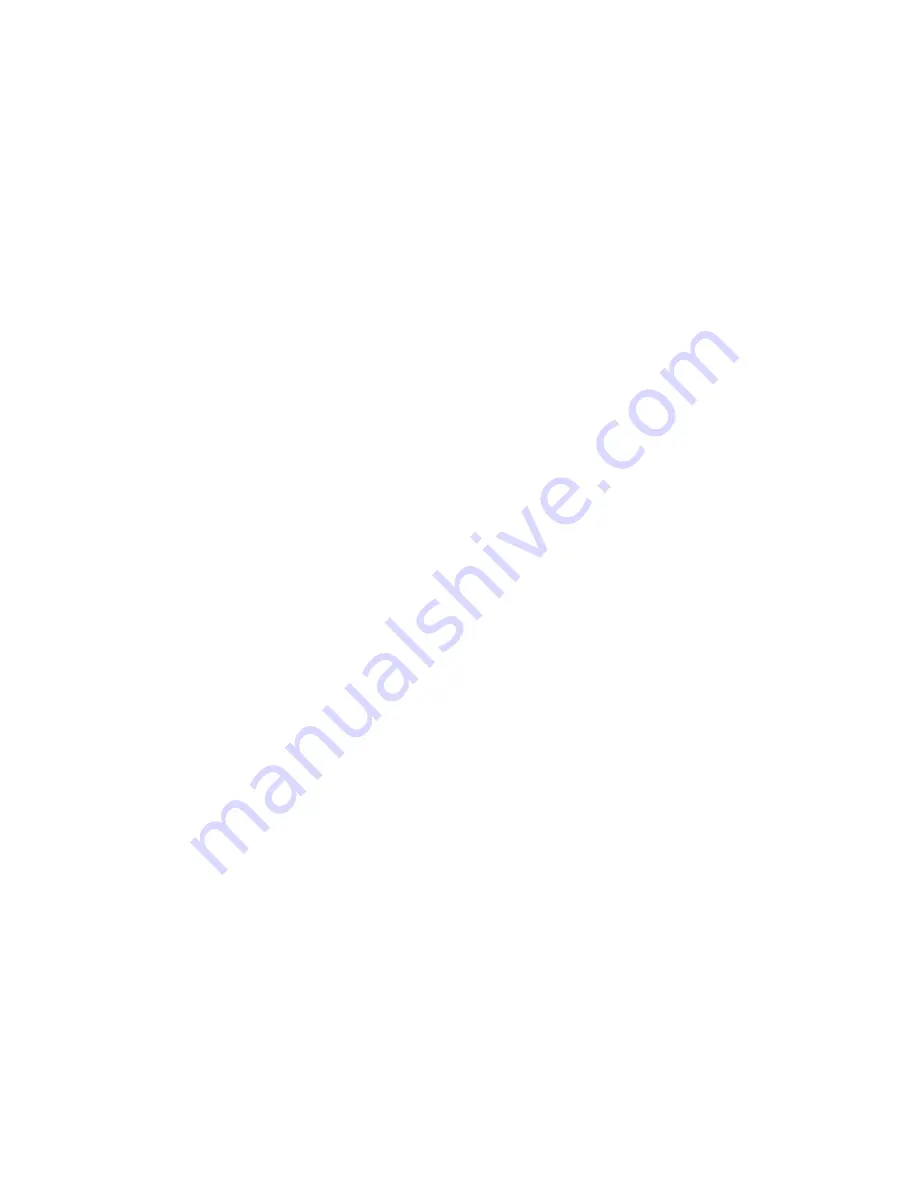
LJ_ioGET_DIGITAL_BIT_DIR
LJ_ioGET_DIGITAL_BIT_STATE
LJ_ioGET_DIGITAL_PORT //Also sets directions to input. x1 is number of bits.
LJ_ioGET_DIGITAL_PORT_DIR //x1 is number of bits.
LJ_ioGET_DIGITAL_PORT_STATE //x1 is number of bits.
LJ_ioPUT_DIGITAL_BIT //Also sets direction to output.
LJ_ioPUT_DIGITAL_PORT //Also sets directions to output. x1 is number of bits.
DIR is short for direction. 0=input and 1=output.
The general bit and port IOTypes automatically control direction, but the _DIR and _STATE ones do not. These can be used to
read the current condition of digital I/O without changing the current condition. Note that the _STATE reads are actually doing a
read using the input circuitry, not reading the state value last written. When you use LJ_ioGET_DIGITAL_BIT_STATE or
LJ_ioGET_DIGITAL_PORT_STATE on a line set to output, it leaves it set as output, but it is doing an actual state read based on
the voltage(s) on the pin(s). So if you set a line to output-high, but then something external is driving it low, it might read low.
When a request is done with one of the port IOTypes, the Channel parameter is used to specify the starting bit number, and the x1
parameter is used to specify the number of applicable bits. The bit numbers corresponding to different I/O are:
0-7 FIO0-FIO7
8-15 EIO0-EIO7
16-19 CIO0-CIO3
Note that the GetResult function does not have an x1 parameter. That means that if two (or more) port requests are added with the
same IOType and Channel, but different x1, the result retrieved by GetResult would be undefined. The
GetFirstResult/GetNextResult commands do have the x1 parameter, and thus can handle retrieving responses from multiple port
requests with the same IOType and Channel.
Following is example pseudocode for various digital I/O operations:
//Execute the pin_configuration_reset IOType so that all
//pin assignments are in the factory default condition.
//The ePut function is used, which combines the add/go/get.
ePut (lngHandle, LJ_ioPIN_CONFIGURATION_RESET, 0, 0, 0);
//Now, an add/go/get block to execute multiple requests.
//Request a read from FIO2.
AddRequest (lngHandle, LJ_ioGET_DIGITAL_BIT, 2, 0, 0, 0);
//Request a read from FIO4-EIO5 (10-bits starting
//from digital channel #4).
AddRequest (lngHandle, LJ_ioGET_DIGITAL_PORT, 4, 0, 10, 0);
//Set FIO3 to output-high.
AddRequest (lngHandle, LJ_ioPUT_DIGITAL_BIT, 3, 1, 0, 0);
//Set EIO6-CIO2 (5-bits starting from digital channel #14)
//to b10100 (=d20). That is EIO6=0, EIO7=0, CIO0=1,
//CIO1=0, and CIO2=1.
AddRequest (lngHandle, LJ_ioPUT_DIGITAL_PORT, 14, 20, 5, 0);
//Execute the requests.
GoOne (lngHandle);
//Get the FIO2 read.
GetResult (lngHandle, LJ_ioGET_DIGITAL_BIT, 2, &dblValue);
//Get the FIO4-EIO5 read.
GetResult (lngHandle, LJ_ioGET_DIGITAL_PORT, 4, &dblValue);
4.3.6 - Timers & Counters
There are eight IOTypes used to write or read timer and counter information:
LJ_ioGET_COUNTER
LJ_ioPUT_COUNTER_ENABLE
LJ_ioGET_COUNTER_ENABLE
LJ_ioPUT_COUNTER_RESET //Sets flag to reset on next read.
LJ_ioGET_TIMER
LJ_ioPUT_TIMER_VALUE
LJ_ioPUT_TIMER_MODE
LJ_ioGET_TIMER_MODE
In addition to specifying the channel number, the following mode constants are passed in the value parameter when doing a
request with the timer mode IOType:
LJ_tmPWM16 //16-bit PWM output
LJ_tmPWM8 //8-bit PWM output
LJ_tmRISINGEDGES32 //Period input (32-bit, rising edges)
LJ_tmFALLINGEDGES32 //Period input (32-bit, falling edges)
LJ_tmDUTYCYCLE //Duty cycle input
LJ_tmFIRMCOUNTER //Firmware counter input
LJ_tmFIRMCOUNTERDEBOUNCE //Firmware counter input (with debounce)
LJ_tmFREQOUT //Frequency output
LJ_tmQUAD //Quadrature input
LJ_tmTIMERSTOP //Timer stop input (odd timers only)
LJ_tmSYSTIMERLOW //System timer low read
LJ_tmSYSTIMERHIGH //System timer high read
LJ_tmRISINGEDGES16 //Period input (16-bit, rising edges)
LJ_tmFALLINGEDGES16 //Period input (16-bit, falling edges)
The following are special channels, used with the get/put config IOTypes, to configure a parameter that applies to all
timers/counters:
LJ_chNUMBER_TIMERS_ENABLED //0-2
LJ_chTIMER_CLOCK_BASE //Value constants below
LJ_chTIMER_CLOCK_DIVISOR //0-255, where 0=256
LJ_chTIMER_COUNTER_PIN_OFFSET //4-8 only starting with hardware rev 1.30.
With the clock base special channel above, the following constants are passed in the value parameter to select the frequency:
LJ_tc4MHZ //4 MHz clock base
LJ_tc12MHZ //12 MHz clock base
LJ_tc48MHZ //48 MHz clock base
LJ_tc1MHZ_DIV //1 MHz clock base w/ divisor (no Counter0)
LJ_tc4MHZ_DIV //4 MHz clock base w/ divisor (no Counter0)
LJ_tc12MHZ_DIV //12 MHz clock base w/ divisor (no Counter0)
LJ_tc48MHZ_DIV //48 MHz clock base w/ divisor (no Counter0)
LJ_tcSYS //Equivalent to LJ_tc48MHZ
Following is example pseudocode for configuring various timers and a hardware counter:
//Execute the pin_configuration_reset IOType so that all
35