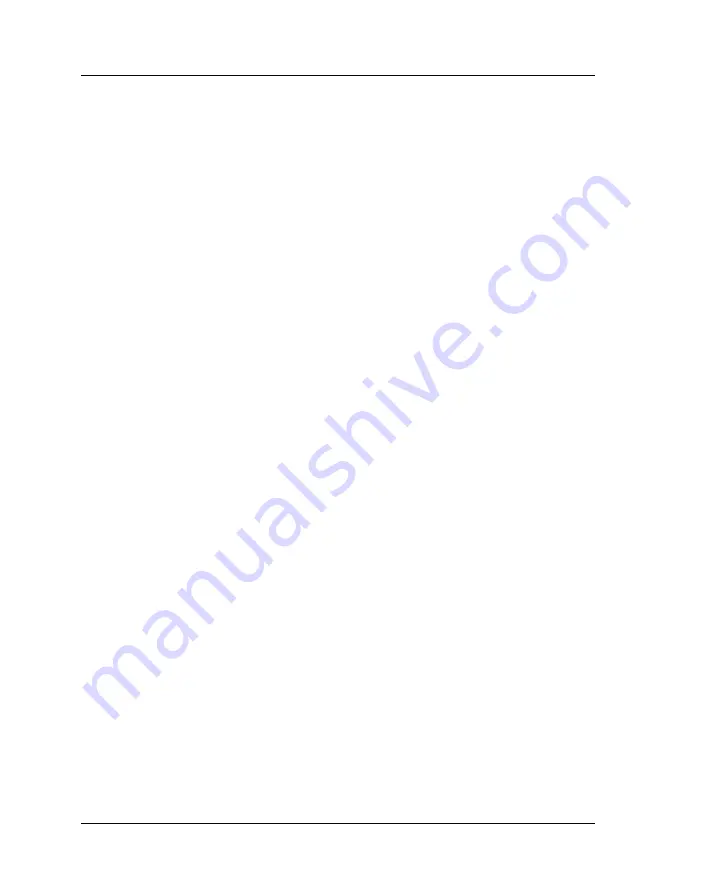
Chapter 4 — Sample Applications
47
FMTestApp
// If the cue was successful, set the encoder state to cued and
// activate the start button.
pFMInterface->SetEncoderState( esCued );
SetButtons();
}
Note that we always check the
result
return value to ensure that the method suc-
ceeded. COM-related errors raise exceptions, while the components themselves
return a long result which is typically set to 0, if successful, or a negative value
on error. For a complete list of Filter Manager error codes, refer to Appendix C.
All of the Filter Manager calls listed in the above code have been discussed in
previous sections.
Releasing the COM Libraries
Like all resources, the COM libraries must be released when the programmer is
finished using them. For this purpose, a single method is provided by COM called
CoUninitialize
. The
CoUninitialize
method should be invoked as follows in the
destructor of the client code:
…
CoUninitialize();
…
At this point, you may be wondering about releasing the interface pointers. The
_com_ptr_t
Smart Pointer class handles this for you. The
IArgusFMPtr
destructor
is invoked when the Smart Pointer goes out of scope. In its destructor, it releases the
interface pointer it encapsulates after calling the
Release
method on the interface.
Registering to Receive Filter Manager Events
When you’re programming in C++, registering to receive events is not the easiest
of tasks. However, ATL does provide a template class, IDispEventImpl, to assist
C++ client applications in receiving events from the server.
Before registering to receive events, make certain that you have already called
_Module.Init( NULL, AfxGetInstanceHandle())
to initialize the data members of
the COM server module. This should be done when you initialize your applica-
tion. Also remember to call
_Module.Term()
before exiting your application.
These are the steps you’ll need to follow to register for events using the
IDispEventImpl template: