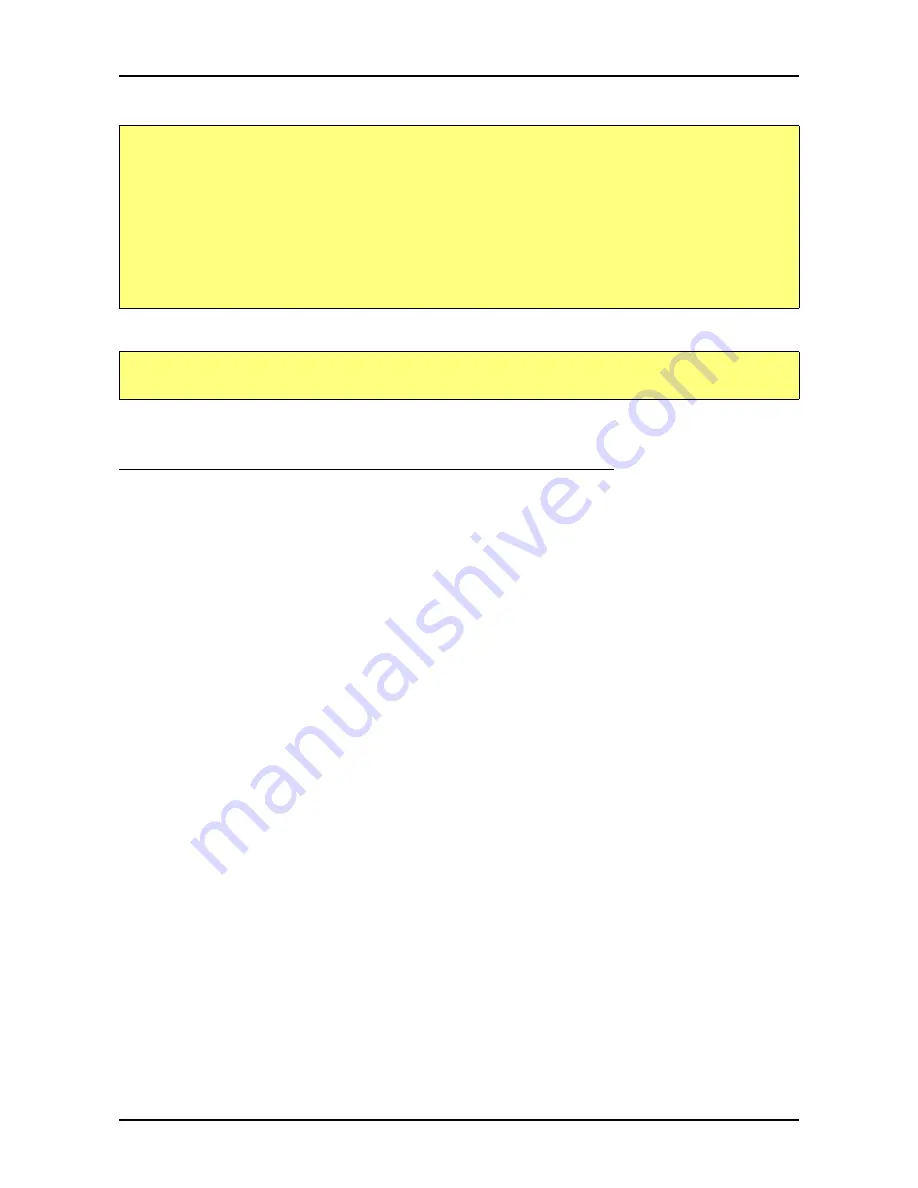
Software
Driver functions
(c) Spectrum GmbH
47
Function spcm_dwGetParam
Example:
The example reads out the serial number of the installed card and prints it. As the serial number is available under all circumstances there is
no error checking when calling this function.
Different call types of spcm_dwSetParam and spcm_dwGetParam: _i32, _i64, _i64m
The three functions only differ in the type of the parameters that are used to call them. As some of the registers can exceed the 32 bit integer
range (like memory size or post trigger) it is recommended to use the _i64 function to access these registers. However as there are some
programs or compilers that don’t support 64 bit integer variables there are two functions that are limited to 32 bit integer variables. In case
that you do not access registers that exceed 32 bit integer please use the _i32 function. In case that you access a register which exceeds 64
bit value please use the _i64m calling convention. Inhere the 64 bit value is splitted in a low double word part and a high double word part.
Please be sure to fill both parts with valid information.
If accessing 64 bit registers with 32 bit functions the behaviour differs depending on the real value that is currently located in the register.
Please have a look at this table to see the different reactions depending on the size of the register:
uint32 _stdcall spcm_dwGetParam_i32 ( // Return value is an error code
drv_handle hDevice, // handle to an already opened device
int32 lRegister, // software register to be read out
int32* plValue); // pointer for the return value
uint32 _stdcall spcm_dwGetParam_i64m ( // Return value is an error code
drv_handle hDevice, // handle to an already opened device
int32 lRegister, // software register to be read out
int32* plValueHigh, // pointer for the upper part of the return value
uint32* pdwValueLow); // pointer for the lower part of the return value
uint32 _stdcall spcm_dwGetParam_i64 ( // Return value is an error code
drv_handle hDevice, // handle to an already opened device
int32 lRegister, // software register to be read out
int64* pllValue); // pointer for the return value
int32 lSerialNumber;
spcm_dwGetParam_i32 (hDrv, SPC_PCISERIALNO, &lSerialNumber);
printf (“Your card has serial number: %05d\n”, lSerialNumber);
Internal register read/write
Function type
Behaviour
32 bit register
read
spcm_dwGetParam_i32
value is returned as 32 bit integer in plValue
32 bit register
read
spcm_dwGetParam_i64
value is returned as 64 bit integer in pllValue
32 bit register
read
spcm_dwGetParam_i64m
value is returned as 64 bit integer, the lower part in plValueLow, the upper part in plValueHigh. The upper part can
be ignored as it’s only a sign extension
32 bit register
write
spcm_dwSetParam_i32
32 bit value can be directly written
32 bit register
write
spcm_dwSetParam_i64
64 bit value can be directly written, please be sure not to exceed the valid register value range
32 bit register
write
spcm_dwSetParam_i64m
32 bit value is written as llValueLow, the value llValueHigh needs to contain the sign extension of this value. In case
of llValueLow being a value >= 0 llValueHigh can be 0, in case of llValueLow being a value < 0, llValueHigh has to
be -1.
64 bit register
read
spcm_dwGetParam_i32
If the internal register has a value that is inside the 32 bit integer range (-2G up to (2G - 1)) the value is returned
normally. If the internal register exceeds this size an error code ERR_EXCEEDSINT32 is returned. As an example:
reading back the installed memory will work as long as this memory is < 2 GByte. If the installed memory is >= 2
GByte the function will return an error.
64 bit register
read
spcm_dwGetParam_i64
value is returned as 64 bit integer value in pllValue independent of the value of the internal register.
64 bit register
read
spcm_dwGetParam_i64m
the internal value is splitted into a low and a high part. As long as the internal value is within the 32 bit range, the
low part plValueLow contains the 32 bit value and the upper part plValueHigh can be ignored. If the internal value
exceeds the 32 bit range it is absolutely necessary to take both value parts into account.
64 bit register
write
spcm_dwSetParam_i32
the value to be written is limited to 32 bit range. If a value higher than the 32 bit range should be written, one of
the other function types need to used.
64 bit register
write
spcm_dwSetParam_i64
the value has to be splitted into two parts. Be sure to fill the upper part lValueHigh with the correct sign extension
even if you only write a 32 bit value as the driver every time interprets both parts of the function call.
64 bit register
write
spcm_dwSetParam_i64m
the value can be written directly independent of the size.