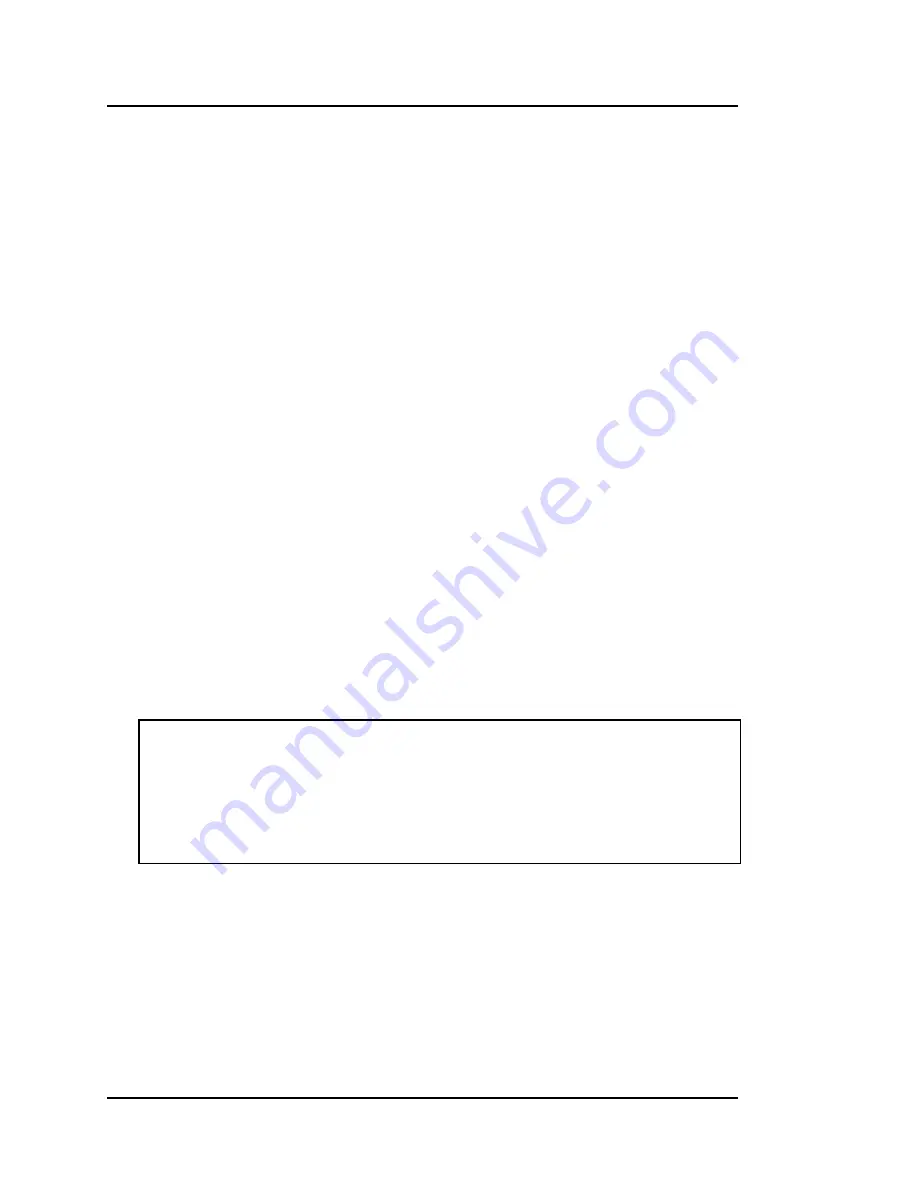
Danaher Motion
06/2005
Project
M-SS-005-03 Rev
E
55
Functions are different from subroutines in one respect. Functions always
return a value to the task that called the function. Otherwise, functions and
subroutines use the same syntax and follow the same rules of application
and behavior.
Because funtions return a value, you can use functions expressions (i.e.,
tangent=sin(x)/cos(x) or If (Sgn(x) Or Sgn(y)) Then…). The syntax for
defining a function is:
Function <
name
> ({{ByVal} <
p_1
> as <
type_1
> }…{, {ByVal} <
p_n
> as
type_n
>}) As
<
function type
>
{
local variable declaration
}
{
function code
}
END function
You can pass parameters (either scalar or array) to the function. These
parameters are then used in the code of the function. Declare the variable
names and types of parameters you want to pass in the declaration line for
the function. Parameters can be passed by reference or by value (ByVal).
The default is by reference. When you pass a variable by reference, you
actually pass the address of the variable to the function, which changes the
value of the variable (if the function code is written to do this). When you
pass a variable by value, a copy of the value of the variable is passed to the
function. The function cannot change the value of the variable. Arrays are
only passed by reference.
To set up the return value, assign the value you want to return to a virtual
variable with the same name as the function somewhere in the code of the
function. You do not declare the variable, but the function uses it to obtain
the return value. If the function's code includes conditional statements,
assignments to the function variable can be made in multiple locations in the
function code.
There is no explicit limit on the number of functions allowed in a task. All
functions must be located following the main program and must be contained
wholly outside of the main program.
A function can be recursive (can call itself). The following example defines a
recursive function to calculate the value of
N
:
FUNCTION Factorial (ByVal N As Long) As Double
'Declaring
N
as long truncates floating point numbers to integers
'The function returns a Double value
If N < 3 then 'This statement stops the recursion
Factorial = N '0!=0; 1!=1' 2!=2
Else
Factorial=N * Factorial(N-1) 'Recursive statement
End If
END FUNCTION
When writing a recursive function, you must have an IF statement to force
the function to return without the recursive call being executed. Otherwise,
the function never returns once it is called.
3. 4
M
ULTI
-
TASKING
The MC supports multi-tasking. You can have multiple tasks running
independently, sharing a single computer. A task is a section of code that
runs in its own context. Microsoft Windows
®
is a multi-tasking system. If you
open Explorer and Word at the same time, they run nearly independently of
each another.