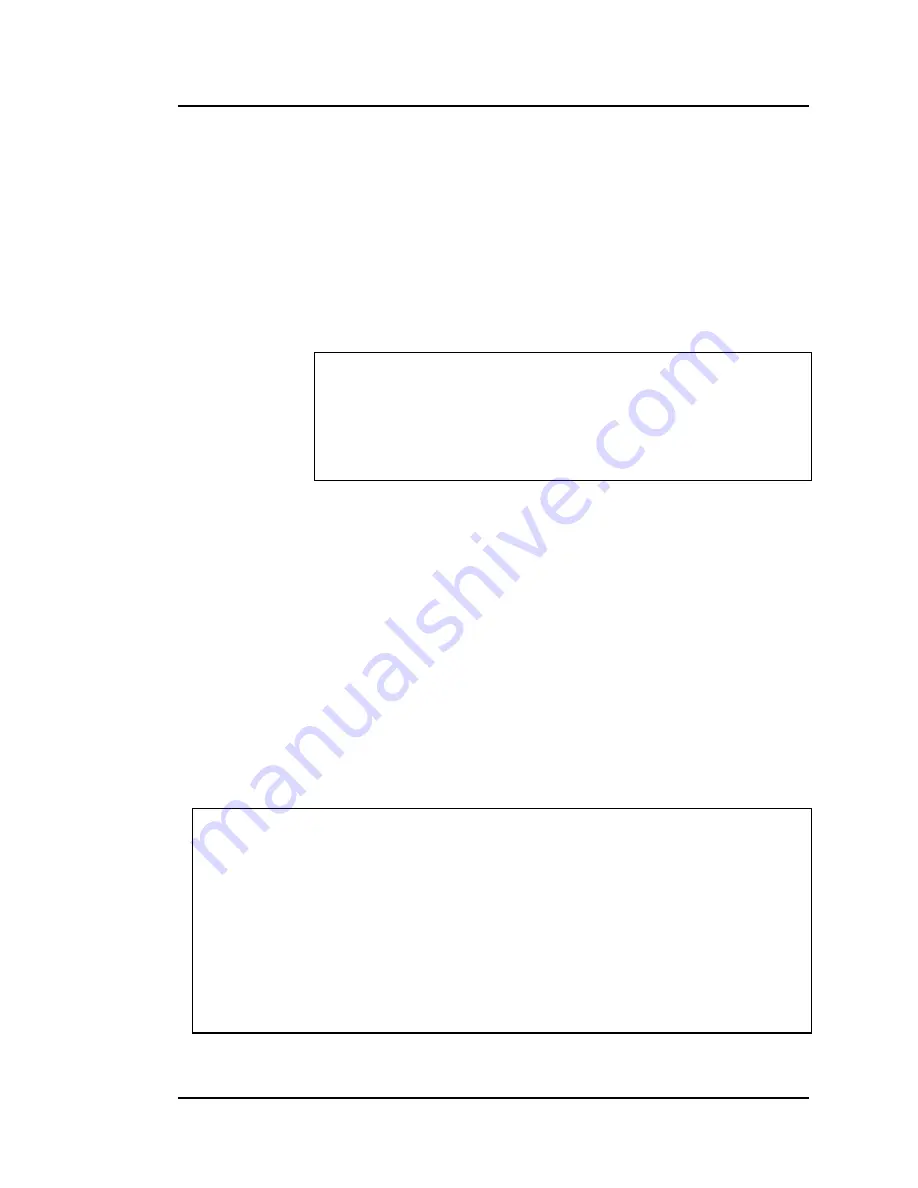
BASIC Moves Development Studio
06/2005
Danaher Motion
36 Rev
E
M-SS-005-03l
2. 3
P
ROGRAM
D
ECLARATIONS
You must declare the start of programs and subroutines. For programs, use
Program…End Program
. Use
Sub…End Sub
keywords for subroutines.
2.3.1. Program
The
Program…End Program
keywords mark the boundary between the
variable declaration section and the main program. Each task must have only
one
Program
keyword, which ends with the
End Program
keyword. Another
option is the
Program Continue…Terminate Program
block. In this case, the
program is automatically executed after loading, and automatically unloaded
from memory when it ends.
{Import of libraries}
{Declaration of global and static variables}
PROGRAM
…
END PROGRAM
{Local functions and subroutines}
2.3.2. Subroutine
Parameters (either scalar or array) passed to the subroutine are used within
the code of the subroutine. The declaration line for the subroutine
(
SUB<name>
) is used to declare names and types of the parameters to pass.
Parameters are passed either by reference or by value (ByVal). The default
method is to pass parameters by reference. Whole arrays are passed only
by reference. Trying to pass a whole array by value results in a translation
error. However, array elements can be passed both by reference and by
value. The syntax for a subroutine is:
SUB <
name
> ({<
par_1
>([*])+ as <
type_1
>}…{, <
par_
n>([*])+ as<
type_
n>})
…
END SUB
<
par_l
>: name of array variable
<
par_
n>: name of array variable
[*]: dimension of an array without specifying the bounds
+: means one or more [*]
SUB
CalculateMean(x[*][*] as DOUBLE, TheMean[*] as LONG)
DIM sum as DOUBLE
DIM I as LONG
FOR i = 1 to 100
sum = sum + x[i][1]
NEXT i
TheMean[1] = sum/100
END SUB
SUB
PrintMean(ByVal Mean as LONG)
PRINT “Mean Value Is “, Mean
END SUB
CALL
CalculateMean(XArray, TheMeanArray) ‘ Pass entire array by reference
CALL
PrintMean(TheMeanArray[1]) ‘ Pass a single array element by value