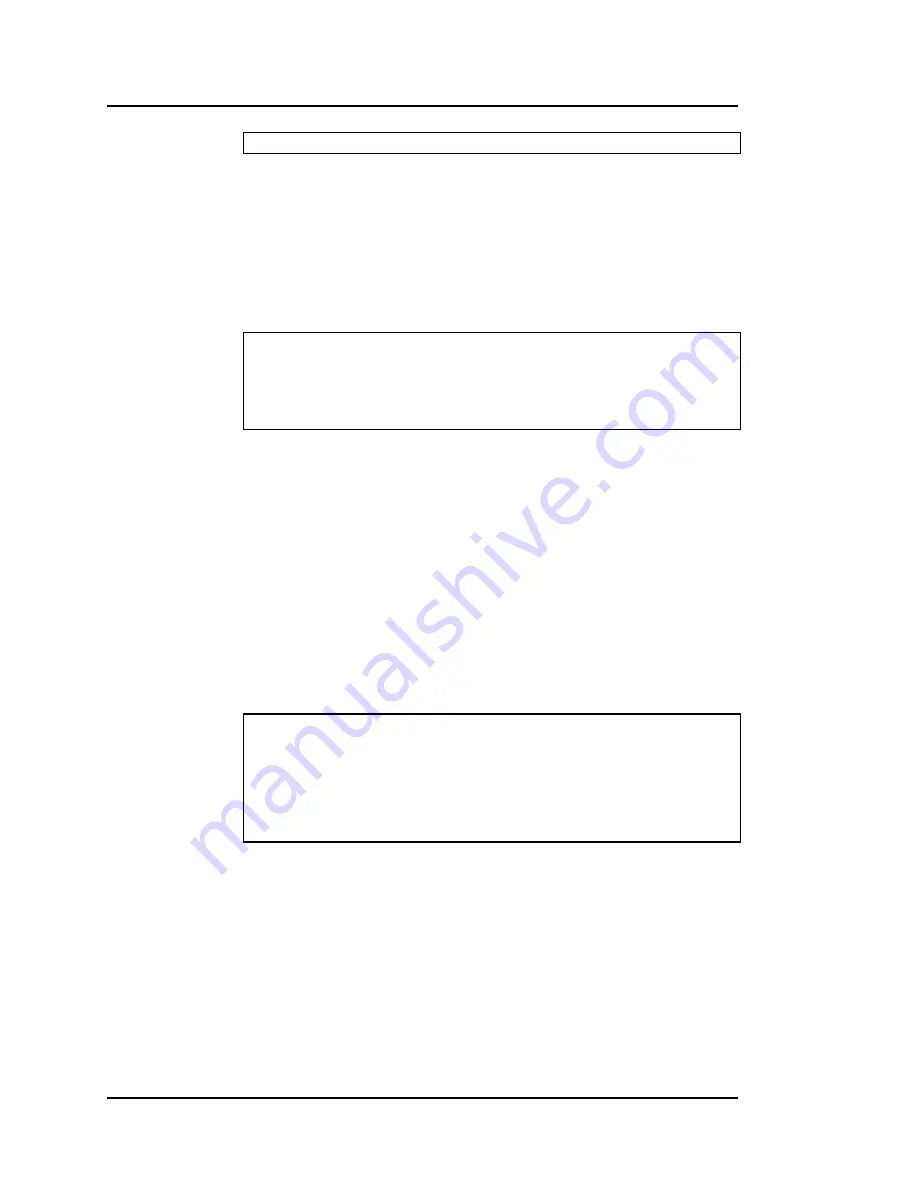
Danaher Motion
06/2005
Project
M-SS-005-03 Rev
E
53
Use
CALL
to execute a subroutine:
CALL <subroutine>({<p_1>...<p_n>})
where:
<
subroutine>
is the name of the subroutine
<p_1>
...
<p_n>
are the subroutine parameters
Parentheses are not used in a subroutine if no parameters are passed.
MC-BASIC automatically checks the type of compliance between the
subroutine declaration and the subroutine call. Any type mismatch causes an
error during program loading. Automatic casting applies to numericc
variables types. For example, suppose MySub is a subroutine that takes a
Long parameter. In this case, the following scenario applies:
CALL MySub(3.3432)
'OK: The double value 3.3432 is demoted to the Long value, 3
CALL MySub(a)
'Error: a is a string, there is a type mismatch
Call MySub(3,4)
'Error: The number of parameters is not correct
See the Subroutine Example in Appendix A for further details.
3.3.1. Arrays
Arrays can only be passed by reference. If a user tries to pass a whole array
by value, the translator gives an error. Array syntax is:
SUB <
name
> ({<
p_1
>([*])+ as <
type_1
>}…{, <
p_n
>([*])+ as <
type_n
>})
{
local variable declaration
}
{
subroutine code
}
END SUB
where
<
p_l
> : name of array variable
<
p_n
> : name of array variable
[*] : dimension of array without specifying the bounds
+ : means one or more
Syntax example:
SUB mean(x[*][*] as DOUBLE, TheMean[*] as LONG)
DIM sum as DOUBLE
DIM I as LONG
FOR i = 1 to 100
sum = sum + x[i][1]
NEXT i
TheMean[1] = sum/100
END SUB
Subroutine Libraries
. As you develop programs for a variety of applications,
you will find that there are some subroutines that you use repeatedly. You
can collect such subroutines (and user-defined functions) into a library file.
Then, when you program a task, import the
.
lib
file at the beginning of the
program and you call any of its defined subroutines (functions).
An MC-BASIC library is an ASCII file containing only the sub-program's
code. The file does not have a main program part. The names of the library
files must have the extension,
.LIB
.