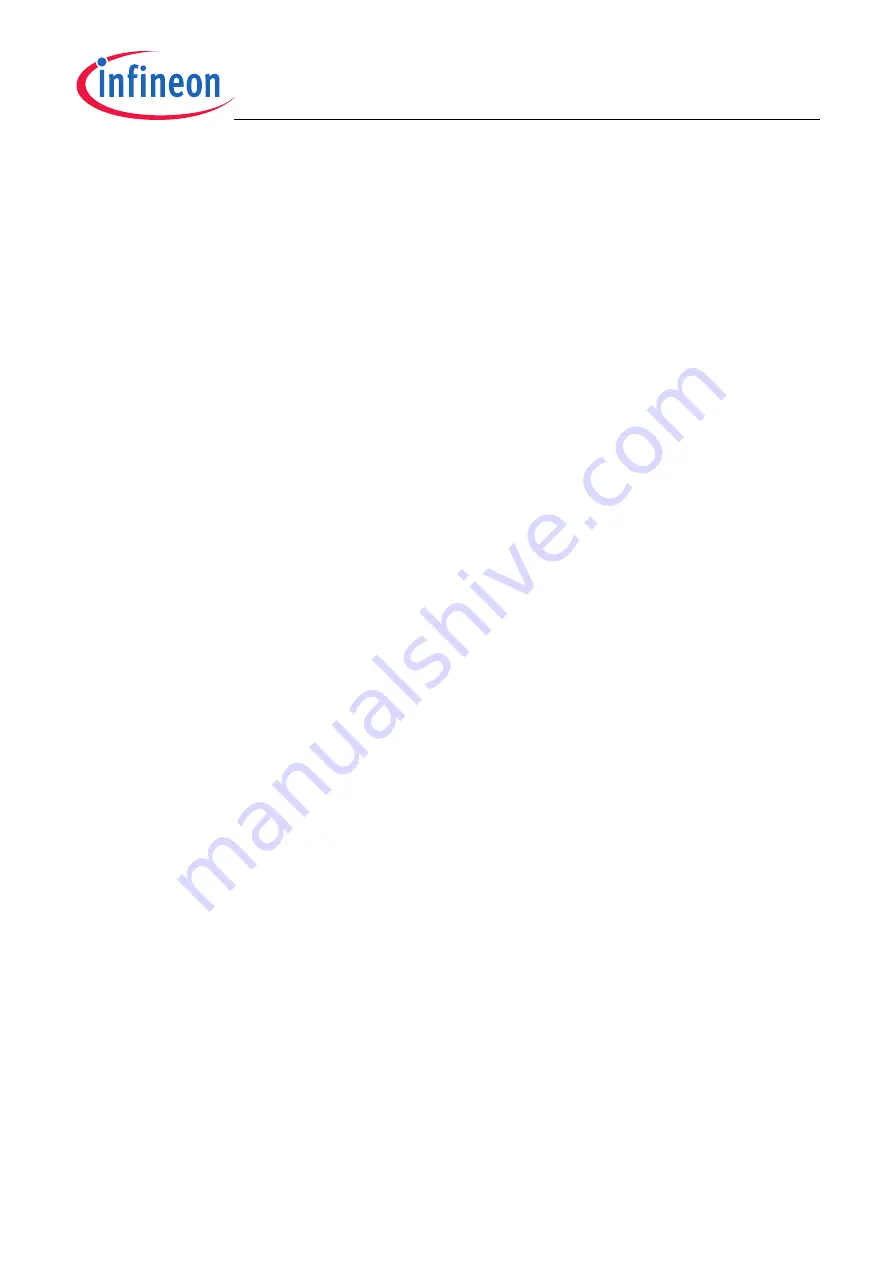
TLE5012B
Interfaces
User’s Manual
39
Rev. 1.2, 2018-02
CRC generation software code example
Two software codes with C-language to generate CRC are provided. The first example is a more intuitive though
slower solution, since two iterative loops are done; a loop for each byte and an inner loop for each bit. It is also a
compact solution.
The second code is faster, since the inner loop is implemented as a look-up table (LUT). Therefore, the CRC does
not need to be calculated each time, but is taken from the look-up table, saving some computational time. As a
look-up table is required, some extra memory space is needed compared to the first example.
Example 1:
//“message” is the data transfer for which a CRC has to be calculated.
//A typical “message” consists of 2 bytes for the command word plus 2 bytes for the
//data word plus 2 bytes for the safety word.
//“Bytelength” is the number of bytes in the “message”. A typical “message” has 6
//bytes.
unsigned char CRC8(unsigned char *message, unsigned char Bytelength)
{
//“crc” defined as the 8-bits that will be generated through the message till the
//final crc is generated. In the example above this are the blue lines out of the
//XOR operation.
unsigned char crc;
//“Byteidx” is a counter to compare the bytes used for the CRC calculation
unsigned char Byteidx, Bitidx;
//Initially the CRC remainder has to be set with the original seed (0xFF for the
//TLE5012B).
crc = 0xFF;
//For all the bytes of the message.
for(Byteidx=0; Byteidx<Bytelength; +)
{
//“crc” is calculated as the XOR operation from the previous “crc” and the “message”.
//“^” is the XOR operator.
crc ^= message[Byteidx];
//For each bit position in a 8-bit word
for(Bitidx=0; Bitidx<8; +)
{
//If the MSB of the “crc” is 1(with the &0x80 mask we get the MSB of the crc).
if((crc&0x80)!=0)
{
//“crc” advances on position (“crc” is moved left 1 bit: the MSB is deleted since it
//will be cancelled out with the first one of the generator polynomial and a new bit
//from the “message” is taken as LSB.)
crc <<=1;
//“crc” is calculated as the XOR operation from the previous “crc” and the generator
//polynomial (0x1D for TLE5012B). Be aware that here the x8 bit is not taken since
//the MSB of the “crc” already has been deleted in the previous step.
crc ^= 0x1D;
}