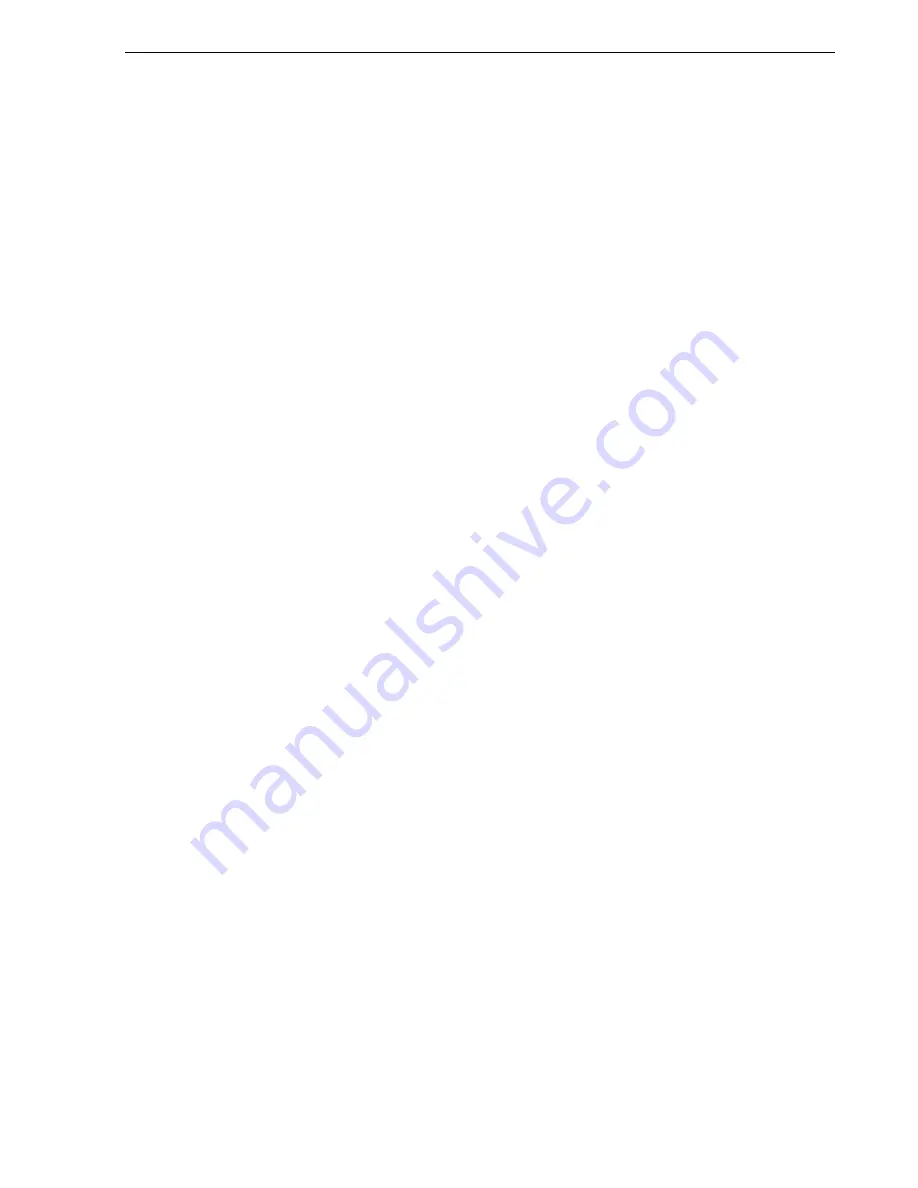
CHAPTER 5 APPLICATION EXAMPLES
Application Note U17121EJ1V1AN
89
/////////////////////////////////////////////////////////////////////////
// Function name: ATA_Write_DMA //
// Function: Executes WRITE DMA command (Protocol:DM, Command:CAh). //
// Argument: dev_num : Device selection (0:Master/1:Slave) //
// lba : LBA //
// sec_cnt : Number of sectors //
// Return value: //
// STATUS_SUCCESS : Normal end //
// STATUS_TIMEOUT_DEVICE_SELECTION : DEVICE SELECTION error end //
// STATUS_TIMEOUT_BSY0_DRQ0 : BSY=0,DRQ=0 timeout error end //
// STATUS_TIMEOUT_DRDY1 : DRDY=1 timeout error end //
// STATUS_TIMEOUT_INTRQ : INTRQ timeout error end //
// STATUS_TIMEOUT_BMEND : BM timeout error end //
// STATUS_IDE_ERROR : Error end after command execution //
// //
/////////////////////////////////////////////////////////////////////////
int ATA_Write_DMA(int dev_num, UWORD lba, UHWORD sec_cnt)
{
int status;
ATA_COMMAND ac;
ac.features = 0x00; // Features register
ac.sector_count = sector_count; // SectorCount register
ac.sector_number = (lba & 0xFF); // SectorNumber register
ac.cylinder_low = (lba>>8 & 0xFF); // CylinderLow register
ac.cylinder_high = (lba>>16 & 0xFF); // CylinderHigh register
ac.device_head = 0x40|(dev_num<<4)|(lba>>24 & 0x0F); // Device/Head register
ac.command = 0xCA; // Command register
status = ATA_DMA(&ac);
return status;
}
/////////////////////////////////
// Protocol execution function //
/////////////////////////////////
/////////////////////////////////////////////////////////////////////////
// Function name: ATA_Device_Selection //
// Function: Executes device selection protocol. //
// Argument: dev_num : (0:Master / 1:Slave) //
// Return value: //
// STATUS_SUCCESS : Normal end //
// STATUS_TIMEOUT_BSY0_DRQ0 : BSY=0,DRQ=0 timeout error end //
// //
/////////////////////////////////////////////////////////////////////////
int ATA_Device_Selection(int dev_num)
{