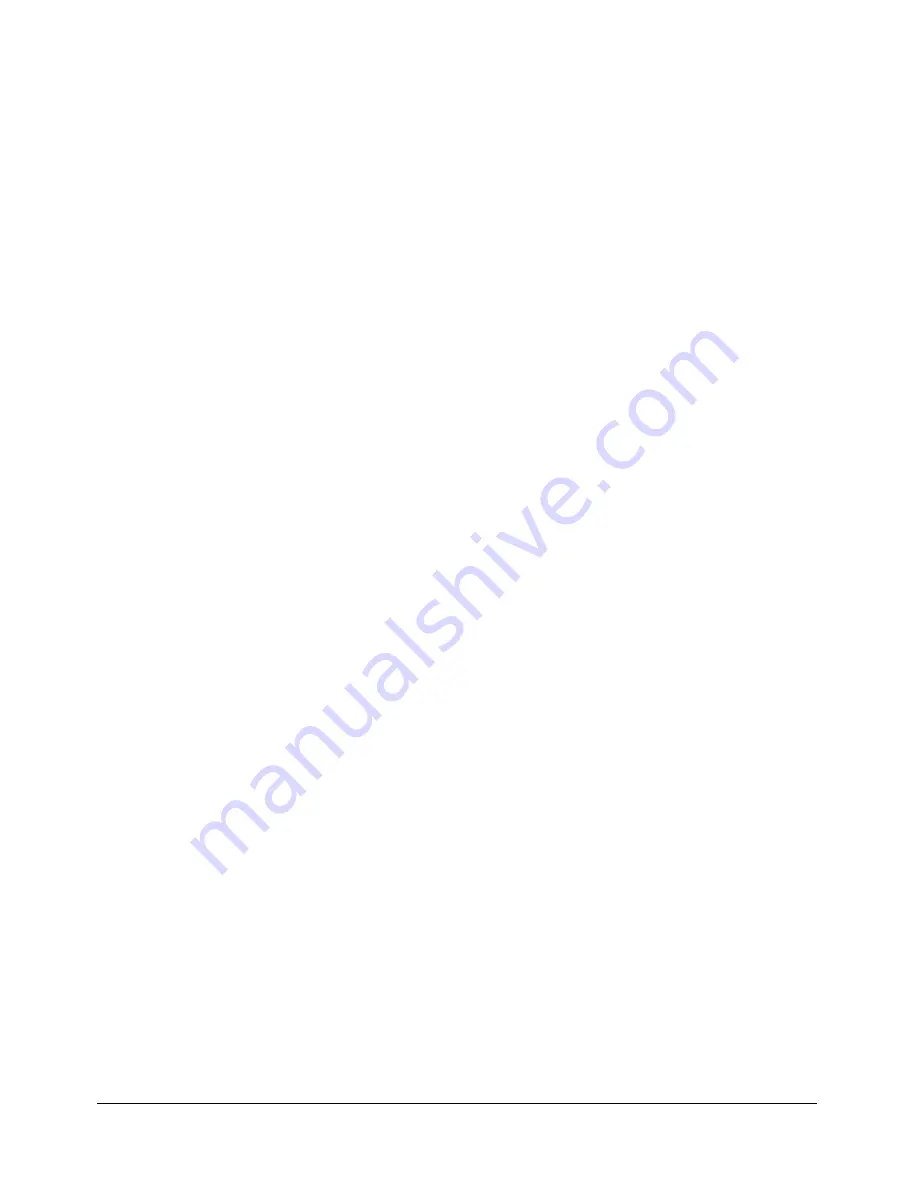
Using classes: a simple example
49
4.
In your text editor, enter the following code (in this procedure, new code you type in each step
is
bold
):
class Person {
}
This is called the class
declaration
. In its most basic form, a class declaration consists of the
class
keyword, followed by the class name (Person, in this case), and then left and right curly
braces (
{}
). Everything between the braces is called the class
body
and is where the class’s
properties and methods are defined.
The name of the class (Person) must exactly match the name of the AS file that contains it
(Person.as). This is very important; if these two names don’t match exactly, including
capitalization, the class won’t compile.
5.
To create the properties for the Person class, use the
var
keyword to define two variables named
age
and
name
, as shown in the following example:
class Person {
var age:Number;
var name:String;
}
Tip:
By convention, class properties are defined at the top of the class body, which makes the
code easier to understand, but this isn’t required.
The colon syntax (
var age:Number
and
var name:String
) used in the variable declarations
is an example of strict data typing. When you type a variable in this way (
var
variableName
:
variableType
), the ActionScript 2.0 compiler ensures that any values
assigned to that variable match the specified type. If the correct data type is not used in the
FLA file importing this class, an error is thrown by the compiler. Using strict typing is good
practice and can make debugging your scripts easier. (For more information, see
“Strict data
typing” on page 24
.)
6.
Next, you’ll add a special function called a
constructor function
. In object-oriented
programming, the constructor function initializes each new instance of a class.
The constructor function always has the same name as the class. To create the class’s
constructor function, add the following code:
class Person {
var age:Number;
var name:String;
// Constructor function
function Person (myName:String, myAge:Number) {
this.name = myName;
this.age = myAge;
}
}
Summary of Contents for FLEX-FLEX ACTIONSCRIPT LANGUAGE
Page 1: ...Flex ActionScript Language Reference...
Page 8: ......
Page 66: ...66 Chapter 2 Creating Custom Classes with ActionScript 2 0...
Page 76: ......
Page 133: ...break 133 See also for for in do while while switch case continue throw try catch finally...
Page 135: ...case 135 See also break default strict equality switch...
Page 146: ...146 Chapter 5 ActionScript Core Language Elements See also break continue while...
Page 808: ...808 Chapter 7 ActionScript for Flash...
Page 810: ...810 Appendix A Deprecated Flash 4 operators...
Page 815: ...Other keys 815 Num Lock 144 186 187 _ 189 191 192 219 220 221 222 Key Key code...
Page 816: ...816 Appendix B Keyboard Keys and Key Code Values...
Page 822: ...822 Index...